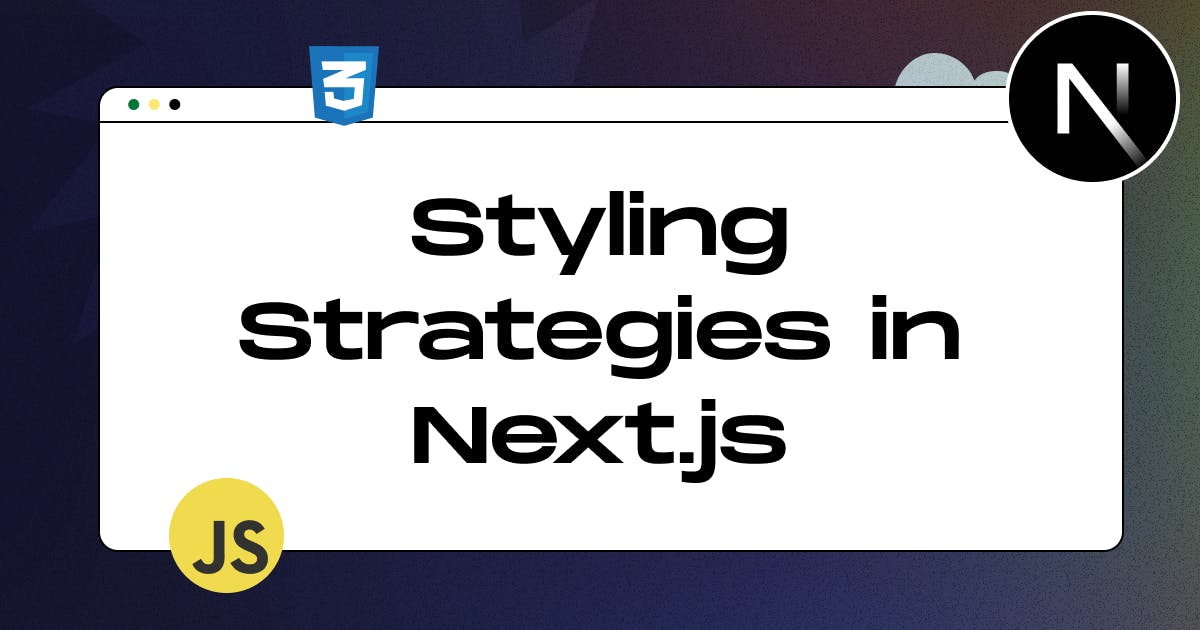
Styling Strategies in Next.js: Discovering CSS-in-JS, CSS Modules, and Styled Components
- Author: Md. Saad
- Published at: March 19, 2024
- Updated at: November 30, 2024
Next.js simplifies styling in React applications with flexibility in approaches. It accommodates CSS Modules, enables local scoping, and supports CSS-in-JS libraries like styled-components for inline styling. Additionally, traditional global styles can be easily managed. This versatility empowers developers to choose a styling method that aligns with the project requirements and coding preferences seamlessly and efficiently.
Styling in Next.js can be done using various approaches, and the framework is flexible enough to accommodate different preferences. Some of the popular methods include:
- Global Styles.
- CSS Modules
- CSS-in-JS library like ‘styled-components’ or ‘emotion
- Tailwind CSS
- Sass
Let's Go through and find out how all the possible methods can be added.
Global Styles
By default, Next.js generates a global.css file. It will be in the app folder and linked within the app/layout.jsx file. Change the app/global.css file as below and it will be added for all pages in next.js.
// app/global.css
.container{
padding: 50px 50px 60px;
max-width: 750px;
margin: 0 auto;
}
Inside the root layout (app/layout.js), import the global.css stylesheet to apply the styles to every route in your application:
import "./globals.css";
export default function RootLayout({children}) {
return (
<html lang="en">
<body className=”container”>{children}</body>
</html>
);
}
CSS Modules
- Create a CSS file with the" .module.css" extension. Styles defined in this file will be locally scoped to the component.
- Import and use the styles in your React components.
- An example is given below.:
// styles.module.css
.myComponent {
color: #333;
padding: 10px;
background-color: #eee;
}
A style module has been created and in the following example in a component named Component.js that style file has been imported.
// Component.jsx
import styles from './styles.module.css';
const MyComponent = () => {
return <div className={styles.myComponent}>This is a CSS Module component</div>;
};
export default MyComponent;
CSS-in-JS (Styled Components)
- Use a CSS-in-JS library like ‘styled-components’ or ‘emotion’.
- Write styles directly in your JavaScript/TypeScript files using template literals.
- Example with styled-components:
- In next.config.js, first activate styled-components.
module.exports = {
compiler: {
styledComponents: true,
},
}
Then, add a global registry component and a function to retrieve all CSS style rules generated during a render using the styled-components API. Then use the useServerInsertedHTML hook to inject the styles collected in the registry into the <head> HTML tag in the root layout. Create lib/registry.js and add the following codes:
'use client'
import React, { useState } from 'react'
import { useServerInsertedHTML } from 'next/navigation'
import { ServerStyleSheet, StyleSheetManager } from 'styled-components'
export default function StyledComponentsRegistry({ children }) {
// Only create stylesheet once with lazy initial state
// x-ref: https://reactjs.org/docs/hooks-reference.html#lazy-initial-state
const [styledComponentsStyleSheet] = useState(() => new ServerStyleSheet())
useServerInsertedHTML(() => {
const styles = styledComponentsStyleSheet.getStyleElement()
styledComponentsStyleSheet.instance.clearTag()
return <>{styles}</>
})
if (typeof window !== 'undefined') return <>{children}</>
return (
<StyleSheetManager sheet={styledComponentsStyleSheet.instance}>
{children}
</StyleSheetManager>
)
}
Finally, Wrap the children of the root layout(app/layout.jsx) with the style registry component:
import StyledComponentsRegistry from './lib/registry'
export default function RootLayout({ children }) {
return (
<html>
<body>
<StyledComponentsRegistry>{children}</StyledComponentsRegistry>
</body>
</html>
)
}
Tailwind CSS
Tailwind CSS is a utility-first CSS framework that works exceptionally well with Next.js.
- Install the Tailwind CSS packages and run the init command to generate both the tailwind.config.js and postcss.config.js files:
npm install -D tailwindcss postcss autoprefixer
npx tailwindcss init -p
- Now. Add paths to the files that will utilise Tailwind CSS class names inside tailwind.config.js:
/* @type {import('tailwindcss').Config} */
module.exports = {
content: [
'./app/**/*.{js,ts,jsx,tsx,mdx}', // Note the addition of the `app` directory.
'./pages/**/*.{js,ts,jsx,tsx,mdx}',
'./components/**/*.{js,ts,jsx,tsx,mdx}',
// Or if using `src` directory:
'./src/**/*.{js,ts,jsx,tsx,mdx}',
],
theme: {
extend: {},
},
plugins: [],
}
- Now, it is time to add tailwind directives to add tailwind global CSS in the applications: for example in the default app/global.css files add the following codes.
@tailwind base;
@tailwind components;
@tailwind utilities;
- At last like the global stylesheet add the global.css file in the app/layout.jsx file.
SASS files
Integrating SASS (Syntactically Awesome Stylesheets) files in Next.js is a common practice for enhanced styling capabilities. To use SASS in Next.js:
- First, install dependencies, using the following codes.
npm install --save-dev sass
- Ensure your next.config.js file is configured to handle SASS files.
const path = require('path')
module.exports = {
sassOptions: {
includePaths: [path.join(__dirname, 'styles')],
},
}
- Import the SASS files directly into your components.
// Component.jsx
import '../styles/your-styles.scss';
const MyComponent = () => {
return <div className="my-styled-component">Styled with SASS</div>;
};
export default MyComponent;
That's all relating to Styling in Next.js. We have discussed all the necessary styling features in Next.js. As a developer, It's up to you which method you will choose.
For any help, you can also contact us. Our expert team members will help you in all the possible ways.
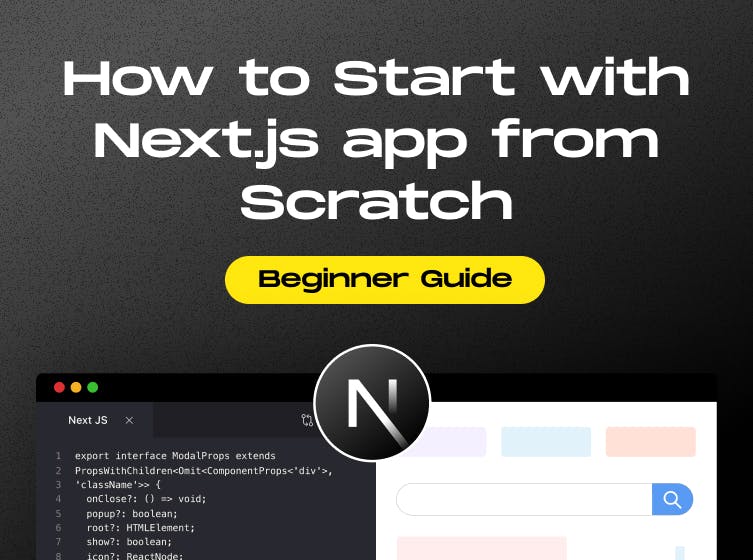
How to Start with Next.js app from Scratch (Beginner Guide)
Next.js provides a zero-config setup for speedy project start-up while allowing customisation via configuration files. Its tight connection with React and effective handling of server-side rendering help to construct scalable and SEO-friendly applications.
Read article