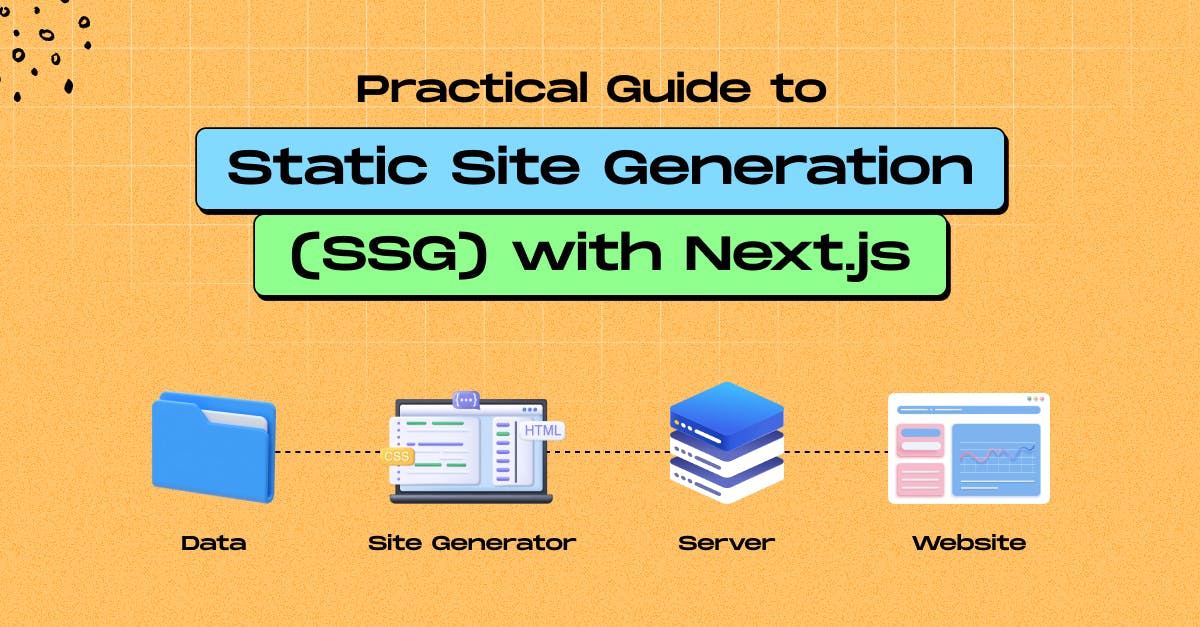
A Practical Guide to Static Site Generation (SSG) with Next.js
- Author: Md. Saad
- Published at: January 10, 2024
- Updated at: August 25, 2024
Static Site Generation (SSG) has recently become very popular in web development. Unlike dynamic websites that generate content on the server-side in response to user requests, static websites pre-generate the content during the build process. The page HTML is generated at the time of build. The result is a collection of static files that include the HTML file and its supporting components (CSS and JavaScript).
Static Site Generation (SSG) is a powerful feature in Next.js that allows you to pre-render pages at build time rather than on each request. This can significantly improve performance and reduce the load on your server. Here's a basic guide on how to use SSG in Next.js:
How Can We Use SSG in Next.js
Next.js allows you to generate pages with or without data statically. To cover this article, I assume you know how to create a Next.js. If you have not learned it already, you should first follow the link to get the basic knowledge needed to create a Next.js app. Letโs start to look into these:
SSG Without Data
If you want to create a Next.js page using Static Site Generation without fetching external data, you can follow this example:
// pages/about.js
import React from 'react';
const About = () => {
return <div>About</div>;
};
export default About;
In this example, the About component is a simple React component that renders the text "About." Since there's no need to fetch external data, this page can be pre-rendered as a static HTML file during the build process.
When you run npm run build and then npm start, Next.js will generate a static version of the about.js page, and it will serve as an HTML file, providing fast and efficient rendering for users accessing the page.
SSG with Data
Again, there are two scenarios for fetching external data for pre-rendering. These are:
๐ Page content fetches only external data. In this case, we will use only-
getStaticProps
๐ Content fetches from specific Page paths from external data. In this case, we use
getStaticPaths
in addition with
getStaticProps
Letโs discuss this in detail in these scenarios.
Page content fetches only external data
In the pages directory, create a new file for the page you want to generate statically. For example, let's create a file named blog.js. This page will fetch data from a content management system. Now copy the following code to the blog.js page.
// pages/blog.js
import React from 'react';
const Blog = ({ posts }) => {
return (
<div>
<h1>Blog Page</h1>
<ul>
{posts.map((post) => (
<li key={post.id}>{post.title}</li>
))}
</ul>
</div>
);
};
export default Blog;
Next.js offered an awesome
getStaticProps
function which is used tofetch data on pre-rendering time. This function gets called at build time and allows you to pass the fetched data to the page's props. Now use the following code to get data on the blog.js page using:
getStaticProps
In this example, the getStaticProps function is used to fetch data at build time and pass it as props to the Blog component.
Content fetches from specific Page paths from external data
In Next.js, when dealing with dynamic routes, you might encounter scenarios where the specific IDs you want to pre-render at build time depend on external data. For instance, if you've initially added only one blog post with ID 1 to the database, you'd prefer to pre-render only the path posts/1 during the build.
Later on, as you add a second post with ID 2, you'd want to pre-render the path posts/2 as well. To manage this dynamic behaviour, Next.js allows you to export an asynchronous function named getStaticPaths from a dynamic page (e.g., pages/posts/[id].js). This function is executed at build time, giving you the flexibility to specify which paths should be pre-rendered. Here's an example of creating a dynamic route for showing a single blog post based on its id:
// pages/posts/[id].js
import React from 'react';
const Post = ({ postData }) => {
return (
<div>
<h1>{postData.title}</h1>
<p>{postData.content}</p>
</div>
);
};
export const getStaticPaths = async () => {
// Fetch external data to determine dynamic paths
const response = await fetch('https://.../posts');
const posts = await response.json();
// Map data to an array of path objects with params
const paths = posts.map((post) => ({
params: { id: post.id.toString() },
}));
return {
paths,
fallback: false, // Set to true if you want to enable incremental static regeneration (ISR)
};
};
export const getStaticProps = async ({ params }) => {
// Fetch external data based on the dynamic path parameter
const response = await fetch(`https://api.example.com/posts/${params.id}`);
const postData = await response.json();
return {
props: {
postData,
},
};
};
export default Post;
In this example:
๐ The filename is [id].js, indicating that it's a dynamic route with the parameter id.
๐ The getStaticPaths function fetches external data to determine dynamic paths based on the id parameter.
๐ The getStaticProps function fetches external data for the specific id and passes it as props to the Post component.
When you run the application, you can access individual blog posts by navigating to URLs like posts/1, posts/2, etc. The corresponding blog post data will be fetched at build time and used to pre-render the pages. Remember to adjust the API URLs and data structure according to your specific use case.
Thatโs all We have successfully implemented Static Site Generation(SSG).
When Should We Use SSG?
When content changes seldom and is largely static, Next.js should be used with Static Site Generation (SSG). SSG improves performance by pre-rendering pages throughout the development process, which results in improved SEO and quicker user experiences. nextjs SSG uses CDNs to save server load and deliver consistent user experiences, making it perfect for marketing sites, blogs, or manuals. Select next js Static Site Generation (SSG) when deployment on static hosting platforms is required, offline support is needed, and real-time data or dynamic content isn't the main need.
If you're searching for top-notch Next.js developers, your ideal destination is StaticMania. We are the perfect choice for Next.js development. Don't hesitate to reach out and discuss your project with us!