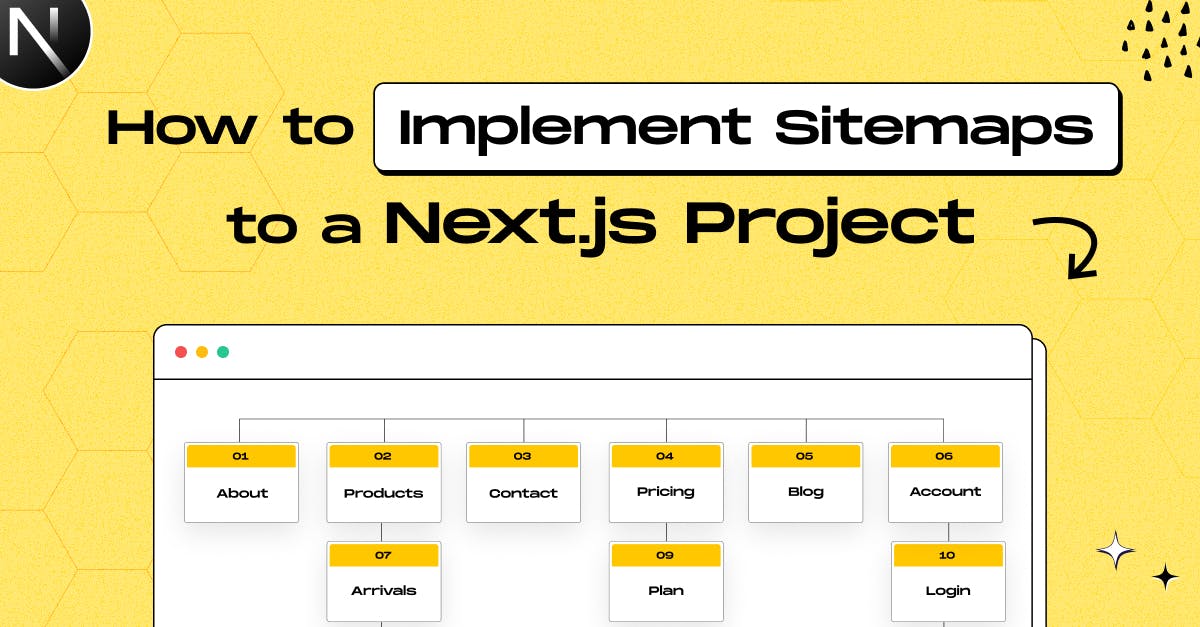
How to Implement Sitemaps in Next.js Project to Improve Search Engine Visibility
- Author: Md. Saad
- Published at: January 21, 2024
- Updated at: January 21, 2024
The simplest method of communicating with Google is through sitemaps. To help Google more effectively crawl your website and identify fresh material, they show the URLs that are part of it and when they change. A sitemap is a file where you provide information about your site's pages, videos, and other files and the relationships between them. Search engines like Google read this file to more intelligently crawl your site.
How to Implement Sitemaps to a Next.js Project
There are two options to add sitemaps in Next.js:
- Manually adding Sitemap
- Dynamically adding Sitemap
Manually adding Sitemap
If you have a smaller application, you may construct a manual sitemap by generating a sitemap.xml file in your project's public directory and identifying the URLs of the pages you wish to include. To do that create a sitemap.js file and place it in the root of your app directory and add the following code.
export default function sitemap() {
return [
{
url: 'https://example.com',
lastModified: new Date(),
changeFrequency: 'yearly',
priority: 1,
},
{
url: 'https://example.com/about',
lastModified: new Date(),
changeFrequency: 'monthly',
priority: 0.8,
},
{
url: 'https://example.com/blog',
lastModified: new Date(),
changeFrequency: 'weekly',
priority: 0.5,
},
]
}
Dynamically Adding Sitemap
In most cases, your site will be dynamic. In that case, you have to use getServerSideProps
To use getServerSideProps to generate a dynamic sitemap, first add a new page named sitemap.js to the pages directory of your Next.js project. This page will be in charge of creating the sitemap. Now, use the following code to add a sitemap.xml file:
//pages/sitemap.xml.js
const EXTERNAL_DATA_URL = 'https://example.com/posts';
function generateSiteMap(posts) {
return `<?xml version="1.0" encoding="UTF-8"?>
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">
<!--We manually set the two URLs we know already-->
<url>
<loc>https://example.com</loc>
</url>
<url>
<loc>https://example.com/guide</loc>
</url>
${posts
.map(({ id }) => {
return `
<url>
<loc>${`${EXTERNAL_DATA_URL}/${id}`}</loc>
</url>
`;
})
.join('')}
</urlset>
`;
}
function SiteMap() {
// getServerSideProps will do the heavy lifting
}
export async function getServerSideProps({ res }) {
// We make an API call to gather the URLs for our site
const request = await fetch(EXTERNAL_DATA_URL);
const posts = await request.json();
// We generate the XML sitemap with the posts data
const sitemap = generateSiteMap(posts);
res.setHeader('Content-Type', 'text/xml');
// we send the XML to the browser
res.write(sitemap);
res.end();
return {
props: {},
};
}
export default SiteMap;
- generateSiteMap function generates an XML string that includes static URLs (such as the homepage and an "about" page) as well as dynamic URLs for each of the blog posts in the posts array.
- EXTERNAL_DATA_URL is the URL from which data will be fetched.
- The getServerSideProps function will return an object with a props property that contains the data you want to pass to the component.
Last Talk
After completing these steps, you will get a dynamic sitemap for your Next.js application that refreshes automatically when new material is added. This will allow search engines to crawl and index your website more effectively. Thus, it will enhance its SEO. If you have any questions on how to incorporate sitemaps into the Next.js project, please contact us. StaticMania teams are available to serve at any time.