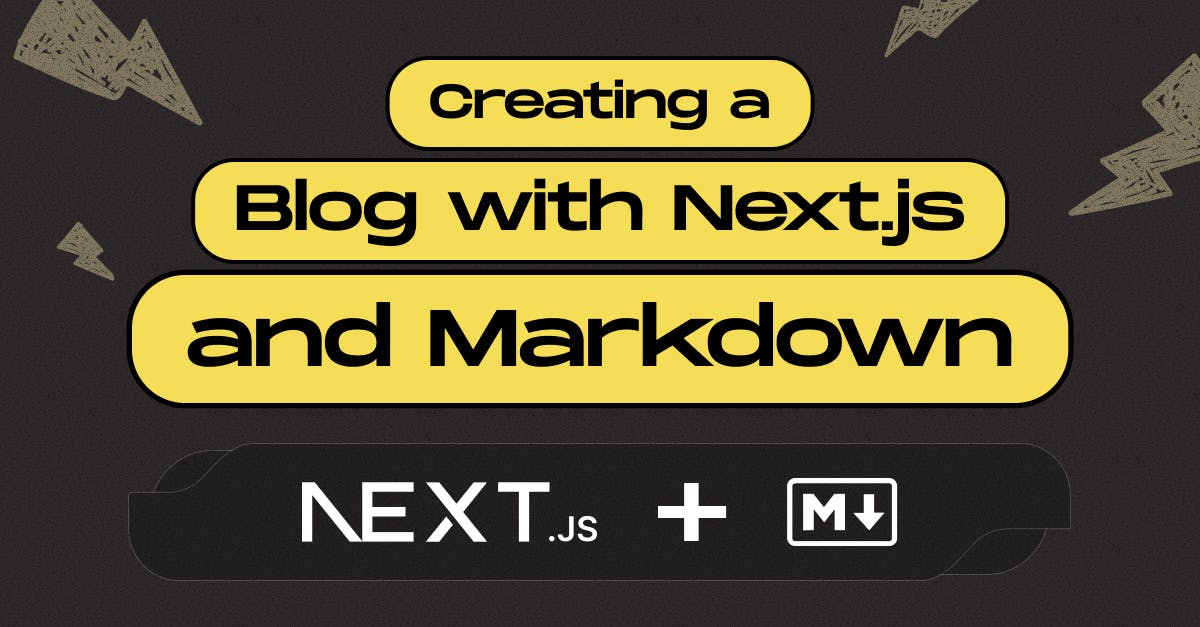
Creating a Blog with Next.js and Markdown
- Author: Md. Saad
- Published at: March 11, 2024
- Updated at: March 11, 2024
A website's blog is essential for SEO, content updates, and visitor engagement. It makes the site more visible to search engines, draws in and keeps a larger audience, and positions it as an authoritative source. With their dynamic online presence, blogs provide credibility and a sense of connection. A blog on a website is essential for increasing traffic, raising search engine results, and staying relevant in the cutthroat world of the internet. Today we will discuss a step-by-step guide to creating a blog list page and a single blog page in Next.js.
Create a Next JS Theme
First, create a next.js theme by typing the following codes. Now follow the given instructions to complete the theme creation. For more info, you can read our blog Getting Started with Next.js
npx create-next-app <APP-NAME>
Create a Blog Post Directory
Now create a blog post directory in the source file naming posts(you can name it whatever you want). In this folder, all blogs will be stored. We will use markdown to write the blog. So, let’s create a sample blog in .md file. Create a file in the posts directory, e.g., first-post.md. Your code might look like the following codes:
---
title: My First Blog Post
date: '2024-01-23'
---
# My First Blog Post
This is the content of my first blog post.
Fetching Markdown Content
Now, it's time to fetch blog data. To do that, first, install a dependency named “gray matter”. It will let us parse the metadata in each markdown file.
npm install gray-matter
You must install “react-markdown” to convert the markdown data to a javascript object.
npm install react-markdown
Now, Create a utility function to fetch Markdown content. Create a utils/GetMarkDownData.js. And add the following codes. These codes will resolve the file path for blog items.
import fs from "fs";
import path from "path";
import matter from "gray-matter";
const getMarkDownData = (folder) => {
const files = fs.readdirSync(folder);
const markdownPosts = files.filter((file) => file.endsWith(".md"));
const postsData = markdownPosts.map((file) => {
const filePath = path.join(folder, file);
const content = fs.readFileSync(filePath, "utf8");
const data = matter(content);
return {
...data.data,
slug: file.replace(".md", ""),
content: data.content,
};
});
return postsData;
}
export default getMarkDownData;
For resolving blog Details page paths, create another file inside the utils folder and name it GetMarkDownContent.js. Now, add the following codes inside the file.
import fs from "fs";
import matter from "gray-matter";
const getMarkDownContent = (folder, slug) => {
const file = `${folder}${slug}.md`;
const content = fs.readFileSync(file, "utf8");
const matterResult = matter(content);
return matterResult;
};
export default getMarkDownContent;
Create a Blog Post Template
As blog data paths are ready, it's time to create blog pages. First, create a blog list page where all the blogs will be shown. So, create an app/blog/page.jsx file. Add the following codes to fetch and show the blogs.
import getMarkDownData from "@/utils/GetMarkDownData";
import Link from "next/link";
function Blog() {
const posts = getMarkDownData("posts/");
return (
<>
{posts.map((post, i) => (
<article key={i}>
<Link href={`/blog/${post.slug}`}>
<h3>{post.title}</h3>
</Link>
<span>{post.date}</span>
</article>
))}
</>
);
}
export default Blog;
You must install “react-markdown” to convert the markdown data to a javascript object. This will create a list of blogs. In which only the title has been added and it is also linked to the corresponding Details page with {post.slug}. For example, a link for our sample blog first-post.md will be generated as http://localhost:3000/blog/ first-post.
Create a Blog Details Template
So far, we have successfully created blog posts, Now create a blog details page. So, create an app/blog/[slug]/page.jsx file. Add the following codes to fetch and show each blog's details data.
import Markdown from "react-markdown";
import getMarkDownContent from "@/utils/GetMarkDownContent";
import getMarkDownData from "@/utils/GetMarkDownData";
export const generateStaticParams = async () => {
const posts = getMarkDownData("data/posts/");
return posts.map((post) => ({
slug: post.slug,
}));
};
const BlogPost = (props) => {
const folder = "posts/";
const slug = props.params.slug;
const post = getMarkDownContent(folder, slug);
const postParams = post.data;
return (
<>
<section className="blog-details">
<h2 className="mb-6">{postParams.title}</h2>
<Markdown>{post.content}</Markdown>
</section>
</>
);
};
export default BlogPost;
This will fetch all the data from our sample first-post.md file. Markdown will generate all the markdown items into pure HTML files.
Conclusion
Congrats! Blog information and blog list pages have been successfully built. Please feel free to modify and add as needed.
Want to create a High-Traffic Blog with Next.js? Contact with Staticmania. A dedicated Next.js team will guide you through the journey.