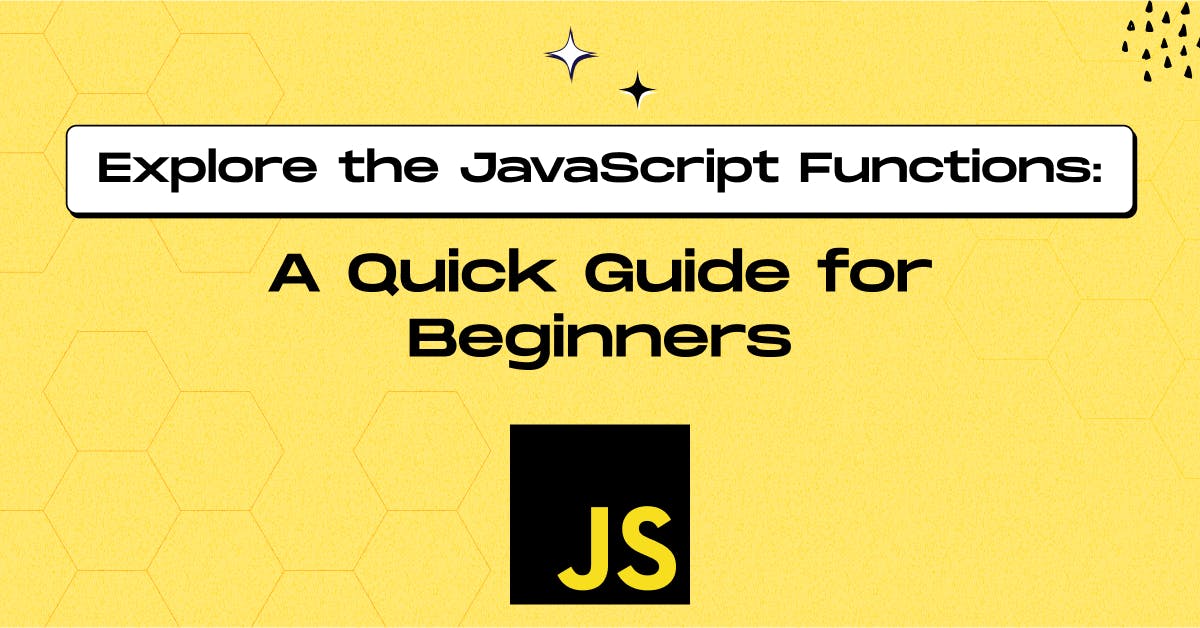
Explore the JavaScript Functions: A Quick Guide for Beginners
- Author: Md. Saad
- Published at: November 24, 2024
- Updated at: November 24, 2024
Introduction
Functions are an essential language component in JavaScript, allowing us to bundle and reuse code, build dynamic web applications, and handle sophisticated functionality with a few lines. Understanding functions is essential whether you're new to JavaScript or looking to improve your skills. Let's review the fundamentals and syntax of JavaScript functions to help you get started!
What is a function in JavaScript?
A function is a reusable part of code that completes a certain task. Instead of writing the same code again, you can define it once in a function and then call that function whenever you need it. Functions help to keep your code organised, readable, and efficient.
In JavaScript, functions can be created in multiple ways, each with unique syntax. Once you define a function, you can "call" it (or "invoke" it) to run the code inside.
How to Declare a Function?
- In JavaScript, a function is defined using the function keyword, followed by a name and parentheses ().
- Function names can include letters, digits, underscores, and dollar signs (same rules as variable names).
- The parentheses can contain parameter names, separated by commas: (parameter1, parameter2, ...)
- Parameters are specified within the parentheses in the function definition.
- Arguments are the values passed to the function when it is called.
- The arguments (or parameters) act as local variables within the function.
The code that the function executes is enclosed within curly brackets {}:
function functionName (parameters) {
// code to be executed
}
functionName()
In this example:
- Function: This keyword tells JavaScript that you are defining a function.
- functionName: This is the name you give to your function. It should describe the action the function performs.
- Parameters: These are the inputs to the function. You can have multiple parameters separated by commas. Parameters act as placeholders that get filled with actual values (arguments) when you call the function.
- Code block: This is where you put the code that will run when the function is called.
Example:
function functionName (name) {
console.log("Hello, " + name + "!");
}
Here, I have created the functionName function. This function takes one parameter (name) and logs a message to the console. You would call this function like so:
functionName("Alice"); // Output: Hello, Alice!
Function Executions
Function call, execute, or invoke—whatever you say is very processed. To call a function, you have to write the function with a parenthesis (). Accessing a function without () returns a string version of the entire function's definition, not the result. Example of JavaScript Functions execution with parenthesis:
Example:
function functionName (name) {
console.log("Hello, " + name + "!");
}
functionName("Alice");
// Output: Hello, Alice!
An example of function execution without parenthesis will output the following result:
An example of function execution without parenthesis will output the following result:
function functionName (name) {
console.log("Hello, " + name + "!");
}
functionName;
// Output:
function functionName (name) {
console.log("Hello, " + name + "!");
}
Functions Used as Variable Values
In addition to JavaScript function declarations, JavaScript allows you to define functions as expressions. That means we can define a function like a variable.
Example:
const functionName = function(name) {
console.log("Hello, " + name + "!");
};
functionName ("Bob"); // Output: Hello, Bob!
This example has just defined the function using a function expression. This means that the name used for functions before is now a variable. So, the functionName variable is nothing but a function now. Now, we can execute the variable like a normal function using parenthesis.
Function Parameters and Default Values
JavaScript allows you to set default values for parameters if they aren’t provided during a function call. This feature, added in ES6, can help prevent errors when arguments are missing.
function testFunction(name = "Guest") {
console.log("Hello, " + name + "!");
}
testFunction(); // Output: Hello, Guest!
testFunction("Alice"); // Output: Hello, Alice!
Here, if the name is not provided in the function call, it will return the default value, which is "Guest".
Rest Parameters
The rest of the parameters in JavaScript allow functions to accept an indefinite number of arguments as an array, making them useful for handling a variable number of arguments in a clean, readable way.
The syntax for using rest parameters is simple. You define them by using three dots ... followed by the parameter name:
We must remember two things while defining rest parameters in a function.
- A function definition can have only one rest parameter.
- The rest parameter must be the last in a function.
You can mix regular parameters with the rest parameters. Only the arguments that don’t match regular parameters are collected into the rest parameter array.
Example:
Imagine you have a function to calculate the sum of an unknown number of numbers. Rest parameters make this easy:
function createMessage(sender, ...messages) {
messages.forEach(message => console.log(`[${sender}]: ${message}`));
}
createMessage("Admin", "Welcome!", "Please read the guidelines.");
// Output:
// [Admin]: Welcome!
// [Admin]: Please read the guidelines.
Returning Values
In the web application, there will be multiple functions. In many cases, you may need to use the output value of a function in another one. You can do this using the return keyword. It means everything that you do inside a function, all the tasks, all the logic, all the operations, and at the end of it, if you want the function to return a value so that that value can be utilised elsewhere, you have to use a return statement, followed by what you want to return. Here is an example of a return:
function add(a, b) {
return a + b;
}
const sum = add(5, 7);
console.log(sum); // Output: 12
In this example, there is a function add. This function will do a summation addition of two things. So it takes a and b as two parameters. Then, it sums up those two parameters with this arithmetic operation a plus b and returns the result. Finally, execute the function and assign the values 5, and 7. It stores the value in a variable sum and later prints the value using console.log().
When a function encounters a return statement, it immediately stops executing and returns the specified value. If a function does not return anything, it will return undefined automatically.
Arrow Functions
Arrow Functions are introduced in ES6. They provide a more simple syntax pattern for writing functions. They are very useful when working with callbacks or short functions. You don't need any function keyword at all to make an arrow function. You need an arrow-like syntax using a combination of the equals and the greater than key without any space side by side (=>). And this is what makes an arrow function.
const functionName = (parameters) => {
// Code to be executed
};
If your arrow function has only one parameter and a single line of code, you can omit the parentheses around the parameter and the curly braces around the code:
const testFunction= name => console.log("Hello, " + name + "!");
testFunction("Charlie"); // Output: Hello, Charlie!
You can also add multiple parameters in an arrow function. Example of Arrow Function with Multiple Parameters:
const add = (a, b) => a + b;
console.log(add(3, 4)); // Output: 7
Note: Arrow functions differ from regular functions in handling this context, which can be beneficial or limiting depending on your needs.
Nested Function
Javascript allows the creation of a function within another function. In JavaScript, nested functions are functions defined within other functions. The nested function must be called inside the outer function itself.
Example of nested function:
function outerFunction() {
console.log("outerValue")
const innerFunction = () => {
console.log("innerValue");
};
innerFunction();
}
outerFunction();
// First Output: outerValue
// Second Output: innerValue
When you call the outer function, it will be invoked and print its console.log. Then it will see that an inner function has been defined. And so it will invoke the inner function also. So the output is the outer and then the inner function.
Function Scope
In JavaScript, function scope determines the accessibility (or visibility) of variables declared within a function. Variables declared inside a function are only accessible within that function and are not visible outside of it. Moreover, a function can access all the variables inside the scope it is defined.
function globalScope() {
let message = "Hello!";
console.log(message); // Output: Hello!
}
globalScope();
console.log(message); // Error: message is not defined
So, you can see that the variable that is defined inside this function cannot be accessed from anywhere outside of the function.
let globalVar = "I'm global";
function localFunction() {
let localVar = "I'm local";
console.log(globalVar); // Output: I'm global
console.log(localVar); // Output: I'm local
}
localFunction();
But, in this example, you can access the globalVar inside the function.
This method will also be applicable in the nested function. That means the inner function will be able to access anything that is defined in this outer function, and also the outer function cannot access anything from the inner function because the inner function is defined in the outer function scope.
Closures
A closure in JavaScript is a powerful feature that allows an inner function to access variables and parameters from its outer (enclosing) function even after that outer function has finished executing. Closures are created whenever a function is defined inside another function and gains access to the outer function's scope. Example of Closure:
function outerFunction() {
let outerVariable = "I'm an outer variable";
function innerFunction() {
console.log(outerVariable);
}
return innerFunction;
}
const closureFunction = outerFunction();
closureFunction(); // Output: I'm an outer variable
In this example:
- outerFunction defines a variable outerVariable and a nested function innerFunction.
- innerFunction references outerVariable, creating a closure.
- closureFunctionis assigned the result of outerFunction(which is innerFunctionitself) and retains access to outerVariableeven after outerFunctionhas been completed.
Callback Function
A callback function is a function passed into another function as an argument.
function greetingMessage(name, callback) {
console.log('Hi' + ' ' + name);
callback();
}
// callback function
function callBackFunction() {
console.log('I am callback function');
}
// passing function as an argument
greetingMessage('Peter', callBackFunction);
In this example:
- greetingMessage is a function that takes a parameter named callback.
- This callback parameter value can be anything. But in our case, it will be a function.
- callBackFunction is a function that is passed as the arguments of the greetingMessage function.
- This callBackFunction() is called a callback function.
Anonymous Functions
Anonymous functions are functions without a name. They are often used as arguments to other functions, like in event handlers or immediately-invoked function expressions (IIFE).
button.addEventListener("click", function() {
alert("Button was clicked!");
});
In this case, the function doesn’t have a name, and it only runs when the button is clicked.
Higher Order Function
Higher-order functions are functions that operate on other functions, either by taking them as arguments or by returning them. So, a higher-order function is a function that does one of the following:
- Takes one or more functions as arguments, or
- Returns a function as its result.
Some higher-order functions are map, filter, reduce, etc.
Here is an example of a higher-order function that takes functions as arguments:
// Callback function passed as a parameter in the higher-order function
function callbackFunction(){
console.log('I am a callback function');
}
// higher-order function
function higherOrderFunction(func){
console.log('I am higher order function')
func()
}
higherOrderFunction(callbackFunction);
This is a higher-order function because a callbackFunction is a function and it is executed inside higherOrderFunction. Later, a higherOrderFunction function is called with a function as an argument.
Here is an example of a higher-order function that returns functions:
function testHOFFunction(test){
return function(){
console.log("Higher-order function that returns Functions")
}
};
const fn = testHOFFunction()
fn()
//output: higher-order function that returns Functions
Here, testHOFFunction is a higher-order function because it returns a new function fn().
Pure Function
A pure function in JavaScript (and functional programming in general) is a function that has two essential characteristics:
- Same input, same output: Pure functions rely only on their input parameters and consistently produce the same output for the same inputs.
- No Side Effects: Pure functions do not affect external states or data. They do not change variables outside their scope, modify input arguments (immutability), or perform actions like logging, HTTP requests, or altering the DOM.
function add(a, b) {
return a + b;
}
console.log(add(2, 3)); // Output: 5
console.log(add(2, 3)); // Output: 5 (Always the same output for same inputs.)
In this example:
- Add takes two numbers and returns their sum.
- It doesn’t rely on or modify any external variables, nor does it produce any side effects. It will always return 5 when given 2 and 3.
Impure Function
An impure function is a function that has side effects or does not always return the same output when given the same input
Here's an example of an impure function that modifies an external variable:
let counter = 0;
function incrementCounter() {
counter += 1;
return counter;
}
console.log(incrementCounter()); // Output: 1
console.log(incrementCounter()); // Output: 2
In this example:
- incrementCounter is impure because it modifies the counter variable, which exists outside its scope.
- Calling incrementCounterrepeatedly produces different results, making it non-deterministic.
Immediately Invoked Function Expressions (IIFE)
An IIFE is a function that runs as soon as it’s defined. This is useful when you want to execute code only once and avoid polluting the global scope.
(function() {
console.log("This function runs immediately!");
})();
So we started with an anonymous function, then we used a group operator around that so that we get a function definition, and then the last parenthesis is a pair of parenthesis used always to call or invoke the function.
Recursion
Recursion means a function that refers to itself.
- Base Case: This is the condition that stops the recursion. Without it, the function would call itself infinitely, leading to a stack overflow.
- Recursive Case: The part of the function that includes the recursive call. This typically involves modifying the input or reducing the problem size in some way to eventually reach the base case.
Here's how we would implement this in JavaScript:
function factorial(n) {
if (n === 0 || n === 1) { // Base case
return 1;
}
return n * factorial(n - 1); // Recursive case
}
console.log(factorial(5)); // Output: 120
In this example:
- The base case is when n is 0 or 1, where the function returns 1 (since 0! and 1! both equal 1).
- The recursive case is n * factorial(n - 1), which calls factorial with a decremented value of n.
- When factorial(5) is called, it leads to a series of nested calls:
- factorial(5) calls factorial(4).
- factorial(4) calls factorial(3).
- factorial(3) calls factorial(2).
- factorial(2) calls factorial(1).
- factorial(1)reaches the base case and returns1.
Conclusion
Functions are the backbone of JavaScript. I hope you enjoyed learning all about JavaScript functions. Some of them are at a very high level. Some are in-depth. However, the purpose of the article was to go over all of the aspects of JavaScript functions and give you confidence that you can understand some of these concepts if you study gradually and connect the dots.
Keep exploring and enhancing your knowledge of JavaScript functions! Please do not hesitate to contact StaticMania with any questions or feedback. Happy coding!