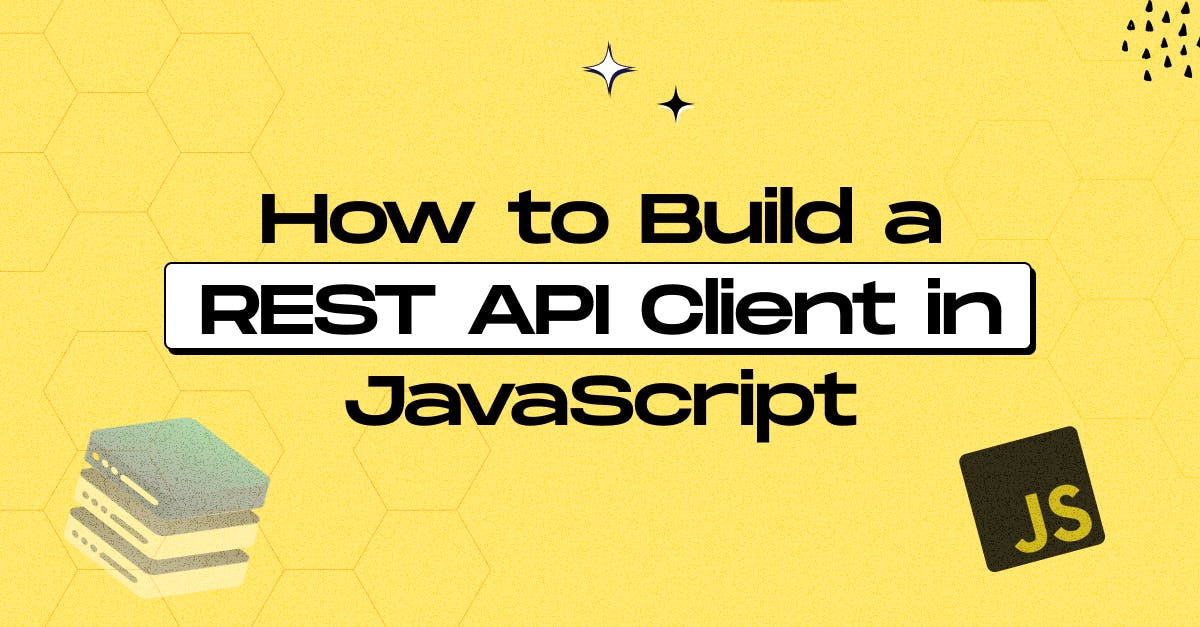
How to Build a REST API Client in JavaScript
- Author: Md. Saad
- Published at: February 16, 2025
- Updated at: February 17, 2025
Introduction
In modern web development, interacting with REST APIs is a common task. Whether fetching data from a server, submitting forms, or performing CRUD operations, JavaScript provides various ways to consume RESTful services. In this blog, we'll explore how to build a REST API client in JavaScript using the Fetch API.
Understanding REST APIs
In modern web development, interacting with REST APIs is a common task. Whether fetching data from a server, submitting forms, or performing CRUD operations, JavaScript provides various ways to consume RESTful services. In this blog, we'll explore how to build a REST API client in JavaScript using the Fetch API.
REST (Representational State Transfer) is an architectural style defining constraints for creating web services. REST APIs follow a client-server architecture, where the client sends requests to the server, and the server responds with data. These APIs use standard HTTP methods to perform operations on resources, which are typically represented as URLs.
Key Characteristics of REST APIs:
- Stateless: Each request from the client must contain all the necessary information because the server does not store any client-specific state between requests.
- Uniform Interface: REST APIs follow a standardized communication method, using HTTP methods and resource URIs.
- Resource-Based: Everything in a REST API is treated as a resource, identified by a unique URL.
- JSON or XML Response: REST APIs often return data in JSON or XML format, with JSON being the most commonly used format due to its simplicity and compatibility with JavaScript.
- Cacheable: Responses can be cached to improve performance and reduce server load.
Common HTTP Methods in REST-Based Architecture
In a RESTful API, the five most commonly used HTTP methods correspond to CRUD (Create, Read, Update, Delete) operations:
- GET (Read): Retrieves a resource. Returns 200 OK with data in JSON or XML format. If the resource is not found, it returns 404 NOT FOUND or 400 BAD REQUEST. GET is a safe and idempotent operation.
- POST (Create): Creates a new resource, typically subordinate to a parent resource. On success, returns 201 CREATED along with a Location header pointing to the newly created resource. POST is neither safe nor idempotent.
- PUT (Update/Create): Updates an existing resource or creates a new one if the client specifies the resource ID. A successful update returns 200 OK (or 204 NO CONTENT if no response body is needed). If used for creation, it returns 201 CREATED. PUT is not safe but idempotent.
- PATCH (Modify): Partially updates a resource by sending only the changed data. Unlike PUT, which replaces the entire resource, PATCH applies modifications using a structured format like JSON Patch. It is neither safe nor idempotent.
- DELETE (Delete): Removes a resource identified by a URI. On success, it returns 200 OK with a response body or 204 NO CONTENT. DELETE is not safe but idempotent.
Less Common HTTP Methods
- OPTIONS: Retrieves supported HTTP methods for a resource.
- HEAD: Similar to GET but returns only headers, without a response body.
These methods form the foundation of RESTful web services, ensuring structured and predictable interactions between clients and servers.
How to use the Fetch API
The Fetch API is a built-in JavaScript feature that provides an easy way to make HTTP requests.
Making a GET Request (Async/Await)
A GET request asks the server to return data for the specified resource without modification.
Suppose we want to get data from https://jsonplaceholder.typicode.com/posts/1, a dummy API. We can do so by sending a GET request to retrieve data and displaying it in the console. Here is an example:
async function getPost() {
try {
let response = await fetch('https://jsonplaceholder.typicode.com/posts/1');
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
let data = await response.json();
console.log('Retrieved Post:', data);
} catch (error) {
console.error('Error fetching post:', error);
}
}
getPost();
Explanation:
- We use fetch() to send a GET request to the API endpoint.
- We check if the response is successful (response.ok).
- We parse the JSON response using response.json().
- Finally, we log the retrieved post to the console.
Making a POST Request (Async/Await)
A POST request sends data to the server to create a new resource, typically adding it to a database.
Suppose we want to send data to https://jsonplaceholder.typicode.com/posts/, a dummy API, to create a new post. We can do so by creating a new post and sending the POST request to the API. Here is an example :
async function createPost() {
const postData = {
title: 'New Post',
body: 'This is the content.',
userId: 1
};
try {
let response = await fetch('https://jsonplaceholder.typicode.com/posts', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(postData)
});
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
let data = await response.json();
console.log('Post Created:', data);
} catch (error) {
console.error('Error creating post:', error);
}
}
createPost();
Explanation:
- We create an object postData with the post's title, body, and user ID.
- We send a POST request using fetch(), specifying method: 'POST'.
- We set headers to indicate we're sending JSON data.
- The request body is converted to a JSON string using JSON.stringify().
- We parse the response and log the newly created post.
Making a PUT Request (Async/Await)
A PUT request updates an existing resource entirely or creates it if it does not exist. We can use it to update an existing post by sending new data to the server. Here is a basic example:
async function updatePost() {
const updatedData = {
title: 'Updated Post',
body: 'Updated content.',
userId: 1
};
try {
let response = await fetch('https://jsonplaceholder.typicode.com/posts/1', {
method: 'PUT',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(updatedData)
});
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
let data = await response.json();
console.log('Post Updated:', data);
} catch (error) {
console.error('Error updating post:', error);
}
}
updatePost();
Explanation:
- We define updatedData with new post details.
- We send a PUT request, specifying method: 'PUT'.
- We include headers and JSON data in the request body.
- We parse the response and log the updated post.
Making a PATCH Request (Async/Await)
A PATCH request modifies part of an existing resource without affecting the entire entity. Here is an example where we are updating specific fields of an existing post.
async function patchPost() {
const patchData = {
title: 'Partially Updated Title'
};
try {
let response = await fetch('https://jsonplaceholder.typicode.com/posts/1', {
method: 'PATCH',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(patchData)
});
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
let data = await response.json();
console.log('Post Partially Updated:', data);
} catch (error) {
console.error('Error updating post:', error);
}
}
patchPost();
Explanation:
- We create an object patchData containing only the field we want to update.
- We send a PATCH request, specifying method: 'PATCH'.
- We include headers to indicate we're sending JSON data.
- The request body is converted to a JSON string.
- We parse the response and log the partially updated post.
Making a DELETE Request (Async/Await)
A DELETE request removes a specified resource from the server.
async function deletePost() {
try {
let response = await fetch('https://jsonplaceholder.typicode.com/posts/1', {
method: 'DELETE'
});
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
console.log('Post deleted successfully');
} catch (error) {
console.error('Error deleting post:', error);
}
}
deletePost();
Explanation:
- We send a DELETE request using fetch() with the method 'DELETE'.
- If successful, we log that the post was deleted.
Conclusion
Building a REST API client in JavaScript is straightforward with the Fetch API. It provides a flexible and efficient way to make HTTP requests. By mastering these techniques, you'll be well-equipped to integrate RESTful services into your applications. Happy coding!
Want more tips on Javascript , Next.js and React.js? Follow staticmania.com.
FAQ Section of How to Build a REST API Client in JavaScript
Answer: A REST API (Representational State Transfer) is a web service that allows clients to communicate with servers using standard HTTP methods like GET, POST, PUT, PATCH, and DELETE. It is stateless, meaning each request from the client must contain all necessary information, and responses are typically returned in JSON format.
Answer: To make a GET request using the Fetch API, call fetch(url), check for a successful response, and parse the JSON data using response.json(). This allows you to retrieve and use data from an API in your application.
Answer: PUT replaces the entire resource with new data, while PATCH updates only the specified fields. PUT is used when you want to overwrite an existing record, whereas PATCH is used for partial updates to an existing resource.
Answer: To send a POST request, use fetch(url, { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify(data) }). This sends the data as JSON to the server, typically creating a new resource.
Answer: Always check the response.ok before parsing JSON, use try...catch to handle network errors or invalid responses. This ensures your app gracefully manages failed API calls.
Answer: To delete a resource, send a DELETE request using fetch(url, { method: 'DELETE' }). If successful, the API usually returns a 204 No Content status, indicating the resource was removed.