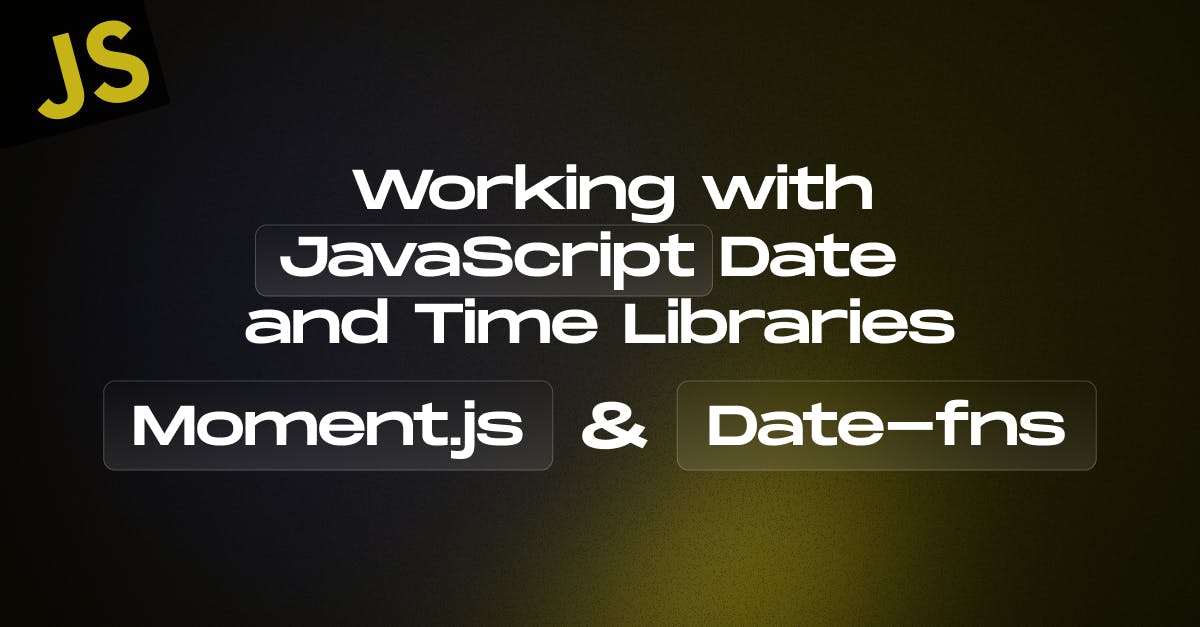
Working with JavaScript Date and Time Libraries: Moment.js and Date-fns
- Author: Md. Saad
- Published at: March 02, 2025
- Updated at: March 04, 2025
Working with dates and times in JavaScript can be challenging due to the complexities of time zones, formatting, and manipulation. Thankfully, libraries like Moment.js and Date-fns simplify these tasks. In this blog, we’ll explore libraries, their features and how to use them effectively.
Understanding Moment.js
Moment.js is one of the most popular libraries for handling dates and times in JavaScript. It provides powerful methods for parsing, formatting, and manipulating dates.
Installing Moment.js
To use Moment.js, install it via npm:
npm install moment
Include it in an HTML file:
<script src="https://cdnjs.cloudflare.com/ajax/libs/moment.js/2.29.4/moment.min.js"></script>
Key Features of Moment.js
- Parsing dates from different formats
- Formatting dates easily
- Handling time zones with Moment Timezone
- Relative time calculations (e.g., "3 days ago")
Basic Usage
const moment = require('moment');
// Get the current date
console.log(moment().format('YYYY-MM-DD HH:mm:ss'));
// Parse a date
const date = moment("2025-02-15", "YYYY-MM-DD");
console.log(date.format('dddd, MMMM Do YYYY'));
// Add days
console.log(moment().add(7, 'days').format('YYYY-MM-DD'));
// Get relative time
console.log(moment("2025-02-10").fromNow());
Downsides of Moment.js
- Large library size (~300 KB)
- Mutability issues (modifying a Moment instance changes the original.)
- No longer actively maintained (considered legacy)
Understanding Date-fns
Date-fns is a modern JavaScript date utility library that is modular, lightweight, and faster than Moment.js.
Installing Date-fns
To use Moment.js, install it via npm:
npm install date-fns
Include it in an HTML file:
<script src="https://cdnjs.cloudflare.com/ajax/libs/moment.js/2.29.4/moment.min.js"></script>
Key Features of Date-fns
- Modular (import only what you need)
- Immutable functions (does not modify original date objects)
- Better performance compared to Moment.js
- Supports time zones via date-fns-tz
Basic Usage
const { format, parse, addDays, differenceInDays } = require('date-fns');
// Get the current date
console.log(format(new Date(), 'yyyy-MM-dd HH:mm:ss'));
// Parse a date
const date = parse('2025-02-15', 'yyyy-MM-dd', new Date());
console.log(format(date, 'EEEE, MMMM do yyyy'));
// Add days
console.log(format(addDays(new Date(), 7), 'yyyy-MM-dd'));
// Get the difference in days
console.log(differenceInDays(new Date(), new Date('2025-02-10')));
Downsides of Date-fns
- Some functions require explicit date-fns-tz for time zone handling
- Less built-in localization support compared to Moment.js
Moment.js vs. Date-fns: Which One Should You Choose?
Choosing between Moment.js and Date-fns depends on your project’s requirements. Below is a detailed comparison to help you decide:
Aspect | Date-fns | Moment.js |
---|---|---|
Library Size |
Compact, especially with tree-shaking (~30 KB) |
Larger and monolithic (~300 KB) |
Performance |
Faster due to immutability |
Slower due to mutable objects |
Modularity |
Highly modular; import only what’s needed |
Requires importing the full library |
Ease of Use |
Functional, concise methods |
Chainable API, intuitive |
Time Zone Support |
Basic support via date-fns-tz |
Extensive support with Moment-Timezone |
Internationalization |
Strong support |
Strong support |
String Parsing |
Simple parsing capabilities |
Advanced parsing features |
Maintenance |
Actively maintained, modern approach |
No longer maintained (deprecated) |
Immutability |
Immutable functions |
Mutable objects |
Key Takeaways
- Choose Date-fns if you need a lightweight, fast, and modular solution with modern best practices.
- Choose Moment.js if your project is already built with it and migration isn’t feasible, or if you require advanced time zone handling.
- Consider Luxon if you need a modern alternative to both libraries with strong timezone and formatting support.
Ultimately, Date-fns is the better choice for new projects, while Moment.js remains useful for maintaining older applications.
Best Practices: When to Use Moment.js
- If your project already uses Moment.js and migrating is not feasible,.
- When you require built-in internationalization and localization support.
- If you need a simple way to handle relative time calculations (e.g., "3 days ago").
- When working with time zones using Moment Timezone.
Best Practices: When to Use Date-fns.js
- If you need a lightweight and fast library.
- When working with modern JavaScript frameworks that favor tree-shaking and modular imports.
- If you require immutable functions that don’t modify the original date object.
- When performance is a key concern in your application.
- If you prefer a library that is actively maintained and regularly updated.
Conclusion
Both Moment.js and Date-fns are powerful libraries for handling date and time operations in JavaScript. However, with Moment.js being deprecated and Date-fns offering a lightweight, modular, and immutable approach, Date-fns is the preferred choice for new projects. That said, switching may not always be necessary if your application already relies on Moment.js, especially if it meets your current needs.
Ultimately, the best choice depends on your project’s specific requirements. If performance, maintainability, and modern JavaScript practices matter most, Date-fns is the way to go. Otherwise, for legacy support and advanced time zone handling, Moment.js still has its place.
Need help with Javascript problems in your project? Get in Touch With Us Today!
FAQ Section – Working with JavaScript Date and Time Libraries: Moment.js and Date-fns
Moment.js is a legacy, large-sized library with mutability issues but provides strong time zone support. Date-fns is a modern, lightweight, immutable, and modular alternative with better performance and active maintenance.
Moment.js is deprecated and no longer actively maintained. It has a large file size (~300 KB), mutability issues, and lacks modern JavaScript optimizations like tree-shaking. Libraries like Date-fns and Luxon are better alternatives.
In Moment.js, use moment().format('YYYY-MM-DD'). In Date-fns, use format(new Date(), 'yyyy-MM-dd'). Date-fns is more modular, allowing you to import only what’s needed for better performance.
Moment.js (with Moment Timezone) offers extensive time zone handling, making it suitable for applications requiring precise time zone conversions. Date-fns requires an additional package (date-fns-tz) for time zone support but is more lightweight.
Use Moment.js if your project already relies on it and migration is not feasible or if you require advanced time zone handling and built-in localization. However, for new projects, Date-fns is the better choice due to its performance and maintainability.
Date-fns is the best alternative due to its lightweight, modular, and immutable design. Luxon is another great choice if you need advanced time zone and internationalization support with a modern API.