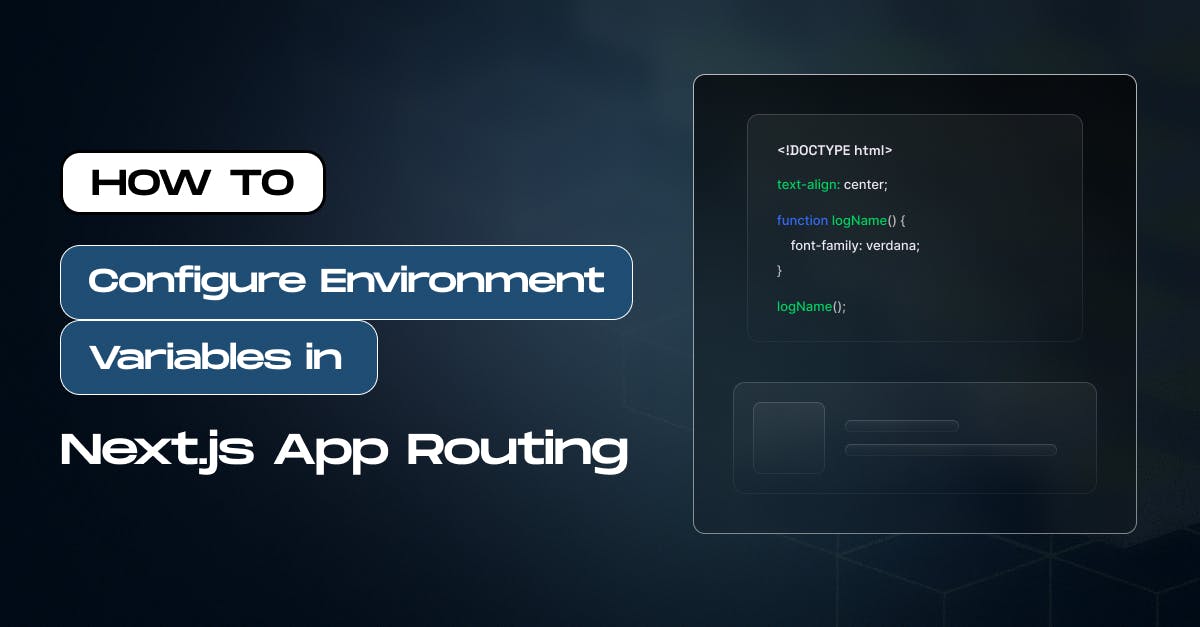
How to Configure Environment Variables in Next.js App Routing
- Author: Md. Saad
- Published at: July 14, 2024
- Updated at: August 03, 2024
Environment variables play a crucial role in configuring Next.js applications. Whether you’re handling API keys, authentication tokens, or other sensitive information, understanding Next.js environment variables is essential for a secure and efficient application. In this article, we’ll explore how to set up and manage environment variables specifically for app routing within your Next.js project. Let’s dive in!
What are Environment Variables?
A .env file is a simple text file used to store environment variables for a project. They are defined in a key and value pair. These variables typically contain configuration settings such as database connection strings, API keys, port numbers, and other sensitive or environment-specific information. The .env file is commonly used in software development to manage these variables separately from the application's source code, making it easier to maintain and secure configurations across different environments (e.g., development, testing, production). The following are valid examples of environment variables:
API_KEY='f9E58Q37&frs_wklmn$'
API_KEY= f9E58Q37&frs_wklmn$$
ACCESS_TOKEN='f9E58Q37&frs_wklmn$’
Loading Environment Variables
Next.js manages environment variables by default. Next.js made it much easier to integrate support for environment variables. That means using environment variables in your applications is now easy and smooth. Here’s how to work with environment variables in a Next.js project:
Setting Up Environment Variables
Create a .env file at the project's root. In this file, all the environment variables will be saved. Later, we can access these variables using process.env. For example, add a .env.local file in your project root directory with the following content:
DB_HOST=localhost
DB_USER=myuser
DB_PASS=mypassword
Next.js will automatically load the variables as process.env.DB_HOST, process.env.DB_USER and process.env.DB_PASS which we can then use as shown below:
export async function GET() {
const db = await myDB.connect({
host: process.env.DB_HOST,
username: process.env.DB_USER,
password: process.env.DB_PASS,
})
// ...
}
Furthermore, A dollar ($) sign can also refer to other variables in the same env.local file.
//.env.local file
TWITTER_USER=nextjs
TWITTER_URL=https://twitter.com/$TWITTER_USER
Good to know: .env, .env.development, and .env.production files should be included in your repository as they define defaults. .env*.local should be added to .gitignore, as those files are intended to be ignored. .env.local is where secrets can be stored.
Integrating Browser-Specific Environment Variables
By default, environment variables are only available on the server. But Next.js gives us a way to let the browser see the variables. Prefix the variable name with NEXT_PUBLIC_. These variables will be exposed to the browser and can be accessed on both the client and server sides. For example:
# env.local
NEXT_PUBLIC_GOOGLE_ANALYTICS_ID='your_google_analytics_id_here'
Now, we can use it our code like the following:
export default function Home() {
return (
<div>
<h1>API URL: {process.env.NEXT_PUBLIC_GOOGLE_ANALYTICS_ID}</h1>
</div>
);
}
Supported Environment Variables
IN General, .env.local file is enough for our tasks. However, we might need to add other environment variables files for the development or production environment. Great news is that Next.js supports multiple environment files:
- .env – for all environments
- .env.local – for local development. .env.local always overrides the default set.
- .env.development – for development environment
- .env.production – for production environment
- .env.test – for test environment
Environment Variable Load Order
Environment variables are looked up in the following places, in order, to stop once the variable is found.
- process.env
- .env.$(NODE_ENV).local
- .env.local (Not checked when NODE_ENV is test.)
- .env.$(NODE_ENV)
- .env
For example, if NODE_ENV is development and you define a variable in both .env.development.local and .env, the value in .env.development.local will be used.
Environment Variables on Vercel
When deploying your Next.js application to Vercel, Environment Variables can be configured in the Project Settings. All types of environmental variables should be configured there. For, more info on configuring environment files visit the varcel official page.
Note: If environment variable names are not prefaced with NEXT_PUBLIC_, .env* file will not be available to Edge Runtime. So. it is strongly recommended to manage environment variables in Project Settings, from where all environment variables are available.
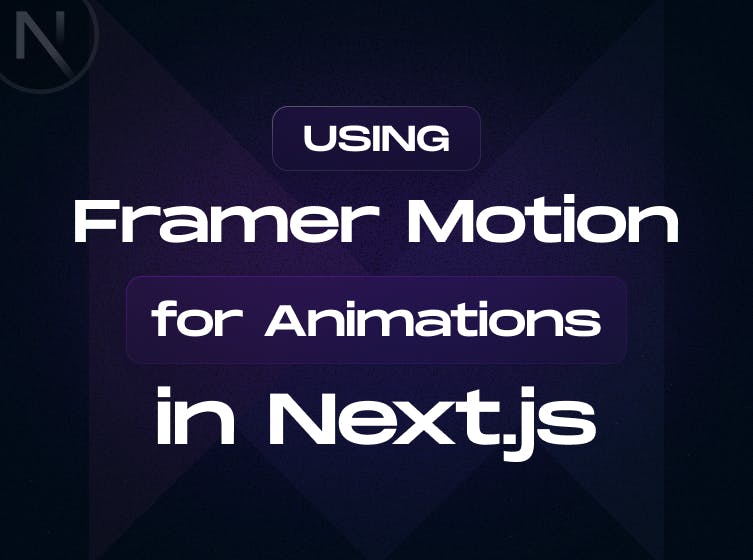
How to Use Framer Motion for Animations in Next.js
Framer Motion is a popular motion library for React. With Framer Motion, developers can easily build animations and transitions using React and little to no code. It's a production-ready tool that can create complex animations and interactions.
Read articleEnvironment Variables on Vercel
That’s All. You have learned about how to configure Next.js environment variables in app routing. This should help you manage environment variables effectively in a Next.js project. If you have any specific questions or issues, please contact us for any queries!