Table of Contents
Get a Free Assistance
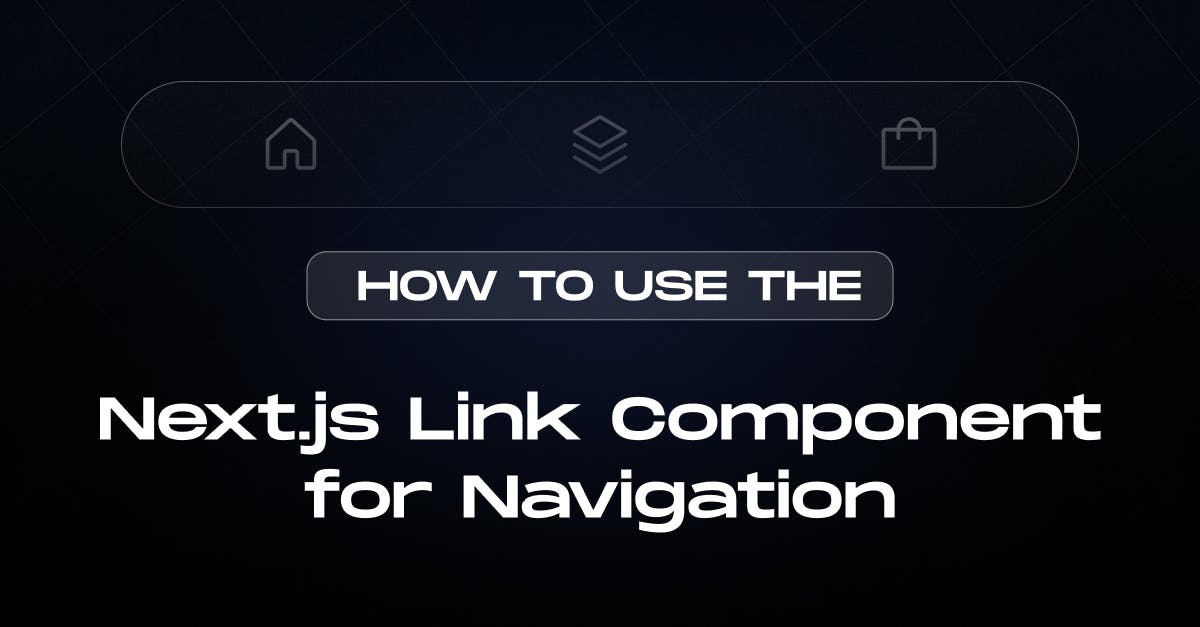
How to Use the Next.js Link Component for Navigation
- Author: Md. Saad
- Published at: August 25, 2024
- Updated at: August 25, 2024
The Link component in Next.js is essential to building navigable web pages and enabling client-side transitions between routes. Unlike traditional anchor (<a>) tags, the Link component ensures that navigation is optimized, preventing full page reloads, thus preserving the state and speeding up the navigation process. Let’s move on to the details on how to use the Next.JS Link Component for Navigation.
Basic Usage of the Link Component
To use the Link component, we first need to import it from the next/link package:
import Link from 'next/link';
export default function HomePage() {
return (
<div>
<Link href="/about">Go to About Page</Link>
</div>
);
}
In this example, href="/about" specifies the path we want to navigate to.
Available Props
We can customize the behavior of the Link component using various props:
👉 href: The path or URL to navigate to. (required field).
👉 prefetch: Automatically prefetches the page in the background (enabled by default).
👉 scroll: Controls whether the page scrolls to the top after navigation (enabled by default).
👉 replace: Replaces the current history state instead of pushing a new one.
Note: <a> tag attributes such as className or target="_blank" can be added to <Link> as props and will be passed to the underlying <a> element.
Props Details
✔ href:
This is a required field. This is used to add the path or URL where we want to navigate to.
<Link href="/dashboard">Dashboard</Link>
We can also pass query parameters in the href prop:
<Link
href={{
pathname: '/about',
query: { name: 'test' },
}}
>
About
</Link>
✔ Replace:
To replace the current URL instead of pushing a new entry into the history stack, we can use the replace prop. It takes boolean values. The default value is false. When true, next/link will replace the current history state instead of adding a new URL into the browser’s history stack.
import Link from 'next/link'
export default function Page() {
return (
<Link href="/dashboard" replace>
Dashboard
</Link>
)
}
Remember, The default behaviour of the Link component is to push a new URL into the history stack. We can use the replace prop to prevent adding a new entry.
✔ Scroll:
By default, <Link> scrolls to the top of a newly created route or keeps the scroll position for both forward and backward navigation. It also takes boolean values. The default value is true. When false, next/link will not scroll to the top of the page after a navigation.
import Link from 'next/link'
export default function Page() {
return (
<Link href="/dashboard" scroll={false}>
Dashboard
</Link>
)
}
Note: If there is a hash defined <Link> will scroll to the specific id, like a normal <a> tag. To prevent scrolling to the top /hash scroll={false} can be added to Link:
<Link href="/#hashid" scroll={false}>
Disables scrolling to the top
</Link>
✔ Prefetch:
The prefetch property in Next.js' Link component is used to control whether the linked page should be preloaded in the background. By default, Next.js will automatically prefetch the resources needed for the linked page when the Link component is rendered in the viewport. This prefetching occurs in the background, ensuring that when a user clicks the link, the navigation is faster and smoother because the resources have already been loaded. It takes boolean values as well as null values. The default value is null.
👉 null: Depending on the dynamic or static route, it will affect how prefetch behaves. For static routes, the entire route (including all data) will be prefetched. For dynamic routes, the partial route down to the next segment with a loading.Js border will be prefetched.
👉 true: if added true, the full route will be prefetched for both static and dynamic routes.
👉 false: If added false, prefetching will never happen either when entering the viewport or when hovering.
import Link from 'next/link'
export default function Page() {
return (
<Link href="/about" prefetch={false}>
About
</Link>
)
}
Linking to Dynamic Routes
For dynamic routes, generating the path of the link via template literals can be helpful.
For example, we can generate a list of links to the dynamic route app/blog/[slug]/page.js:
import Link from 'next/link'
function Page({ posts }) {
return (
<ul>
{posts.map((post) => (
<li key={post.id}>
<Link href={`/blog/${post.slug}`}>{post.title}</Link>
</li>
))}
</ul>
)
}
What if the child is a custom component that wraps a <a> tag or the child is a functional component?
If the child of Link is a custom component that wraps a <a> tag, we must add passHref to Link. This is necessary if we’re using libraries like styled-components. Without this, the <a> tag will not have the href attribute, which hurts our site's accessibility and might affect SEO. If we’re using ESLint, there is a built-in rule next/link-passhref to ensure correct usage of passHref.
import Link from 'next/link'
import styled from 'styled-components'
// This creates a custom component that wraps an <a> tag
const RedLink = styled.a`
color: red;
`
function NavLink({ href, name }) {
return (
<Link href={href} passHref legacyBehavior>
<RedLink>{name}</RedLink>
</Link>
)
}
export default NavLink
If the child of Link is a functional component, in addition to using passHref and legacyBehavior, we must wrap the component in React.forwardRef:
import Link from 'next/link'
// `onClick`, `href`, and `ref` need to be passed to the DOM element
// for proper handling
const MyButton = React.forwardRef(({ onClick, href }, ref) => {
return (
<a href={href} onClick={onClick} ref={ref}>
Click Me
</a>
)
})
function Home() {
return (
<Link href="/about" passHref legacyBehavior>
<MyButton />
</Link>
)
}
export default Home
Middleware and Link
Middleware is frequently used for authentication and other tasks that require redirecting the user to an alternate website. We must specify both the URL to display and the URL to prefetch to Next.js for the <Link /> component to prefetch links with rewrites via Middleware. This is necessary to prevent unnecessary fetches to middleware to determine the proper prefetch path.
For instance, we might include something like this in our middleware to reroute users to the appropriate page if we wish to serve a /dashboard route with visitor views and authentication:
// middleware.js
export function middleware(req) {
const nextUrl = req.nextUrl
if (nextUrl.pathname === '/dashboard') {
if (req.cookies.authToken) {
return NextResponse.rewrite(new URL('/auth/dashboard', req.url))
} else {
return NextResponse.rewrite(new URL('/public/dashboard', req.url))
}
}
}
In this case, we will use the following code in our <Link /> component:
import Link from 'next/link'
import useIsAuthed from './hooks/useIsAuthed'
export default function Page() {
const isAuthed = useIsAuthed()
const path = isAuthed ? '/auth/dashboard' : '/public/dashboard'
return (
<Link as="/dashboard" href={path}>
Dashboard
</Link>
)
}
Conclusion
The Link component in Next.js is a powerful tool for client-side routing, ensuring efficient and fast navigation within our application. By using it effectively, we can create seamless and dynamic user experiences.
We hope right now you are well aware of how to use the Next.js Link Component for navigation. Then, If you still feel the need for help, feel free to contact us. The dedicated teams at StaticMania are ready to assist you 24/7.