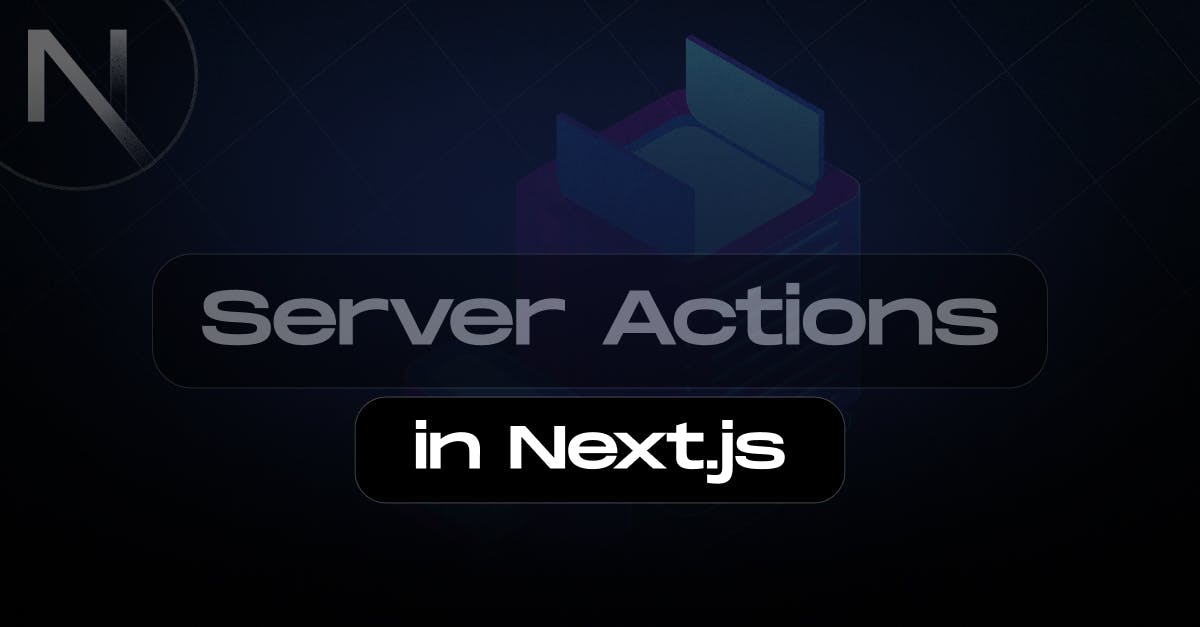
Exploring Server Actions in Next.js: A Game-Changing Feature for Web Developers
- Author: Md. Saad
- Published at: October 21, 2024
- Updated at: May 04, 2025
Next.js has long been a favourite in the React ecosystem, and with every update, it keeps evolving to meet modern development needs. One of its standout additions is Server Actions, a feature designed to shift key logic from the client to the server. Server Actions improve security and performance by allowing developers to handle operations like data updates, form submissions, and database interactions server-side. In this post, we’ll explain what Server Actions are, why they matter, and how you can use them effectively in your Next.js projects.
What are Server Actions?
Server Actions in Next.js are asynchronous functions that run strictly on the server, offering a cleaner, safer way to handle operations like form submissions, data updates, or any logic that shouldn't live in the browser. One of their key advantages is flexibility—they can be triggered from both Server and Client Components. But regardless of where they're invoked, the execution happens securely on the server.
This setup improves performance by reducing unnecessary client-side work and protects sensitive logic from being exposed in the browser. It's a smarter, more modern approach to handling backend interactions in frontend code without relying on traditional API endpoints.
Implementing Server Action
Server Actions are defined using the React "use server" directive. You can add this directive at the top of an asynchronous function to designate that specific function as a Server Action. Alternatively, you can place the "use server" directive at the beginning of a file, which will automatically mark all exported functions in that file as Server Actions. This allows for flexible handling of server-side logic directly from your component.
First, create an async function using the server directive to use the server actions inside the server component. You must place the directive on top of the function body. Then, you can call the server function. Here’s a basic example:
// app/page.jsx
export default function Page() {
// Server Action
async function create() {
'use server'
// Perform data mutation
}
return <button onClick={create}>Create</button>
}
You can also define Server Actions in a separate file and import them into your components:
// app/actions.js
'use server'
export async function create() {
// Perform data mutation
}
// app/ui/button.jsx
import { create } from '@/app/actions'
export function Button() {
return <button onClick={create}>Create</button>
}
Implementing Server Action in client components
A Next.js project may have client and server components. We may want to use server actions inside the client components, and Next Js allows us to do that as well.
To invoke a Server Action in a Client Component, create a new file and include the "use server" directive at the start. All exported functions in the file will be tagged as Server Actions, which can be utilised in both client and server components:
// app/actions.js
'use server'
export async function create() {
// Perform data mutation
}
// app/ui/button.jsx
'use client'
import { create } from '@/app/actions'
export function Button() {
return <button onClick={create}>Create</button>
}
Passing actions as props
In Next.js, you can also pass a Server Action to a Client Component as a prop like this:
<ClientComponent updateItemAction={updateItem} />
// app/client-component.js
'use client'
export default function ClientComponent({ updateItemAction }) {
return <form action={updateItemAction}>{/* ... */}</form>
}
Typically, the Next.js TypeScript plugin would issue a warning when passing a function (like updateItemAction) from the server to the client, as functions generally can't be serialized across client-server boundaries. However, Next.js uses a special heuristic: when a prop is named action or ends with Action, the plugin assumes it may be a Server Action.
This is just a naming convention and doesn't imply the TypeScript plugin can definitively know if it's dealing with a Server Action or a regular function. Still, at runtime, type-checking will ensure that you don't mistakenly pass a non-serializable function into a Client Component.
Using Server Actions in Forms
Server Actions can be invoked using the action attribute in a <form> element.
When a form is submitted, the Server Action automatically receives the FormData object. This means you don't need to use useState to manage form fields. Instead, you can extract the form data directly using the native FormData methods.
// app/page.jsx
export default function Page() {
async function handleSubmit(formData) {
'use server'
// Handle form submission
}
return (
<form action={handleSubmit}>
<input type="text" name="name" />
<button type="submit">Submit</button>
</form>
)
}
You can pass additional arguments to a Server Action using JavaScript's bind method. Here’s an example:
'use client'
import { updateUser } from './actions'
export function UserProfile({ userId }) {
// Bind the userId as an additional argument to the updateUser function
const updateUserWithId = updateUser.bind(null, userId)
return (
<form action={updateUserWithId}>
<input type="text" name="name" />
<button type="submit">Update User Name</button>
</form>
)
}
In this example, the updateUserWithId function binds the userId argument to the updateUser function. When the form is submitted, the updateUser function will receive the userId along with the form data.
Using Server Actions in Forms
Server Actions can be invoked using the action attribute in a <form> element.
When a form is submitted, the Server Action automatically receives the FormData object. This means you don't need to use useState to manage form fields. Instead, you can extract the form data directly using the native FormData methods.
// app/page.jsx
export default function Page() {
async function handleSubmit(formData) {
'use server'
// Handle form submission
}
return (
<form action={handleSubmit}>
<input type="text" name="name" />
<button type="submit">Submit</button>
</form>
)
}
You can pass additional arguments to a Server Action using JavaScript's bind method. Here’s an example:
'use client'
import { updateUser } from './actions'
export function UserProfile({ userId }) {
// Bind the userId as an additional argument to the updateUser function
const updateUserWithId = updateUser.bind(null, userId)
return (
<form action={updateUserWithId}>
<input type="text" name="name" />
<button type="submit">Update User Name</button>
</form>
)
}
In this example, the updateUserWithId function binds the userId argument to the updateUser function. When the form is submitted, the updateUser function will receive the userId along with the form data.
On the server side:
'use server'
export async function updateUser(userId, formData) {
// handle updating the user with the userId and formData
}
This allows you to pass the userId as an additional argument to the server action alongside the form data.
Using Server Action we can also add other awesome features in our next js form such as Programmatic form submission, server-side form validation, revalidating data, error handling and so on. You can read further from the Next.js official documentation to learn more.
Final Thoughts
Server Actions in Next.js offer a clean and efficient way to manage server-side logic, without the overhead of traditional API routes. Whether processing form data, updating databases, or securing sensitive operations, this feature helps improve performance, streamline development, and keep your frontend focused.
As modern web applications evolve, adopting tools like Server Actions can help you stay ahead with a more secure and maintainable architecture.
Need help implementing Server Actions or optimising your Next.js project? The team at StaticMania is here to support you, whether it’s for performance tuning, feature development, or full-stack integration. Let’s build smarter, faster web experiences together.
FAQs
Server Actions are asynchronous functions that run on the server in a Next.js application. They allow developers to perform operations like form submissions and database mutations securely, without exposing logic to the client side.
To create a Server Action, use the "use server" directive at the top of an async function. You can place it inside a component or at the beginning of a file to mark all exports as Server Actions.
Yes, Server Actions can be used in Client Components by passing them as props. You must define the actions in a file with the "use server" directive, and Next.js will allow them to be safely invoked from the client.
Server Actions improve security and performance by moving critical logic—like data updates or form handling—away from the client. This helps avoid exposing sensitive operations and reduces the need for external APIs or form state management.
You can use a Server Action as the action attribute in a <form> tag. When the form is submitted, the action function receives the FormData directly, so there's no need to manage form fields with useState.
In many cases, yes. Server Actions simplify development by keeping business logic closer to the component and reducing boilerplate. However, traditional API routes may still be useful for complex workflows or RESTful APIs.