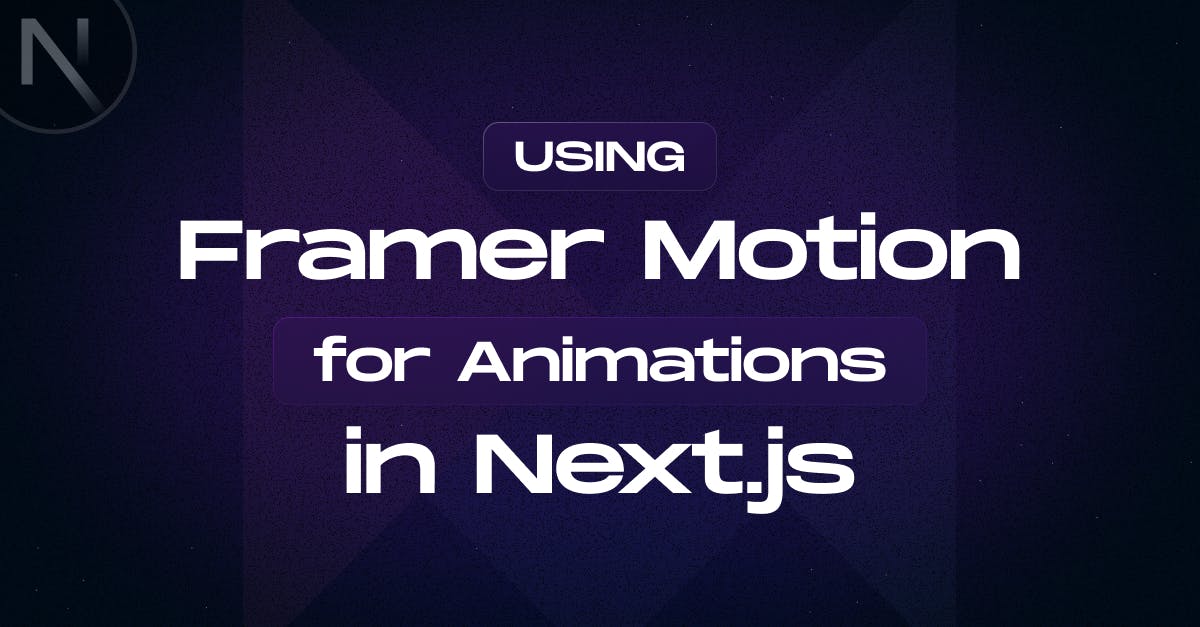
How to Use Framer Motion for Animations in Next.js
- Author: Md. Saad
- Published at: August 03, 2024
- Updated at: August 03, 2024
Framer Motion is a popular motion library for React. With Framer Motion, developers can easily build animations and transitions using React and little to no code. It's a production-ready tool that can create complex animations and interactions. As Next.js is a React-based web application with server-side rendering and static website generation, Framer motion animation works perfectly with it. Furthermore, integrating Framer Motion in Next.js with the App Router enables smooth, customizable animations, enhancing user experience with seamless transitions and dynamic visuals for a polished, interactive application. Today we will learn How to Use Framer Motion for Animations in Next.js in a simple way
Prerequisites
To follow along with this guide and code, you should have the following:
- Basic understanding of HTML, CSS, and JavaScript
- At least a little experience or knowledge of Next.js.
- Node and npm or yarn installed on your local computer
Setting up a Next.js Application
The first and foremost step to begin with is creating a Next.js App. Use the following command to create a next.js app.
npx create-next-app@latest app-name
As it is not an article on creating the next.js app, we will not discuss it in detail. If you want to read it in detail, follow the link
Install Framer Motion
As your next.js project is successfully created, it’s time to add Framer Motion. Install Framer Motion using yarn or npm:
yarn add framer-motion
# or
npm install framer-motion
After installation, use the framer-motion command to import Framer Motion.
import { motion } from "framer-motion"
Basic Example
First of all, we should remember that framer motion only works in client components. Let’s code a basic Framer Motion.
go to the app/page.js file.
"use client";
import {motion} from "framer-motion";
export default function Home() {
return (
<div>
<h1>Welcome to the Home Page</h1>
</div>
);
}
At first, add “use client” to make the page a client page. Then import framer motion using
import {motion} from "framer-motion";
Now change the div with motion.div
Now add the following codes in the motion.div
initial={{opacity: 0, y: 100}}
animate={{
opacity: 1,
y: 0,
}}
transition={{duration: 0.5}}
Here initial, animate and transition values will control the framer motion animation prop can accept an object value like the example. If we change one of them, the motion animation will automatically change.
So, Finally, our code should be like this:
"use client";
import {motion} from "framer-motion";
export default function Home() {
return (
<motion.div
initial={{opacity: 0, y: 100}}
animate={{
opacity: 1,
y: 0,
transition:{duration:0.5}
}}
>
<div>
<h1>Welcome to the Home Page</h1>
</div>
</motion.div>
);
}
How to Optimize Framer Motion animation with Variants
In Framer Motion, variants are a powerful feature used to define multiple animation states and transitions for a component in a reusable and organized way. Instead of specifying animations directly in the component's props, you can define a set of named states (variants) and then apply those states to the component. Let’s optimize our code with variants:
Create a variant component. Let’s name it fadeUpVariant. Inside it add initial and animate objects. In the initial object, the initial state of the item will be added. In animate object, we will add the animation of the item.
const fadeUpVariant = {
initial: { opacity: 0, y: 100 },
animate: {
opacity: 1,
y: 0,
transition: {
duration: 0.5,
},
},
}
Now, add variants prop in the motion.div. Also, update other props. variants will take the name of variant components within curly braces. Initial and animate values will take the names of the objects inside the variants.
After updating the variant all the codes should be like the following.
"use client";
import {motion} from "framer-motion";
const fadeUpVariant = {
initial: {opacity: 0, y: 100},
animate: {
opacity: 1,
y: 0,
transition: {
duration: 0.5,
},
},
};
export default function Home() {
return (
<motion.div
variants={fadeUpVariant}
initial="initial"
animate="animate"
>
<h1>Welcome to the Home Page</h1>
</motion.div>
);
}
}
Add Exit animations
The exit prop defines the animation that plays when the component is removed from the DOM. Usually, it is very difficult to animate When an item exists. But Framer motion introduced an exit property that makes our work easy. To use exit animations in Framer Motion, we need to wrap our component with the AnimatePresence component, which enables exit animations. Let’s create another example of it:
First thing first, Import AnimatePresence from framer motion.
import {motion, AnimatePresence} from "framer-motion";
Let’s create another variant and name it exitAnimation. Now add hidden, visible and exit objects. Initially, the item will be hidden and later it will be visible after animate. I have used a new object Exit. This will create an animation when the item disappears.
export const exitAnimation = {
hidden: {opacity: 0, x: -100},
visible: {opacity: 1, x: 0},
exit: {opacity: 0, x: 100},
};
Now, create a toggle button. Then we will create the toggle item and wrap it with. let’s code it:
<motion.button
variants={fadeUpVariant}
initial="initial"
animate="animate"
onClick={() => setIsVisible(!isVisible)}
>
Toggle Component
</motion.button>
{isVisible && (
<motion.div
key="box"
initial="hidden"
animate="visible"
exit="exit"
variants={exitAnimation}
>
<h1>Hello, Framer Motion!</h1>
</motion.div>
)}
</AnimatePresence>
In this example:
- The motion.div component uses the initial, animate, and exit props to define its animation states.
- The exit prop uses the exit variant to define the animation that should play when the component is removed.
Finally, wrap the whole code with AnimatePresence to active exit animation.
So, the final code will be the following:
"use client";
import {motion, AnimatePresence} from "framer-motion";
import {useState} from "react";
export const fadeUpVariant = {
initial: {opacity: 0, y: 100},
animate: {
opacity: 1,
y: 0,
transition: {
duration: 0.5,
},
},
};
export const exitAnimation = {
hidden: {opacity: 0, x: -100},
visible: {opacity: 1, x: 0},
exit: {opacity: 0, x: 100},
};
export default function Home() {
const [isVisible, setIsVisible] = useState(false);
return (
<AnimatePresence>
<motion.div
variants={fadeUpVariant}
initial="initial"
animate="animate"
>
<h1>Welcome to the Home Page</h1>
</motion.div>
<motion.button
variants={fadeUpVariant}
initial="initial"
animate="animate"
onClick={() => setIsVisible(!isVisible)}
>
Toggle Component
</motion.button>
{isVisible && (
<motion.div
key="box"
initial="hidden"
animate="visible"
exit="exit"
variants={exitAnimation}
>
<h1>Hello, Framer Motion!</h1>
</motion.div>
)}
</AnimatePresence>
);
}
How to Use Framer Motion in a Server Components
As Framer motion works only in client Components, it requires a slightly different approach to use in Server Components. Since server components cannot have client-side interactivity directly, we can use client components for the parts that need animations. Let’s discuss:
Create a Client Component and define your animation in the client component. Client components handle client-side behaviour, such as animations. Let’s create an AnimatedComponent.jsx file. Now create a variant and other animation like before. I have added a sample below:
// components/AnimatedComponent.jsx
'use client'
import { motion } from 'framer-motion'
export const fadeUpVariant = {
initial: { opacity: 0, y: 100 },
animate: {
opacity: 1,
y: 0,
transition: {
duration: 0.5,
},
},
}
const FadeUpAnimation = ({ children}) => {
return (
<motion.div variants={fadeUpVariant} initial="initial" animate="animate">
{children}
</motion.div>
)
}
export default FadeUpAnimation
At this stage, we have to use the Framer Motion Client Components into the Server Component. Let’s do this now.
- Go to the app/page.js file. Remove all the codes and add your code.
- Import FadeUpAnimation components.
- Wrap the codes with <FadeUpAnimation> </FadeUpAnimation>
Your final code in the app/page.js should be:
import FadeUpAnimation from "@/components/FadeUpAnimation";
export default function Home() {
return (
<FadeUpAnimation>
<h1>Welcome to the Home Page</h1>
</FadeUpAnimation>
);
}
By following these steps, we can integrate Framer Motion into our Next.js application using server components and client components together. This approach allows us to leverage the benefits of server-side rendering while still adding client-side animations and interactions.
At this moment, it is time to check whether it works or not. Let’s run the site using the command
npm run dev
or
Yarn dev
That’s all. It should open the site's localhost:3000 port on the browser. Also, a nice transition should be seen after loading. You can further customize the animations by changing the framer motion properties, such as variants, animate and others.
Visit the official Framer Motion website to learn more about it. There are some incredible and far more sophisticated options out there that can enhance your user experience.
To make your website smooth and eye-catching that enhance the user experience, contact us. We will guide you on the journey.