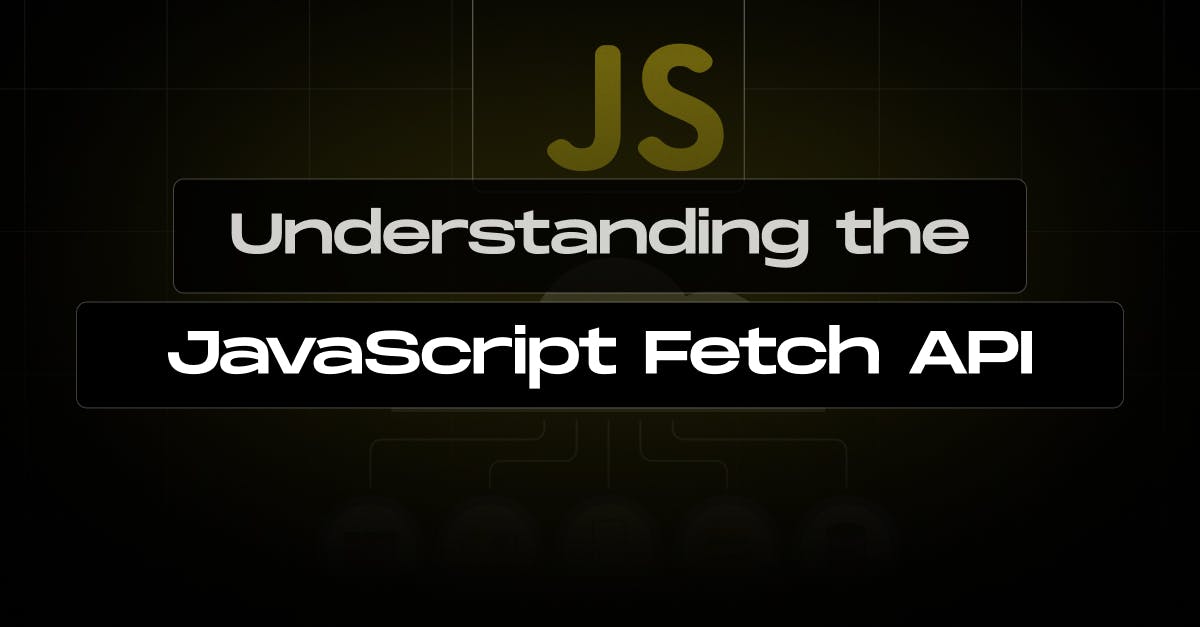
Understanding the JavaScript Fetch API
- Author: Md. Saad
- Published at: January 19, 2025
- Updated at: January 20, 2025
Accessing API data is the most important aspect of web development. We use Fetch to get this data. Fetch is a JavaScript function that allows you to query any URL/API and return data. Another significant part is that fetch is asynchronous, meaning it will run in the background and notify you when it is finished via promises. This article will discuss how to use the Javascript Fetch API.
What is the Fetch API?
The Fetch API offers a JavaScript interface for handling HTTP requests and processing their responses. Generally, the Fetch API functions similarly to XMLHttp Request but has a more up-to-date syntax and features. Since Fetch is a promise-based API, it makes asynchronous code easier to understand and works well with async/await and other contemporary JavaScript capabilities.
How the Fetch API Works
With the Fetch API, you request by calling the fetch() function and providing the target URL as the first argument:
fetch('<Your URL>', {})
The fetch() function takes two parameters:
- URL (required): The destination for your request.
- Options (optional): An object where you can specify request settings, such as the HTTP method (e.g., GET, POST, etc.).
When you call fetch(), it returns a promise. Then, you need to use the .then() method to handle the response when the request succeeded and the .catch() method to manage any errors that occur. Here's an example:
fetch('<Your URL>', {})
.then(response => {
// Process the response here
})
.catch(error => {
// Handle any errors here
});
In the .then() and .catch() methods, you pass a callback function that will execute when those methods are triggered.
Note: The .catch() method is optional. It is mainly used for errors that prevent the request from being made, such as network issues or an invalid URL.
Making a POST Request
Though fetch() makes a GET request, you can use the method option to use a different request method such as POST, PUT, DELETE, PATCH, etc. So, you have to add method option. Furthermore, whenever you send a post request to an API, you have to add header and body properties to work it properly. Let’s add the content-type : application/json at the header and the data that you want to put in the body as a JSON format. Your request should look like the following codes:
fetch('https://api.example.com/submit', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
name: 'John Doe',
email: 'john.doe@example.com'
})
})
.then(response => {
if (!response?.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
return response.json();
})
.then(data => {
console.log('Success:', data);
})
.catch(error => {
console.error('Error:', error);
});
In this example:
- method: Specifies the HTTP method. Here it is POST but can be (PUT, DELETE, PATCH).
- headers: Sets custom headers, like Content-Type.
- body: Contains the request payload, typically as a JSON string.
Making a Basic GET Request
By default, fetch() makes a GET request. GET requests are commonly used to retrieve data from a server, such as user information, product details, or other resources. They are the most straightforward type of HTTP request, often used when no additional data needs to be sent to the server.
Here’s how you can use the Fetch API to make a GET request to an API endpoint:
fetch('https://api.example.com/data')
.then(response => {
if (!response?.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
return response.json();
})
.then(data => {
console.log(data);
})
.catch(error => {
console.error('There was an error!', error);
});
Explanation:
- fetch(url): Sends a request to the given URL.
- .then(response => {...}): Processes the response object.
- Check response?.ok to ensure the request was successful.
- Use response.json() to parse the response body as JSON.
- .catch(error => {...}): Catches and handles any errors that occur during the fetch or in the .then() chain.
Making requests using Async/Await
For cleaner and more readable code, you can use the Async/Await syntax to replace the .then() and .catch() methods. Here is an example of basic get methods using Async/Await.
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
if (!response?.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
const data = await response.json();
console.log(data);
} catch (error) {
console.error('There was an error!', error);
}
}
fetchData();
Using Try, Catch, and Finally
The finally block can also be used in combination with try and catch for a more structured and readable approach. Here is an example:
async function fetchDataWithFinally() {
try {
console.log('Starting request...');
const response = await fetch('https://api.example.com/data');
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error:', error);
} finally {
console.log('Request completed');
// Hide loading spinner or perform cleanup tasks
}
}
fetchDataWithFinally();
Use Cases for Fetch API
There are many instances in which the Fetch API can be applied. Some typical use cases are as follows:
1. Fetching Data from an API
The most common use case is getting data from a server, such as loading user information, weather details, news, articles, and so on.
Example:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error fetching data:', error));
2. Submitting Form Data
You can send form data to a server using the POST method with the Fetch API.
Example:
const formData = {
name: 'John Doe',
email: 'john.doe@example.com'
};
fetch('https://api.example.com/submit', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(formData)
})
.then(response => response.json())
.then(data => console.log('Form submitted:', data))
.catch(error => console.error('Error submitting form:', error));
3. Updating or Deleting Resources
Using methods like PUT, PATCH, or DELETE, you can modify or remove resources on a server.
Example:
// Updating data
fetch('https://api.example.com/resource/123', {
method: 'PUT',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ name: 'Updated Name' })
})
.then(response => response.json())
.then(data => console.log('Resource updated:', data))
.catch(error => console.error('Error updating resource:', error));
// Deleting data
fetch('https://api.example.com/resource/123', {
method: 'DELETE'
})
.then(response => {
if (!response?.ok) {
throw new Error('Error deleting resource');
}
console.log('Resource deleted');
})
.catch(error => console.error('Error deleting resource:', error));
4. Uploading Files
The Fetch API can handle file uploads using the FormData object.
Example:
const fileInput = document.querySelector('#fileInput');
const formData = new FormData();
formData.append('file', fileInput.files[0]);
fetch('https://api.example.com/upload', {
method: 'POST',
body: formData
})
.then(response => response.json())
.then(data => console.log('File uploaded:', data))
.catch(error => console.error('Error uploading file:', error));
5. Handling Errors
Error handling is crucial in the web development world. The Fetch API simplifies the error handling. Common issues in error handling include:
- Network failures, such as when the server is unreachable.
- Non-2xx HTTP responses (e.g., 404 for not found or 500 for server errors).
- Timeouts, which occur when the server takes too long to respond.
- Unauthorized access due to missing or invalid credentials.
fetch('https://api.example.com/invalid-url')
.then(response => {
if (!response?.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
return response.json();
})
.catch(error => {
console.error('Fetch error:', error.message);
});
Advanced Features
The Fetch AP also offers many advanced features that deal with complex use cases, such as setting custom headers, handling authentication, or uploading files. These features enable developers to work with contemporary APIs and optimize their network queries. For example, to work with Headers, you can use the headers object to customize requests:
fetch('https://api.example.com/data', {
headers: {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Accept': 'application/json'
}
})
.then(response => response.json())
.then(data => console.log(data));
Conclusion
Learning how to use APIs is a necessary skill for web developers, and the Fetch API provides a simple yet powerful approach to interacting with external data sources. You may improve your grasp of acquiring and handling data using JavaScript by practising and applying these principles to real-world tasks. Have fun coding!
Do you have any questions about using the JavaScript Fetch API or need professional assistance with your web development projects? Feel free to reach out to StaticMania. We're here to help with all your development needs!
FAQs of JavaScript Fetch API
The Fetch API is a built-in JavaScript interface to make HTTP requests and handle responses, offering a modern way to retrieve and send data over the web.
Yes. Fetch uses modern JavaScript features like promises and async/await, making code more readable, while AJAX often relies on more traditional callback-based approaches.
After calling the Fetch API, check if the response is successful, then parse the response data as JSON for easier handling in your application.
You can specify a request method, headers, and the data to be sent in the body. This makes it simple to submit information to servers or APIs.
By checking if the response was successful and catching any issues that may arise, you can gracefully handle network failures or server-side problems.
Yes. Wrapping Fetch calls in an async function and using await allows you to write asynchronous code that’s easier to read and maintain.
Some older browsers do not support Fetch natively, but adding a polyfill (a piece of code that provides modern functionality in older environments) solves this compatibility issue.