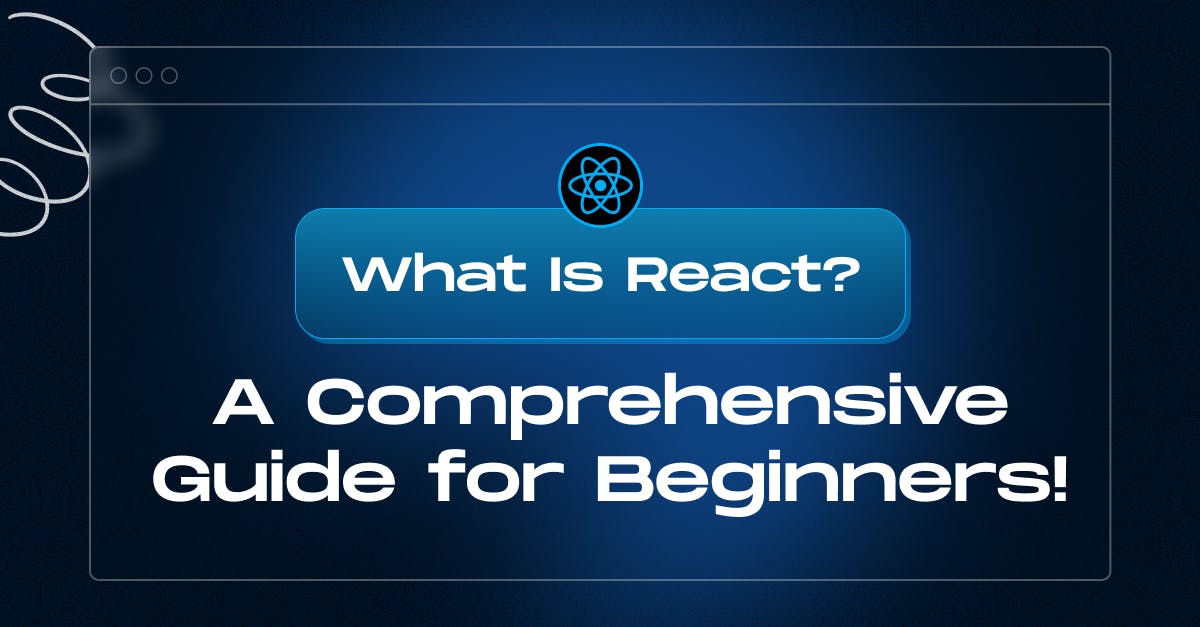
What Is React? A Comprehensive Guide for Beginners!
- Author: Nusrat Sarmin
- Published at: July 11, 2024
- Updated at: July 11, 2024
Imagine a world where building web interfaces is like playing with Lego – you snap together pre-built components to create dynamic, lively UIs that keep users Enthralled. That's the magic of React!
This guide aims to provide a comprehensive overview of what is React, its core concepts, and how to get started with building applications using React.
What Is React?
React is a popular JavaScript library for building user interfaces, particularly single-page applications.
While React might seem ubiquitous today, its journey began in 2011. Fueled by the vision of Jordan Walke, a software engineer at Facebook, React emerged as a fresh take on building user interfaces. React was first released in 2013 and has since become one of the most widely used libraries in front-end development.
React has gained immense popularity due to its simplicity, flexibility, and performance. It allows developers to create reusable UI components, leading to faster development, easier maintenance, and cleaner code.
Moreover, React is not just limited to web development. With React Native, you can build mobile apps with the same principles and components. With React-based frameworks like Next.js and Gatsby, it is easy to build server-rendered applications.
Why Use React?
Here's a breakdown of what makes React so powerful:
👉 Component-Based: React applications are built using reusable components, like building blocks. Imagine a button, a navigation bar, or a product card - these can all be individual React components. This makes it easier to organize and maintain code.
👉 Focus on UI: React is primarily concerned with building user interfaces (UI) rather than the entire application logic. It excels at creating dynamic and responsive UIs that update efficiently.
👉 Virtual DOM: React uses a concept called the Virtual DOM, which is an efficient way to represent the UI. When changes are made, React updates the Virtual DOM first, then efficiently updates the real DOM in the browser, minimizing unnecessary re-renders.
👉 Declarative Approach: React adopts a declarative approach, where you describe what the UI should look like for a given state. React handles the rendering and updating of the UI when the state changes. This makes code more readable and predictable.
Core Concept and Other Features of React
1. Components
Components are the building blocks of a React application. They encapsulate the UI and behavior of a part of the interface, which can be reused and nested within other components. There are two main types of components in React: Functional Components and Class Components.
- Functional Components: These are lightweight components defined as simple JavaScript functions. They receive props as arguments and return JSX that describes the UI. Functional components are ideal for presentational components that focus on UI rendering without the need for state management. For example, a simple Button component that displays text and handles clicks can be implemented as a functional component.
- Class Components: Class components provide more capabilities compared to functional components. Class components are ES6 classes that extend React.Component and have a render method that returns React elements.. While slightly more complex than functional components, class components are suitable for components that require complex logic or state management. Imagine a form component that tracks user input and validates data. This would likely be implemented as a class component.
class Welcome extends React.Component {
render() {
return <h1>Hello, {this.props.name}</h1>;
}
}
2. JSX
JSX is a syntax extension that allows writing HTML-like code within JavaScript. It is not a requirement for using React, but it makes code more readable and easier to write. Under the hood, JSX gets compiled into React.createElement calls, which create the React elements.
JSX provides the power of JavaScript within HTML-like syntax, allowing you to use JavaScript expressions, functions, and event handling directly in your markup.
const element = <h1>Hello, world!</h1>;
// Compiled to:
// const element = React.createElement('h1', null, 'Hello, world!');
3. Virtual DOM
The Virtual DOM is one of React's key performance optimizations. It is a lightweight in-memory representation of the actual DOM. When the state of a component changes, React updates the Virtual DOM first. It then compares the updated Virtual DOM with a snapshot taken before the update (a process called "reconciliation"). And calculates the most efficient way to update the actual DOM. This minimizes the number of direct DOM manipulations, which are expensive in terms of performance.
4. State
State is a JavaScript object that holds dynamic data and determines the behavior of a component. Components can maintain their own internal data using state. This data can be anything from user input to fetched data from an API. When the state changes, React automatically re-renders the component and its children, ensuring the UI reflects the latest data. Think of a counter component that keeps track of its current value using state. When the user clicks a button, the state updates, and React re-renders the component to display the new counter value.
5. Props
Components communicate with each other by passing data through props. Props are read-only attributes passed from parent to child components. They are used to pass data and event handlers down the component tree. Props help make components reusable and maintain a clear data flow within the application.
6. Lifecycle Methods
Lifecycle methods are special methods in class components that allow you to run code at specific points in the component's lifecycle: mounting, updating, and unmounting.
- ‘componentDidMount()’: When a component is being inserted into the DOM.
- ‘componentDidUpdate()’: When a component is being re-rendered due to changes in props or state.
- ‘componentWillUnmount()’: When a component is being removed from the DOM.
7. Hooks
Hooks are functions that let you use state and other React features in functional components. Introduced in React 16.8, hooks enable functional components to manage state, handle side effects, and use lifecycle methods without converting them to class components. Some commonly used hooks are useState, useEffect, and useContext.
8. Data Binding
React uses one-way data binding, meaning data flows in a single direction, from parent to child components. This makes the data flow more predictable and easier to understand, which simplifies debugging. If a child component needs to communicate back to a parent component, it does so via callbacks passed through props.
9. Debugging
React provides powerful tools for debugging, such as the React Developer Tools extension for browsers. This extension allows developers to inspect the component tree, view the state and props, and track component updates. Additionally, the declarative nature of React makes it easier to trace and debug issues as the UI consistently reflects the state.
10. React Router
React Router is a library used to handle routing in React applications. It allows developers to create multi-page applications with dynamic routing, where different URLs map to different components. React Router provides components like BrowserRouter, Route, Link, and Switch to manage the navigation and rendering of components.
Benefits of Using React Library
- Faster Development: React's component-based structure makes it easy to reuse code. You can build pre-made components for common UI elements and use them across your app, saving you time and effort. Instead of writing the same code repeatedly, you just use these components. Plus, React's declarative style lets you specify what the UI should look like for a given state, making development simpler.
- Improved Maintainability: Clean code structure due to isolated components makes maintenance easier. Dividing the UI into independent, reusable components results in cleaner, more organized code. This makes your application easier to understand, modify, and debug, especially in larger projects with multiple developers.
- Enhanced Performance: React's virtual DOM is key to improving performance. It acts as an in-memory version of the real DOM, showing the UI. When changes happen, React quickly figures out the small differences between the virtual DOM and the real DOM. This reduces the number of changes needed in the actual DOM, resulting in smoother and faster UI updates.
- SEO-Friendly Applications: React applications can be SEO-friendly with the right approach. By default, React uses client-side rendering (CSR), which can make it harder for search engines to crawl and index your content. However, using techniques like server-side rendering (SSR) or static site generation (SSG) can make React applications SEO-optimized.
- Large Community and Ecosystem: Extensive resources, libraries, and tools are readily available. React has a large and active developer community. This means you can find many resources, libraries, and tools to help you with your development. There are lots of online tutorials, courses, and forums available to help you learn and solve any problems you face.
- Learn Once, Write Anywhere: React's core concepts can be applied across different platforms. Developers can use React to build web applications, and with React Native, they can create mobile applications for iOS and Android. This flexibility allows for a smoother transition between web and mobile development.
Pros and Cons of React.js
Pros | Cons |
---|---|
|
|
Uses-cases of React
- Interactive and Complex UIs: React excels at creating dynamic and interactive web interfaces. If your application has a lot of user interaction, React can streamline development.
- Single-Page Applications (SPAs): React is ideal for building SPAs, where different parts of a webpage are dynamically updated without reloading the whole page. SPAs built with React feel fast and responsive. Examples include Netflix, Facebook, and Gmail.
- Mobile Applications: React Native, a framework derived from React, allows developers to build mobile applications for iOS and Android using React. React Native shares many of the same principles as React, making it easier for web developers to transition to mobile development.
- Web Development: React is used extensively in web development to create interactive and dynamic user interfaces. Many high-traffic websites and applications use React due to its performance benefits and ease of use.
- E-Commerce: React is well-suited for e-commerce sites, which require dynamic and responsive interfaces to handle product searches, filtering, and cart management.
- Dashboard and Data Visualization: React's component-based architecture makes it ideal for building complex dashboards and data visualization tools, where different components can update independently based on real-time data.
- Real-Time Applications: React's ability to handle frequent UI updates makes it suitable for real-time applications like chat or collaboration tools. The virtual DOM minimizes the number of DOM manipulations needed, keeping the UI performant.
- eCommerce Applications: React's strength in interactive UIs and reusable components makes it ideal for building dynamic e-commerce sites. It can create a smooth user experience with features like shopping carts and product filtering. The component-based approach promotes code reusability for common elements throughout the store. However, it's important to remember that React focuses on the front end. It needs to be paired with a backend solution for things like product data and order processing.
The Future of React.js
React has become a dominant force in front-end development, but what does the future hold for this popular JavaScript library? Let's explore some key trends shaping the React landscape and how they'll influence web development in the years to come.
✅ Greater Emphasis on Performance: Future versions of React are likely to focus even more on performance optimization, including advancements in Server Components and Concurrent Mode.
✅ Improved Developer Tools: Expect continued enhancements in developer tools and frameworks, making it easier to build, test, and deploy React applications.
✅ Expansion of React Native: React Native's ecosystem will grow, providing more tools and libraries for building cross-platform applications with a single codebase.
✅ Server-Side Rendering on the Rise
SSR in React generates initial HTML on the server, improving SEO and load times. With frameworks like Next.js becoming increasingly popular, we can expect SSR to play a bigger role in the React ecosystem.
✅ WebAssembly: React's development team explores new territories, including WebAssembly (WASM) integration. WASM enables code compilation from languages like C++ for efficient browser execution. This feature enhances React's capabilities for demanding applications by enabling complex computations without performance loss.
✅ Concurrent Mode: Another key trend is Concurrent Mode. It helps prioritize urgent tasks over background tasks for smoother user experiences. It improves performance and responsiveness, especially during slow network connections or complex UIs.
✅ Adoption of AI and Machine Learning: As AI and machine learning become more integrated into web applications, React will likely see libraries and tools designed to facilitate these technologies.
The Steps to Getting Started with React
Step 1: Set Up Your Development Environment: Install a code editor or IDE (Integrated Development Environment) you're comfortable with (e.g., Visual Studio Code, WebStorm).
Step 2: Install Node.js and npm: Ensure you have Node.js and npm installed on your system. They're essential for running JavaScript code outside the browser and managing dependencies.
Step 3: Create a React Project: Use create-react-app (a tool included with npm) to quickly set up a new React project with the necessary boilerplate code and dependencies:
npx create-react-app my-first-react-app
Step 4: Run the Development Server: Navigate to your project directory and start the development server:
cd my-first-react-app
npm start
This will launch a local web server, typically at http://localhost:3000/ and open your React application in your default browser.
Step 5: Start Coding React! 👊
Now that your development environment is set up and the server is running. You can start writing React code in your index.js file or the equivalent file depending on your chosen setup method.
React's Rising Stars: Companies Thriving with React
React is a very popular library and is used by a vast number of companies. Here are some notable examples:
- Meta (formerly Facebook): React was created by Facebook and is extensively used throughout their platforms, including Facebook itself and Instagram.
- Netflix: Netflix has adopted React to create a smooth and dynamic user experience for their streaming service.
- Tesla: Tesla utilizes React for both their web applications and certain in-car interfaces, taking advantage of React's ability to handle dynamic data and create interactive UIs.
- Dropbox: Dropbox leverages React for both its web and mobile apps, making use of its flexibility and performance optimization.
- PayPal: PayPal employs React for their web application to ensure a secure and user-friendly payment experience.
- Walmart: Walmart has incorporated React into its web platform to enhance the online shopping experience for customers.
- Uber: Uber's web application is built with React, contributing to a seamless user experience for booking rides.
This is just a small sampling, and there are many other companies that rely on React for their web development needs. React is popular because it's easy to learn, efficient, has a strong community, and allows for diverse applications.
Learning Resources of React
- Official React Documentation: The most comprehensive guide, covering all aspects of React development: https://react.dev/learn
- FreeCodeCamp React Course: Learn React - Full Course for Beginners
- Interactive React Tutorial: A hands-on approach for beginners: https://scrimba.com/learn/learnreact
Finale Resolution
So, what is React exactly? simply put, it's a versatile JavaScript library that lets you build interactive and dynamic UI at your fingertips.
React has become a must-have tool for web developers due to its emphasis on modularity, performance, and developer experience. By harnessing the power of react, you can create complex and scalable web application with ease. React makes development faster and more efficient.
What's more, The future of React looks promising, with ongoing advancements in performance, developer tools, and ecosystem support. As new trends and technologies emerge, React will continue to evolve, maintaining its relevance and utility in the ever-changing landscape of web development.
React is poised to adapt and lead the way, making it an excellent choice for your next project. With its growing community and vast ecosystem of resources, React equips you to craft exceptional user interfaces for the web. So, dive into the world of React and start building the web applications of tomorrow!
Dream of a website that's blazing fast, bends to your needs, and handles anything you throw at it? Ditch the limitations! StaticMania crafts websites that are adaptable, lightning-fast, and ready to conquer your online goals. Let's chat and see how we can transform your website!