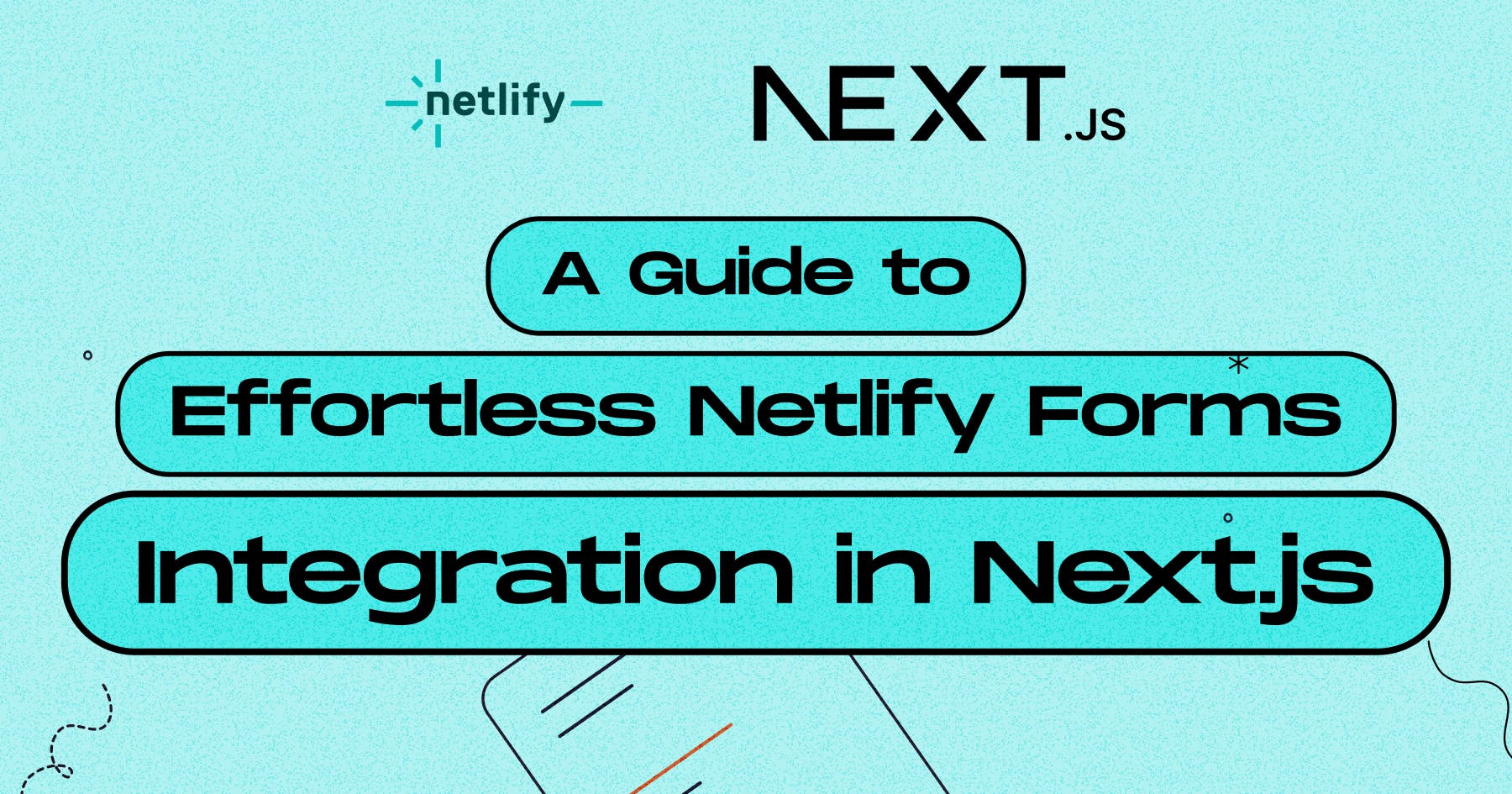
A Guide to Effortless Netlify Forms Integration in Next.js
- Author: Md. Saad
- Published at: April 17, 2024
- Updated at: April 17, 2024
What is Netlify Form?
Netlify Forms is a feature of the Netlify platform that simplifies form handling for static websites. Developers can easily set up HTML forms on their websites with Netlify Forms, and Netlify will take care of form submissions for them. Netlify Forms offers spam protection and data storage by automatically gathering form submissions. It does away with the requirement for server-side programming and backend infrastructure, which simplifies the form setup method. From the Netlify dashboard, one may easily access form submissions and integrate them with other services as required.
What are we going to do?
We will create a very simple contact form in Next.js. Then, we will integrate the Netlify form in it so that people can send messages using it. To use Netlify Forms in a Next.js application, you'll need to follow these general steps:
Prerequisites
- You’ll need a Netlify account, which you can sign up for free Netlify.
- Ensure you have an existing web project built with Next.js. If not, create one using:
npx create-next-app <app-name>
Create A Contact form
First, create a contact form component. Go to your /components folder or the folder where you want to keep the components. Create a ContactForm.jsx file . Now create a form like the following form.
import React from 'react';
const ContactForm = () => {
return (
<form name="contact" action="" method="POST" >
<div>
<label htmlFor="name">Name:</label>
<input type="text" id="name" name="name" />
</div>
<div>
<label htmlFor="email">Email:</label>
<input type="email" id="email" name="email" />
</div>
<div>
<label htmlFor="message">Message:</label>
<textarea id="message" name="message"></textarea>
</div>
<button type="submit">Submit</button>
</form>
);
};
export default ContactForm;
It is a pretty simple form. Now, add Netlify functionality in the form. To do that follow the following steps.
- Add data-netlify="true" in the <form> tag of your code.
- Also, add a hidden input field whose value must be the same as the <form>name.
<input type="hidden" name="form-name" value="contact" />
We have successfully created a Netlify form component. Now, it’s time to use it on the page.
- Create a /app/contact/page.jsx file. This will be the router of our contact page.
- Now we have to import ContactForm.jsx component on this contact page. Our code should look like this:
Import ContactForm from ‘/components/ContactForm`;
export default function Contact() {
return (
<div>
<ContactForm />
</div>
)
}
Collecting Form Submission Messages
You can view the Verified Submissions in your form named "contact" (named after the hidden field we added to our ContactForm component) by clicking on "Forms" from the Netlify dashboard of your website.
Fixed Form Submission Route
So far our form works well. However, there is a problem with it. As it will, when you submit a form powered by Netlify, produce a 404 page. You can either create a dedicated page for successful form submission, set up a function that prevents users from being redirected, or send users back to the homepage (with the ability to add a query parameter to the URL to trigger a "success" message). Let's pursue this last option!
- Create a new file naming /app/success/page.jsx. Now add a Successful message in it.
Your code looks like this.
export default function Success() {
return (
<div>
You have Successfully Submitted the form
</div>
)
}
- Finally, back in ContactForm.jsx, update the action attribute to your <form> that directs users to /success page.
<form name="contact" action="/success" method="POST" >
We have successfully added the Netlify form to the contact page. Furthermore, we have also added a success page to show a success message. But, There is something more we can do with our form which is adding spam-preventing methods. Let’s discuss it now.
Spam Prevention
Netlify form has provided an awesome spam prevention service. All form submissions are filtered for spam using Akismet, an industry leader in spam detection. However, it also supports some extra spam prevention services such as honeypot-field, and reCAPTCHA 2. Let’s go through how to activate these services.
Honeypot field
"Honeypot" fields are designed to trap bots attempting to fill out the form. These fields are hidden from human users but are detectable by bots. If a form submission contains data in the honeypot field, it can be safely assumed to be from a bot and rejected. To implement this in Netlify, add a netlify-honeypot attribute to the form element. The value of the netlify-honeypot must be the same as the name of the <form> name. We have to ensure also that this input field is hidden.
reCAPTCHA 2
To enable Netlify to provide CAPTCHA:
- Add a data-netlify-recaptcha="true" attribute to your <form> tag.
- Insert an empty <div> element within your form where you want the CAPTCHA to display, also with the data-netlify-recaptcha="true" attribute.
Upon publishing your site, Netlify will automatically include the required HTML to render the CAPTCHA within your form
For more information on Spam Prevention. Follow the link.
That’s it. You have successfully added a Netlify Form on your site. Your final code in ContactForm.jsx should look like this:
import React from 'react';
const ContactForm = () => {
return (
<form name="contact" action="/success" method="POST"
data-netlify="true"
// For Honeypot
netlify-honeypot="form-name"
//For ReCaptcha2
data-netlify-recaptcha="true"
>
<input type="hidden" name="form-name" value="contact" />
<div>
<label htmlFor="name">Name:</label>
<input type="text" id="name" name="name" />
</div>
<div>
<label htmlFor="email">Email:</label>
<input type="email" id="email" name="email" />
</div>
<div>
<label htmlFor="message">Message:</label>
<textarea id="message" name="message"></textarea>
</div>
//For recaptcha 2
<div data-netlify-recaptcha="true"></div>
<button type="submit">Submit</button>
</form>
);
};
export default ContactForm;
To create a fully functional Netlify form and deploy your next.js app on Netlify, contact Staticmania. Our dedicated team will guide you on every step.