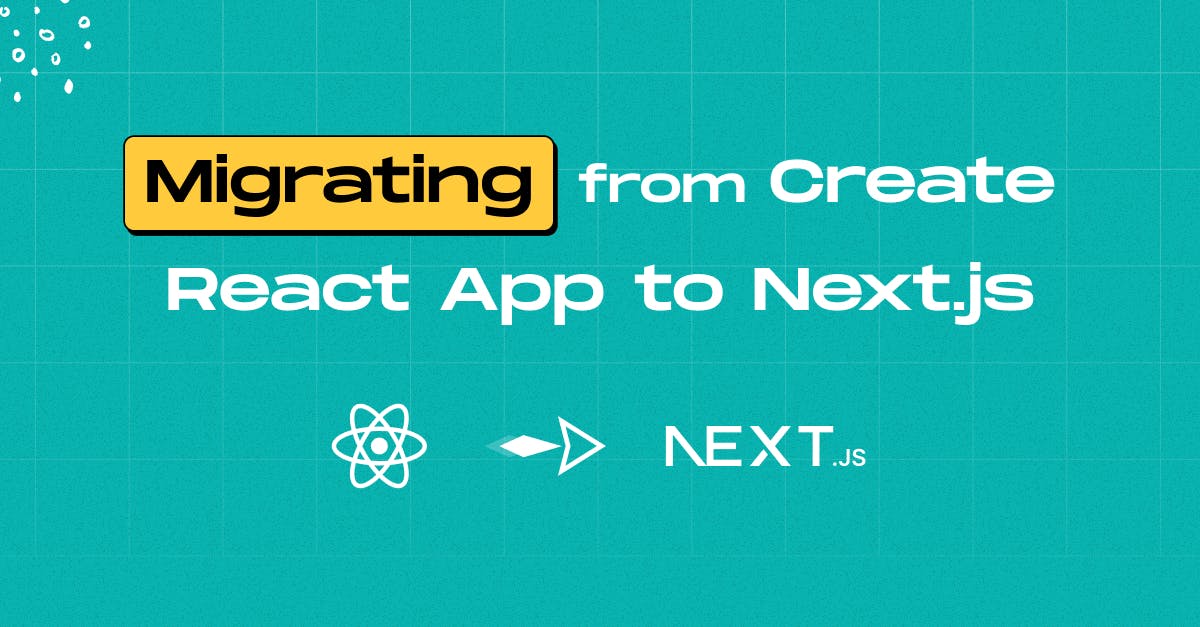
Migrating from Create React App to Next.js
- Author: Md. Saad
- Published at: January 28, 2024
- Updated at: June 22, 2025
Transforming an existing React app into a Next.js app can be highly rewarding, enhancing your application's speed, SEO, and overall user experience. The process involves several steps. In this article, we provide a detailed guide to assist you throughout the entire migration:
1. Update Package.json Scripts:
The initial step in migrating to Next.js is to update the package.json and dependencies. Adjust the package.json scripts to align with Next.js equivalents. Replace the "scripts" section in your package.json with the following:
```json
"scripts": {
"dev": "next",
"build": "next build",
"start": "next start",
"lint": "next lint"
}
```
After updating the scripts section, add the required dependencies:
```json
"dependencies": {
"next": "^14.0.4",
"react": "^18.2.0",
"react-dom": "^18.2.0"
// ADD OTHER DEPENDENCIES
}
```
(Note: The versions of next, react, and react-dom packages may have been updated since the publication of this article.)
Delete your node_modules folder and reinstall all dependencies using your preferred package manager.
2. Static Assets and Compiled Output:
Transfer images, fonts, and other static assets from your Create React App's public directory to the public directory in your new Next.js project.
Delete the index.html file from the public directory and create a new "app" folder in the "src" folder. Inside the "app" directory, create three new files called globals.css, layout.jsx, and page.jsx.
Move all code from your index.css file to the globals.css file.
Add the following code to your layout.jsx file:
```jsx
export const metadata = {
title: 'Next.js',
description: 'Generated by Next.js',
}
export default function RootLayout({ children }) {
return (
<html lang="en">
<body>{children}</body>
</html>
)
}
```
The page.jsx file in your "app" directory serves as your default home page in Next.js version 14. Return something from this page.jsx file, like:
```jsx
export default Page = () => {
return <section>Home Page</section>
}
```
Ensure to add ".next" to your. git ignore file to exclude the Next.js build directory from version control.
Learn Similar Article: How to Migrate Your Site to JAMstack in 2025
3. Transfer Routes:
In React, we use react-router-dom for routing, while Next.js utilizes file-based routing. To transfer, follow these steps:
Move your React components to the "components" directory in your Next.js project. This step is optional but can help organize your code.
In the "app" directory of your Next.js app, create a folder corresponding to your existing routes. For example:
If you have an "about" page, create a folder called "about" inside the "app" directory. Inside the "about" folder, create a new file called "page.jsx." Now, you can run your application and navigate to your created folder, like:
`http://localhost:3000/about`
If your React app used a navigation library like react-router-dom, replace it with Next.js's Link component:
Replace this in your React app:
```jsx
import { Link } from 'react-router-dom'
```
With this in your Next.js app:
```jsx
import Link from 'next/link';
<Link href="/about">About</Link>
```
4. Transfer Search Engine Optimization Data:
Next.js has a Metadata API that defines your application metadata inside the HTML head element for improved SEO and web shareability. Utilize it as follows:
```jsx
export const metadata = {
title: '...',
description: '...',
}
```
Congratulations! You have successfully migrated your React app to Next.js. If you encounter any issues, please feel free to contact us. Our dedicated development team is here to assist you at every step of your journey.