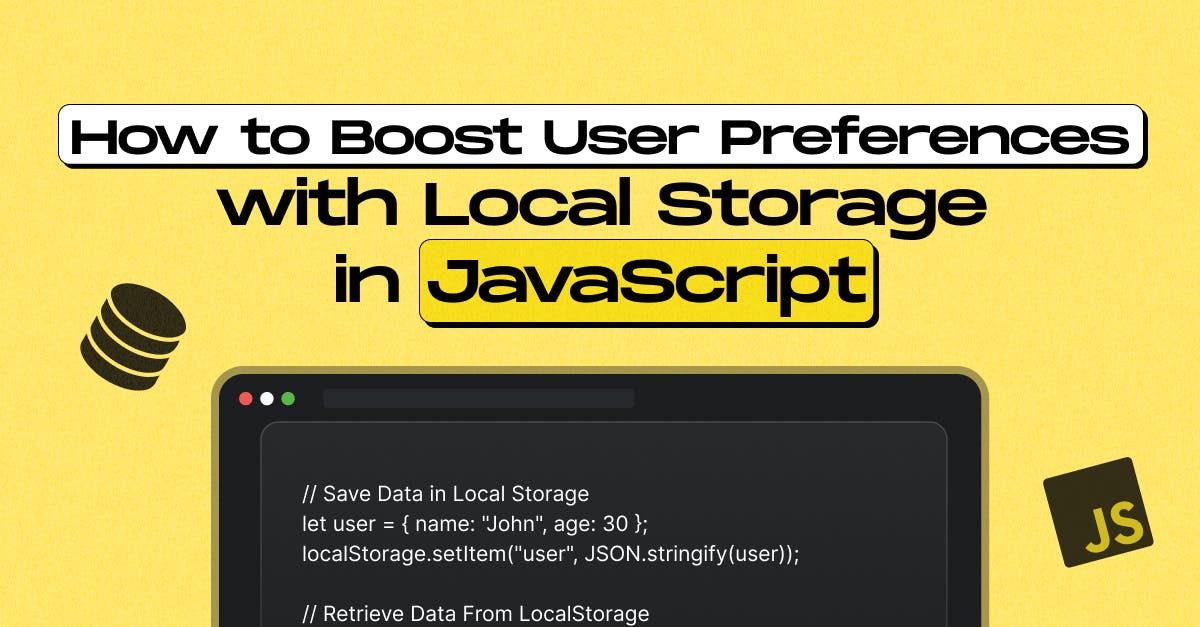
How to Boost User Preferences with Local Storage in JavaScript
- Author: Md. Saad
- Published at: February 23, 2025
- Updated at: February 25, 2025
Local Storage is a powerful feature in JavaScript. It allows developers to store key-value pairs in a user's browser permanently. Unlike session storage, which clears data when the session ends, local storage data is kept even after the browser is closed.
This blog will explore using local storage effectively, its advantages, limitations, and some best practices.
What is Local Storage?
Local Storage is a feature of the Web Storage API that allows websites to store data in a browser persistently. Unlike cookies, which are sent with every HTTP request, Local Storage data remains on the client side and is not transmitted to the server. This makes it a more efficient way to store non-sensitive data to improve the user experience.
How to Use Local Storage
Local Storage operates using simple methods:
1. Saving Data to Local Storage
To store data, use the setItem method:
localStorage.setItem("username", "JohnDoe");
This method stores a key-value pair, where "username" is the key and "JohnDoe" is the value. The data will persist in the browser until explicitly removed.
2. Retrieving Data from Local Storage
To retrieve stored data, use the getItem method:
let username = localStorage.getItem("username");
console.log(username); // Output: JohnDoe
3. Removing Data from Local Storage
To remove a specific item:
localStorage.removeItem("username");
To clear all stored data:
localStorage.clear();
4. Storing Objects in Local Storage
Local Storage only supports strings, so objects need to be converted using JSON.stringify().
let user = { name: "John", age: 30 };
localStorage.setItem("user", JSON.stringify(user));
To retrieve and parse the stored object:
let retrievedUser = JSON.parse(localStorage.getItem("user"));
console.log(retrievedUser.name); // Output: John
Using JSON.stringify() and JSON.parse() ensures that complex data types like objects and arrays are handled properly.
Practical Use Case: Saving User Preferences
A common use case for Local Storage is saving user preferences, such as theme settings. This allows users to keep their preferred settings even after refreshing or reopening the browser.
Example: Dark Mode Toggle
function applyTheme() {
const savedTheme = localStorage.getItem("theme") || "light";
document.body.classList.remove("light", "dark");
document.body.classList.add(savedTheme);
}
// Function to toggle theme and save preference
function toggleTheme() {
const currentTheme = document.body.classList.contains("light") ? "dark" : "light";
document.body.classList.remove("light", "dark");
document.body.classList.add(currentTheme);
localStorage.setItem("theme", currentTheme);
}
// Apply theme on page load
applyTheme();
document.getElementById("themeToggle").addEventListener("click", toggleTheme);
HTML Code:
<button id="themeToggle">Toggle Theme</button>
This ensures that:
- The theme persists across page reloads.
- The theme toggles correctly between light and dark modes.
Advantages of Local Storage
✅ Data remains even after the browser is closed.
✅ Simple API for storing and accessing data.
✅ works for the same source across several tabs in the browser.
Limitations of Local Storage
❌ Not secure for sensitive data (e.g., passwords, tokens).
❌ Only supports string values (requires JSON.stringify for objects).
❌ Synchronous operations can impact performance in large-scale applications.
Best Practices for Using Local Storage
🔹 Avoid storing sensitive information. Use session storage or secure cookies for authentication tokens.
🔹 Use JSON format: Always use JSON.stringify() and JSON.parse() objects.
🔹 Implement proper data validation: Ensure retrieved data is properly validated to avoid errors.
🔹 Monitor storage limits: Avoid exceeding storage capacity to prevent data loss.
🔹 Clear unused data: Regularly remove unnecessary items to keep storage clean.
When to Use Local Storage?
✔ Saving user preferences (e.g., theme settings, UI customization).
✔ Caching non-sensitive API responses.
✔ Storing temporary form data.
✔ Keeping track of user progress in web apps.
Conclusion
Local Storage is a useful feature for storing non-sensitive data persistently in web applications. While it's easy to use, developers must be aware of its limitations and security risks. By following best practices, you can effectively utilize local storage to enhance the user experience.
Have you used Local Storage in your projects? Share your thoughts in the comments below! 🚀
Need help with Javascript problems in your project? Get in Touch With Us Today!
FAQ Section – How to Boost User Preferences with Local Storage in JavaScript
Local Storage is a web storage feature that allows developers to persistently store key-value pairs in a user's browser. Unlike cookies, Local Storage data is not sent with every HTTP request and remains in the browser even after it is closed, making it a more efficient way to store non-sensitive data.
To store data, use localStorage.setItem("key", "value"). To retrieve data, use localStorage.getItem("key"). If storing objects, convert them to a string using JSON.stringify(), and parse them back using JSON.parse().
Local Storage allows data to persist even after the browser is closed, offers a simple API for storing and accessing data, and works across multiple tabs of the same origin without needing server requests.
Local Storage is not secure for storing sensitive data like passwords or tokens, only supports string values (requiring JSON.stringify() for objects), and operates synchronously, which may impact performance if used for large-scale data storage.
Avoid storing sensitive information, always use JSON.stringify() and JSON.parse() for objects, validate retrieved data to prevent errors, monitor storage limits to prevent exceeding capacity, and regularly clear unnecessary data for better performance.
Local Storage is best used for saving user preferences (such as theme settings), caching non-sensitive API responses, temporarily storing form data, and keeping track of user progress in web applications.