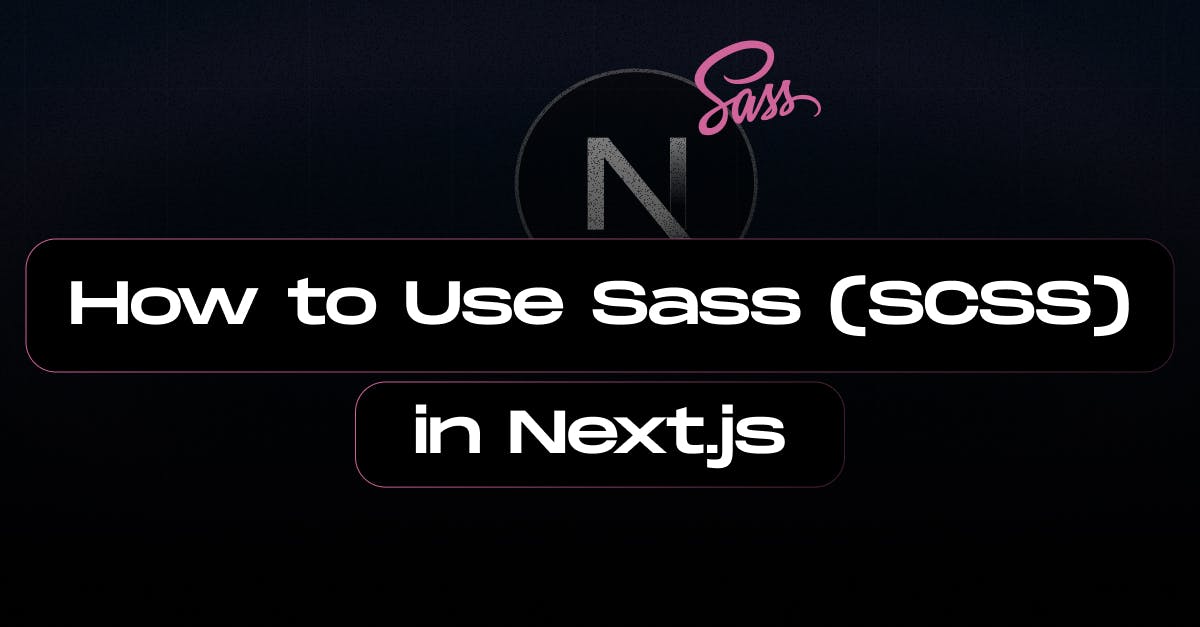
How to Use Sass (SCSS) in Next.js
- Author: Md. Saad
- Published at: November 06, 2024
- Updated at: November 30, 2024
Sass (Syntactically Awesome Style Sheets) is a preprocessor scripting language that extends CSS (Cascading Style Sheets) and adds more powerful, advanced features. It allows developers to write CSS more efficiently and more organised. Sass compiles into standard CSS, meaning web browsers can understand it after compilation.
To use Sass (SCSS) in a Next.js app that uses the App Router (/app directory), follow these steps:
Install sass
Next.js has built-in support for Sass, but you need to install the Sass package. Run the following command in your project directory:
npm install sass
Create SCSS Files
Once Sass is installed, you can create SCSS files as needed. For example, create a file named styles.scss in your /app directory or inside a specific component's folder:
// /app/styles.scss
$primary-color: #3498db;
body {
background-color: $primary-color;
}
Create SCSS Files
In Next.js with the App Router, you can import SCSS files directly into your components or layouts. For example, if you want to use the styles globally, import the SCSS file in your /app/layout.js or /app/layout.jsx:
// /app/layout.js or /app/layout.tsx
import './styles.scss'; // Import the SCSS file globally
export default function RootLayout({ children }) {
return (
<html lang="en">
<body>{children}</body>
</html>
);
}
If you want to import SCSS files in specific components, just import them directly in that component:
// /app/page.jsx
import './pageStyles.scss';
export default function HomePage() {
return (
<div className="homepage">
<h1>Welcome to the Next.js App!</h1>
</div>
);
}
Using CSS Modules (Optional)
If you want to scope styles to specific components using CSS Modules with SCSS, rename the SCSS files to follow the [name].module.scss naming convention. Then, import and use them in your component:
// /app/page.module.scss
.homepage {
color: #3498db;
font-size: 24px;
}
Now import this into the /app/page.jsx file:
// /app/page.jsx
import styles from './page.module.scss';
export default function HomePage() {
return (
<div className={styles.homepage}>
<h1>Welcome to the Next.js App!</h1>
</div>
);
}
With CSS modules, classes in the SCSS file are scoped automatically to the component.
Restart the Development Server
If the server is already running, make sure to restart it to pick up the changes after installing the Sass package:
npm run dev
Conclusion
In conclusion, integrating Sass (SCSS) into a Next.js app that uses the App Router is straightforward. By installing the Sass package and importing SCSS files directly into your components or layouts, you can enhance your app's styling with the power of Sass. Whether using global styles or CSS modules for scoped styles, Next.js provides built-in support for a smooth workflow. Just follow the steps to install, create, and import your SCSS files, and enjoy the flexibility and efficiency that Sass offers in managing your styles.
Keep exploring and enhancing your Next.js projects! Please do not hesitate to contact StaticMania with any questions or feedback. Happy coding!