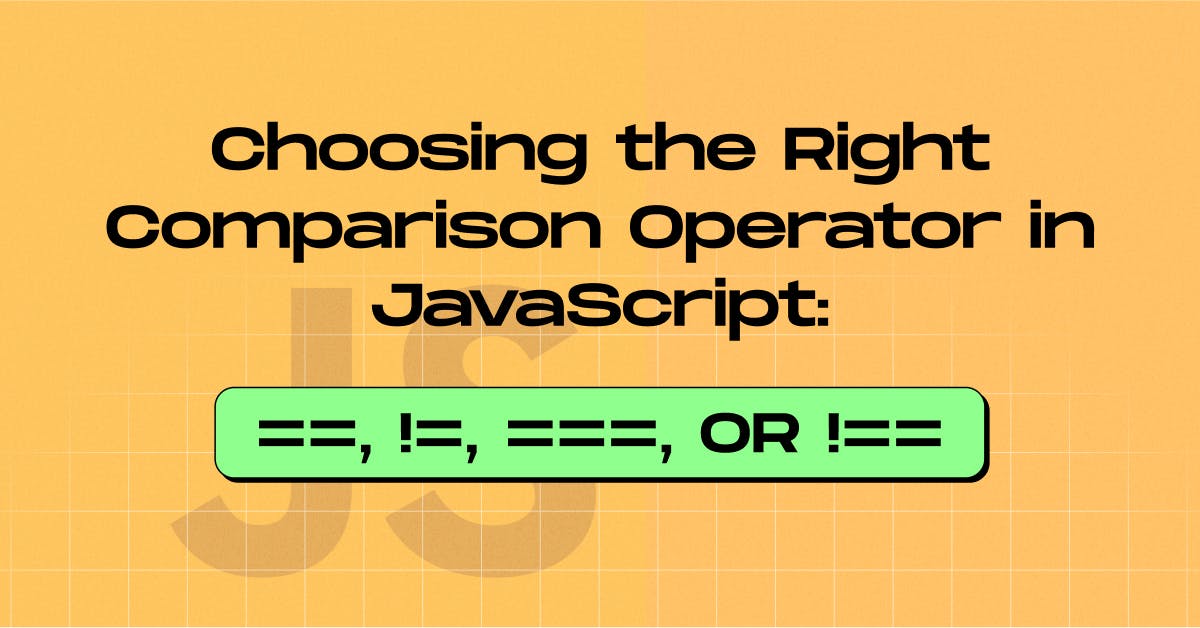
How JavaScript Comparison Operators Really Work: ==, ===, !=, !==
- Author: Md. Saad
- Published at: December 08, 2024
- Updated at: April 10, 2025
Comparison operators are a core part of JavaScript. They evaluate two values and return a boolean (true or false) based on whether the condition is met. These operators are crucial for writing if statements, loops, and other logic where decisions are made based on how values relate to each other.
In JavaScript, comparison operators also check value and reference equality, especially with arrays and objects. ==, !=, ===, and !== are some of the most commonly used—and often misunderstood—operators.
Grasping how these equality operators work helps prevent subtle bugs and makes your code more predictable. This post’ll explain how each function works and when to use them in your JavaScript projects.
Equality Operator (==)
The Equality Operator (==) operator, also known as the loose equality operator, checks for equality between two values without considering data types. If equal, the condition is true; if not, it is false. It performs type coercion before the comparison, making it less strict.
Example:
console.log(5 == '5'); // true
console.log(0 == false); // true
console.log(null == undefined); // true
Explanation:
- 5 == '5': JavaScript converts '5' (a string) to 5 (a number) before comparing, resulting in true.
- 0 == false: Here, false is converted to 0, so the comparison becomes 0 == 0, which is true.
- null == undefined: These are considered loosely equal, so this comparison returns true.
When to Use ==
The == operator can be useful when comparing values without worrying about the data type. However, be cautious, as it can lead to unexpected results due to implicit type conversion.
Inequality Operator (!=)
The inequality operator (!=), or the loose inequality operator, checks if two values are not equal. If the values are equal, then the condition returns false; otherwise, it returns true. Like the equality operator (==) operator, it also performs data type coercion before the comparison, making it less strict.
Example:
console.log(5 != '5'); // false
console.log(0 != false); // false
console.log(5 != 10); // true
Explanation:
- 5 != '5': After type coercion, 5 is equal to 5, so this returns false.
- 0 != false: After coercion, both are equal, so this returns false.
- 5 != 10: The values are different, so this returns true.
When to Use !=
Similar to ==, != can lead to unexpected results due to type coercion. For most cases, it’s safer to use the strict inequality operator (!==) to avoid unintentional type conversions.
Strict Equality Operator (===)
The Strict Equality Operator (===) operator checks whether two values and data types are equal or not. If the values have different data types, the values are considered unequal. Only if both the value and data type of the operands are the same, then the comparison will return true.
Example:
console.log(5 === '5'); // false
console.log(0 === false); // false
console.log(null === undefined); // false
console.log(5 === 5); // true
Explanation:
- 5 === '5': Here, the types are different (number vs. string), so the result is false.
- 0 === false: 0 is a number, and false is a boolean, so this also returns false.
- null === undefined: These are considered equal in implicit data type comparison (==), but not with strict equality (===), so the result is false.
- 5 === 5: Both the type and value are the same, so the result is true
When to Use ===
The === operator is generally preferred in most JavaScript codebases because it avoids the unpredictability of implicit type conversion. By using ===, it ensures that the value and data types are compared, leading to more predictable and accurate code.
Strict Inequality Operator (!==)
The strict inequality operator (!==) checks whether two values are not equal, considering both value and type. If the values and data types are not equal, the condition returns true; otherwise, it returns false.
Example:
console.log(5 !== '5'); // true
console.log(0 !== false); // true
console.log(5 !== 5); // false
Explanation:
- 5 !== '5': The data types are different, which returns true.
- 0 !== false: The types differ (number vs. boolean), so this returns true.
- 5 !== 5: Both value and type are the same, so this returns false.
When to Use !==
!== is generally the recommended operator for checking inequality because it avoids implicit type conversion, leading to fewer unexpected outcomes.
Conclusion
Choosing between ==, !=, ===, and !== depends on how strict you want your comparisons to be. For most modern JavaScript projects, === and !== are the go-to choices for avoiding bugs caused by unexpected type coercion.
Mastering these comparison operators is a simple but important step towards writing code that’s clean, consistent, and easier to debug. Whether you're working on form validation, API logic, or frontend conditions, getting this right can save time and frustration.
If you're tackling a bigger build or want to improve your frontend codebase, StaticMania offers expert support in JavaScript development, performance tuning, and full-scale web projects. From code-level audits to complete web solutions, we help teams ship faster, cleaner, and more scalable applications.
Let us know how we can help — we’re just a message away.