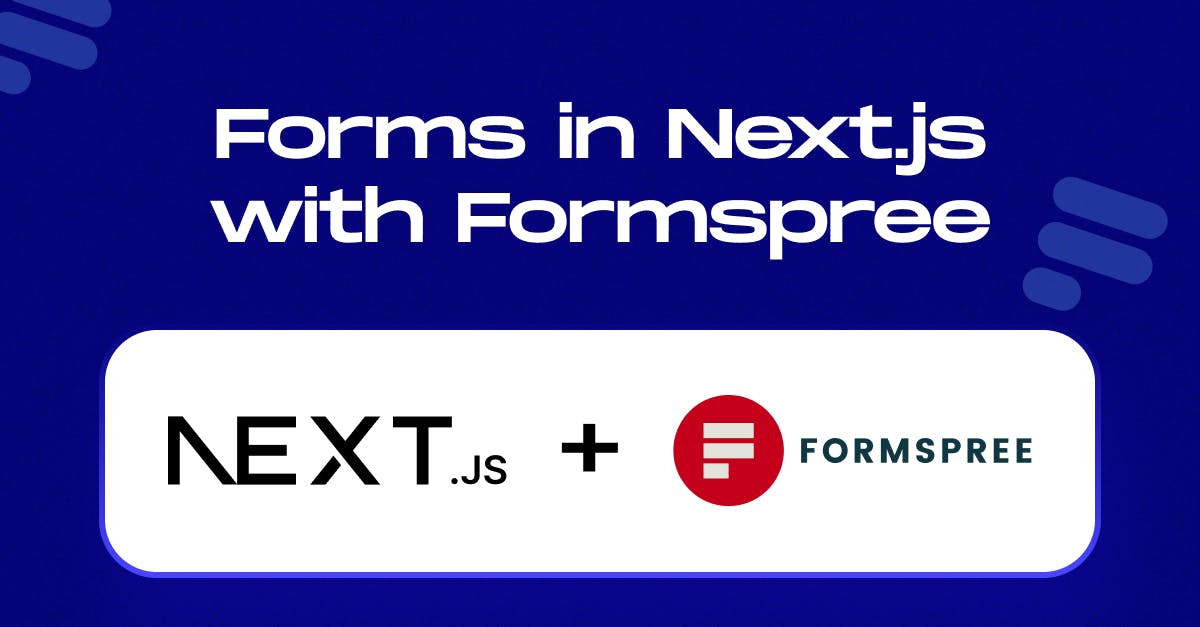
Simplifying Contact Forms in Next.js with Formspree Integration
- Author: Md. Saad
- Published at: March 03, 2024
- Updated at: March 05, 2024
Formspree is a versatile tool that simplifies form handling for developers with HTML, JavaScript, and React forms. It is a backend service, handling form processing and storage without requiring server-side code. Formspree allows you to embed custom contact, order, or email capture forms on your static website. It sends email notifications and auto-responses, offers spam protection, and integrates with third-party services. In this article, we will discuss a step-by-step guide on how to add a contact form to your Next.js website using Formspree:
Prerequisites
- You’ll need a Formspree account, which you can sign up for free here.
- Ensure you have an existing web project built with Next.js. If not, create one using:
npx create-next-app <app-name>
Creating a form endpoint
Hopefully, you have already created a Formspree account. If you don’t have one yet, do it now.
- To create a form endpoint, first, create a project. To start, create a new form with the +New project button, give it a name, select a project type, and click Create project.
- Now click the +New form button, give a Contact form name, select a project, and update the recipient email to the email where you wish to receive your form submissions. Then click Create Form.
The integration choices for your new Formspree form will then be shown to you. Keep in mind that Formspree offers you a variety of implementation samples, including ordinary HTML, Ajax, and React.
- Finally, Copy the 8-character “hashid” from the new form’s endpoint URL. It will be used later in the next js project as YOUR_FORM_ID placeholder in your contact form component.
Adding a form to Next.js
- Create a new file called contact-form.js inside of your components directory.
project-root/
├─ next.config.js
├─ components/
| └─ contact-form.js
└─ pages/
└─ index.js
- Install the helper library from Formspree, @formspree/react. It contains a useForm hook to simplify the process of handling form submission events and managing form state. Install it with:
npm install --save @formspree/react
- Add the following code snippet to the contact-form.js file:
import { useForm, ValidationError } from "@formspree/react";
export default function ContactForm() {
const [state, handleSubmit] = useForm("YOUR_FORM_ID");
if (state.succeeded) {
return <p>Thanks for your submission!</p>;
}
return (
<form onSubmit={handleSubmit}>
<label htmlFor="email">Email Address</label>
<input id="email" type="email" name="email" />
<ValidationError prefix="Email" field="email" errors={state.errors} />
<textarea id="message" name="message" />
<ValidationError prefix="Message" field="message" errors={state.errors} />
<button type="submit" disabled={state.submitting}>Submit</button>
<ValidationError errors={state.errors} />
</form>
);
}
- Replace "YOUR_FORM_ID" with your actual Formspree form endpoint.
- Import the component in your Next.js pages like so:
import ContactForm from "../components/contact-form";
- Insert the component into the page as a React component:
<ContactForm />
That’s it you have successfully added Formspree to your Nexr.js project. BUt there is something more that we can do. Such as creating a form endpoint with Vercel. Let’s discuss it.
Creating a form endpoint with Vercel
- Go to the Formspree Vercel integration page. Now click on Add Integration you’ll be asked to select your Formspree account, and then you’ll see a list of your Vercel projects that you can connect to Formspree.
- Select the projects that you want to use with Formspree. It will ask you to authorize it first. Authorize it then connect to formspree. It will take you to the connect option. Select Dashboard project and click on the connect button. Then click Done to complete the process.
- By default, the integration will create an initial form and store its ID in the NEXT_PUBLIC_FORM environment variable. To update your form code, open contact-form.js and replace the YOUR_FORM_ID placeholder with the environment variable like so:
const [state, handleSubmit] = useForm(process.env.NEXT_PUBLIC_FORM);
Now, when you fill out the form and submit it, you should see a success message. That’s it, you’re done! This guide should help you have a working React contact form in your Next.js project that sends you email notifications. The project repository is hosted on GitHub.
Want to integrate Formspree form in your Next.js project? Contact us! Our Next.js team will help you in all possible ways.