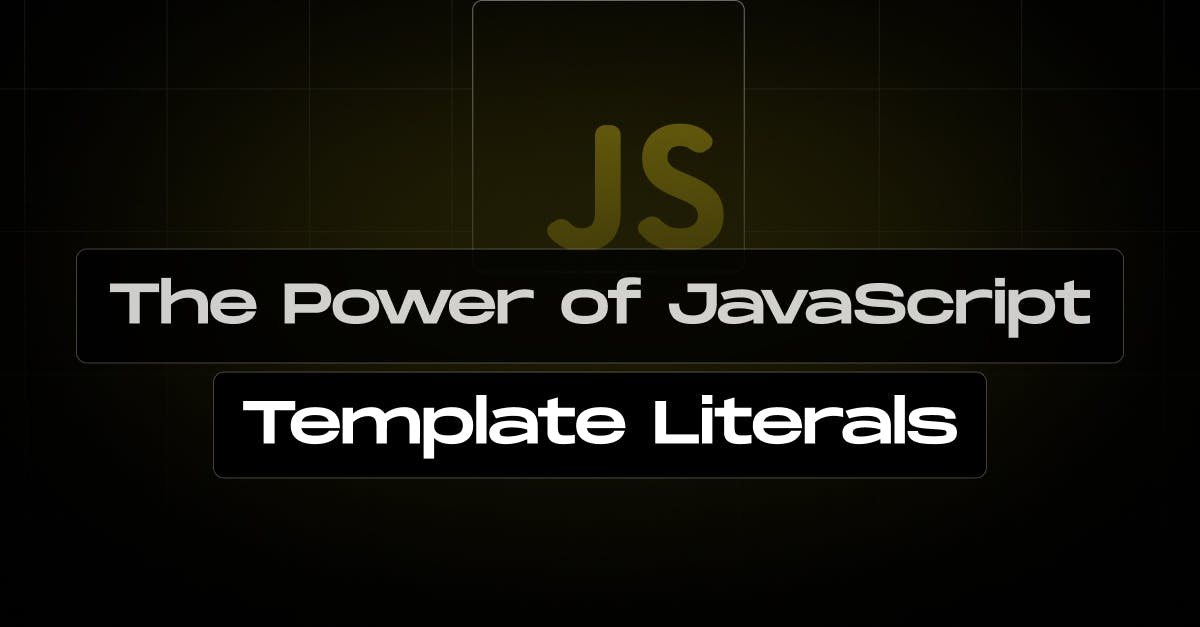
The Power of JavaScript Template Literals
- Author: Md. Saad
- Published at: January 05, 2025
- Updated at: January 06, 2025
Introduction
JavaScript, a versatile and constantly evolving language, has introduced many features since its introduction in ES6. Template literals are one of the most useful tools available to developers in JavaScript. They enhance how we work with strings, making our code cleaner, more readable, and more dynamic. Let's examine why template literals are so effective and look at real-world applications for them.
What Are Template Literals?
Template literals are a feature in Javascript that is an alternative to traditional string concatenation. Generally, we use double quotes " " or single quotes '' to declare regular strings in Javascript. But in template literals, backticks `` are used to denote a string. Besides, it serves far more than a regular string. For example, this allows multi-line strings, string interpolation with embedded expressions, and special constructs called tagged templates.
Template Literals Syntaxes:
`sample template literals text`
`sample template literals text line 1
sample template literals text line 2`
`sample ${expression} text`
tagFunction`sample text ${expression} text`
Template Literals Parameters:
Template literals consist of:
- String text: The literal text within the template. Almost all characters are allowed, including line breaks and whitespace. Invalid escape sequences will result in a syntax error unless a tag function is applied.
- Expression: A dynamic value inserted into the template at a specified position. The expression's value is converted to a string or passed to a tag function if one is defined.
- Tag function: An optional function that processes the template strings and expressions. The tag function's return value determines the template's final output literal. For more details, see tagged templates.
Template Literals Features
Template Literals come with several advanced features:
- String interpolation
- Multi-line strings
- Embedding expressions
- Tagged templates
Let’s look into them:
String Interpolation: A primary benefit of template literals is string interpolation. By doing this, you can include variables and expressions straight into your strings, getting rid of the tedious concatenation operators (+).
In a traditional concatenation way, we use the following example:
const name = "Shelly";
const age = 35;
// Traditional concatenation
const greeting = "Hello, my name is " + name + " and I am " + age + " years old.";
That can be difficult to read, especially when there are multiple expressions. Using template literals, the same result can be generated with this:
const name = "Shelly";
const age = 35;
// Using template literals
const greeting = `Hello, my name is ${name} and I am ${age} years old.`;
console.log(greeting);
The syntax ${expression} within a template literal improves code clarity and expressiveness.
Multi-Line Strings
Before ES6, creating multi-line strings needed laborious methods such as using newline characters (\n) or concatenating strings between lines. Template literals make this easier by allowing true multi-line strings.
Examples:
Without template literals:
// Without template literals
const paragraphWithoutTemplate = "This is a line.\n" +
"This is another line.\n" +
"Yet another line.";
console.log(paragraphWithoutTemplate );
With template literals:
// With template literals
const paragraphWithTemplate = `This is a line.
This is another line.
Yet another line.`;
console.log(paragraphWithTemplate);
Embedding Expressions:
In addition to variables, any javascript expression can be embedded within a template literal. This includes arithmetic operations, function calls, and even ternary expressions.
const number1 = 30;
const number2 = 40;
const result = `The sum of ${number1} and ${number2} is ${number1 + number2}.`;
console.log(result);
// Embedding function calls
const getCurrentYear = () => new Date().getFullYear();
const message = `The current year is ${getCurrentYear()}.`;
console.log(message);
Tagged Template Literals:
JavaScript template literals have a powerful feature that allows for advanced string manipulation using tagged templates. This feature involves two parts: a tag function and the template literal itself.
- The tag function receives an array of strings and the values of the placeholders in the template literal as arguments. It processes them based on your custom logic or formatting rules.
- To apply the tag function, write it directly before the backtick that starts the template literal. This allows the function to handle and format the content dynamically.
Examples:
function highlight(strings, ...values) {
return strings.map((str, i) => `${str}<strong>${values[i] ?? ""}</strong>`).join("");
}
const name = "Alice";
const age = 25;
const result = highlight`My name is ${name} and I am ${age} years old.`;
console.log(result);
// Output: My name is <strong>Alice</strong> and I am <strong>25</strong> years old.
Practical Use Cases of Template Literals
So far, you have learned what template literals are and how they differ from regular strings. Let's take a look at some practical examples.
HTML Markups
In Javascript, it is often seen to use template literals to generate HTML markup. They allow you to add JavaScript expressions directly to HTML strings. Thus, building HTML strings dynamically becomes much simpler with template literals.
const title = "Welcome";
const body = "This is a dynamic HTML content.";
const html = `
<div>
<h1>${title}</h1>
<p>${body}</p>
</div>
`;
console.log(html);
Internalization:
Internationalization (often abbreviated as i18n) is the process of designing applications to support multiple languages and regions. can simplify the process of building multilingual applications.
const user = {
name: "Rina",
};
const language = "es"; // Language code for German
const greetings = {
en: "Hello",
es: "Hola",
fr: "Bonjour",
de: "Hallo",
it: "Ciao"
};
const localizedGreeting = `${greetings[language]} ${user.name}!`;
console.log(localizedGreeting);
// Output: Hola Rina
Dynamic File Paths or URLs
Constructing file paths or URLs dynamically based on user input or configuration becomes simpler with template literals.
Example:
const userId = 12345;
const baseUrl = "https://api.example.com";
const apiEndpoint = `${baseUrl}/users/${userId}/profile`;
console.log(apiEndpoint); // Output: https://api.example.com/users/12345/profile
Conclusion
Template literals empower developers to write cleaner, more expressive, and more maintainable code. They have become a must-use tool in modern JavaScript development by offering features like string interpolation, multi-line support, and tagging. Whether you’re building a simple application or a complex system, leveraging template literals can enhance the clarity and functionality of your code. Embrace the power of template literals, and watch your JavaScript projects become more elegant and efficient!
Need help with a JavaScript solution? Look no further than StaticMania for premium services. Our expertise covers various solutions: custom web development, e-commerce solutions, UI/UX design, headless CMS integration, performance optimization, and SEO-friendly static websites.
FAQs
Template literals are a feature introduced in ES6 that allows developers to create strings using backticks ( ) instead of single (') or double (") quotes. They support string interpolation, multi-line strings, and embedding expressions directly into strings, making code more readable and dynamic.
Unlike traditional strings, template literals use backticks and support:
- String interpolation using ${expression}.
- Multi-line strings without needing escape sequences.
- Tagged templates for advanced string manipulation.
Template literals make code more concise, readable, and easier to maintain. Features like interpolation, multi-line support, and tagging reduce complexity and improve functionality in JavaScript applications.
Template literals simplify how developers work with strings, enabling dynamic content insertion, multi-line strings, and advanced formatting options. They help make code more readable and maintainable.
Template literals were introduced with ES6 (ECMAScript 2015), marking a significant improvement in JavaScript's handling of strings.
Template literals allow seamless integration of expressions and variables into strings. This is especially useful for tasks like generating HTML content, URLs, or localized messages dynamically.