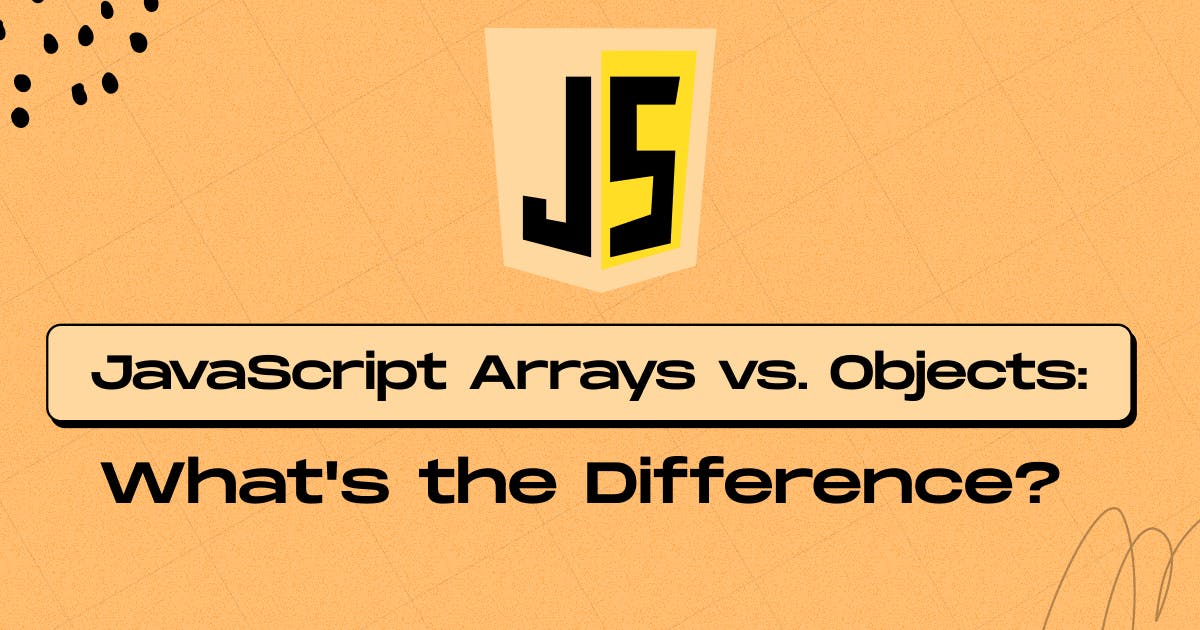
JavaScript Arrays vs. Objects: What's the Difference?
- Author: Md. Saad
- Published at: December 01, 2024
- Updated at: December 01, 2024
Arrays and objects in JavaScript are unique and highly versatile data structures. Arrays and objects are both used to store collections of data, but they serve different purposes and are structured differently. In this article, we will discuss the comparison between arrays and objects to help clarify their distinctions:
What are JavaScript Arrays?
In JavaScript, an array is a special object designed to store ordered collections of values. Arrays are ideal for handling sequential and ordered lists of data.
An array is declared using square brackets []. Then you can access elements in an array using their index (starting from 0), inside square brackets [].
Example of an array:
const fruits = ["apple", "banana", "cherry"];
console.log(fruits[0]); // Output: apple
Key characteristics of arrays:
- Indexed by numbers: Array elements are accessed using numeric indices starting from 0.
- Ordered: Arrays maintain the order of elements, meaning that they are inherently sequenced.
- Flexible Length: Arrays are dynamically sized, so you can add or remove elements without specifying a fixed length.
- Built-in Methods: JavaScript provides various built-in methods to work with arrays, such as push, pop, map, filter, reduce, and more.
What are JavaScript objects?
An object in JavaScript is a data structure that stores data in key-value pairs. Unlike arrays, objects are not ordered, which means the data doesn't follow a specific sequence. Objects are ideal when working with data with a unique identifier.
An object is declared using curly braces {}. Then initialise it with key-value pairs. Finally, you can access values with dot notation followed by the key name (e.g., object.key) or square bracket notation followed by a key name (e.g., object["key"]).
Example of an Object:
const person = {
name: "John",
age: 30,
city: "New York"
};
console.log(person.name); // Output: John
// or
console.log(person[“name”]); // Output: John
Key characteristics of objects:
- Key-Value Pairs: Each element is stored as a key-value pair.
- Unordered Data: This does not preserve the order of elements.
- Flexible Structure: Keys can represent different types of information.
- Dot and Bracket Notation: You can access values with dot notation or bracket notation.
When to Use Arrays vs. Objects
Use Arrays When:
- You need to store multiple items in a specific order.
- You need fast access to elements by their position in a list.
- You want to use array-specific methods for manipulation, such as sort(), map(), filter(), and reduce().
Use Objects When:
- You need to store data as properties of an entity.
- You want to use descriptive keys to identify each piece of data.
- You are working with non-ordered data that doesn’t rely on a specific position.
Conclusion
Arrays and objects are two powerful JavaScript data structures, each with its own set of benefits. Arrays are great for working with ordered lists, but objects are best for organised tagged data. Understanding the distinctions and when to use them will help you write cleaner, more efficient code. With such basic information, you'll be more prepared to choose the best selection for the requirements of your project.
Dive deeper into JavaScript arrays and objects and unlock their full potential! If you have any questions or need guidance, StaticMania is here to help. Happy coding!