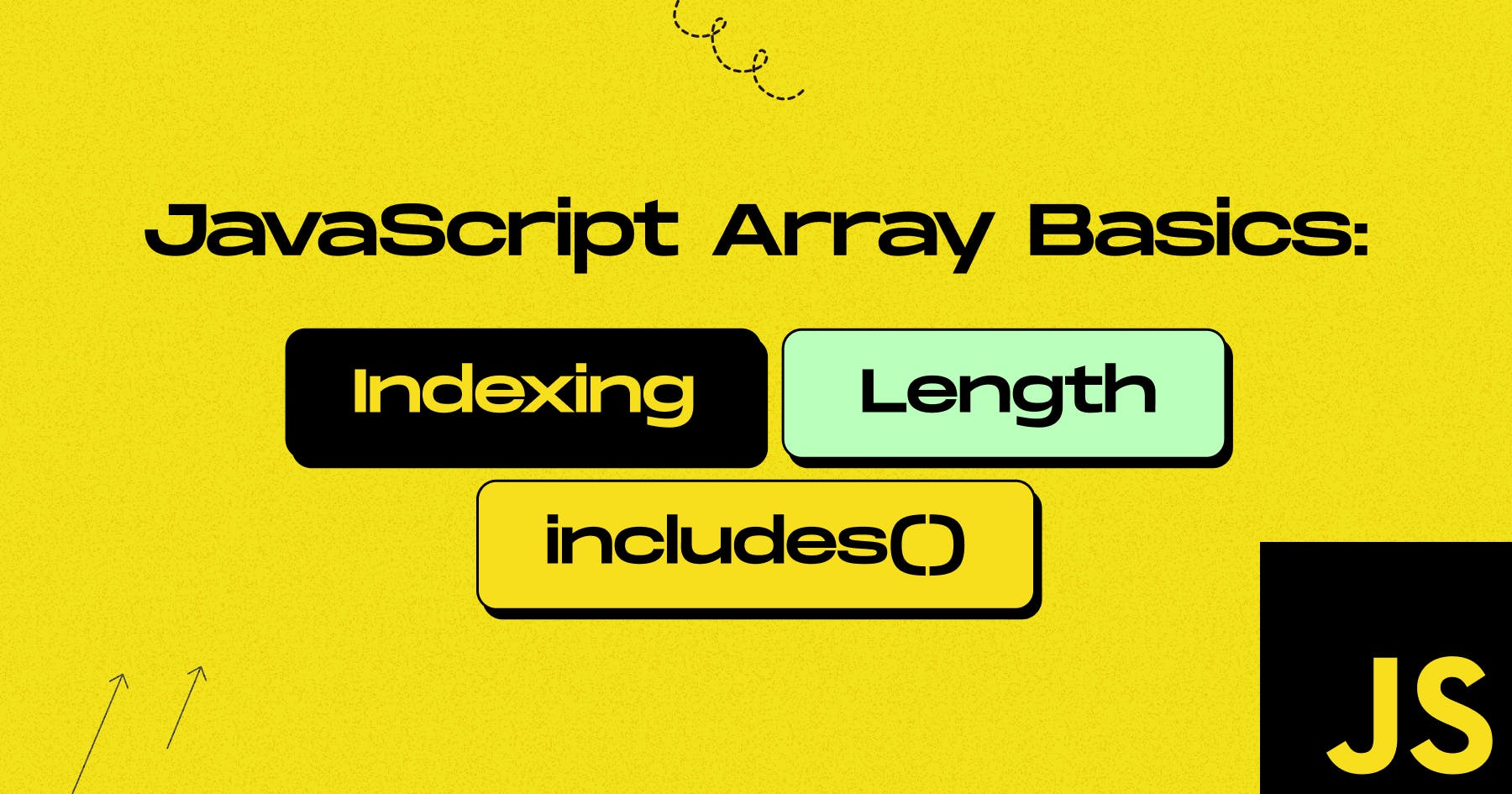
JavaScript Array Basics: Indexing, Length, and includes()
- Author: Md. Saad
- Published at: December 15, 2024
- Updated at: December 15, 2024
In JavaScript, an array is a special object designed to store ordered collections of values. Arrays are ideal for handling sequential and ordered lists of data. A developer must know arrays for managing and manipulating data collections. In this blog, we will discuss three fundamental concepts: indexing, length, and the includes() method
Indexing in Arrays
The position of an item within an array is called an index. In JavaScript, array indexing begins at 0, thus the first item has an index of 0, the second has an index of 1, and so on. Most computer languages use the same zero-based indexing method.
Accessing Array Elements
You can access elements of an array using square brackets ([]) and the index of the element
const fruits = ["apple", "banana", "cherry"];
console.log(fruits[0]); // Output: apple
console.log(fruits[2]); // Output: cherry
The length Property
The length property of an array returns the total number of entries. It is very handy for iterating over arrays or adding new elements. To get the length of an array, you have to use arrayName.length
Example:
const numbers = [10, 20, 30, 40];
console.log(numbers.length); // Output: 4
Using includes()
The includes() function determines whether a particular element is present in an array. If the element is found, it returns true; if not, it returns false.
Basic Syntax:
array.includes(element, startIndex);
- element: The item you’re searching for.
- startIndex (optional): The position to begin the search (default is 0).
Examples:
const colors = ["red", "green", "blue"];
console.log(colors.includes("green")); // Output: true
console.log(colors.includes("yellow")); // Output: false
Starting from a Specific Index
You can specify a starting index for the search.
const numbers = [1, 2, 3, 4, 2];
console.log(numbers.includes(2, 2)); // Output: true (search starts at index 2)
console.log(numbers.includes(2, 4)); // Output: false (search starts at index 4)
Case Sensitivity
The includes() method is case-sensitive.
Example:
const names = ["Alice", "Bob", "Charlie"];
console.log(names.includes("alice")); // Output: false
console.log(names.includes("Alice")); // Output: true
Conclusion
Understanding the fundamentals of arrays in JavaScript is essential for working with data efficiently. This includes knowing how to use indexing to access elements, length to calculate the array size and includes() to check for the existence of an element. Developing dynamic applications and managing more complex tasks will be easier with these tools.
Master the art of working with JavaScript arrays! For any support or suggestions, feel free to reach out to StaticMania. Happy coding! 🚀