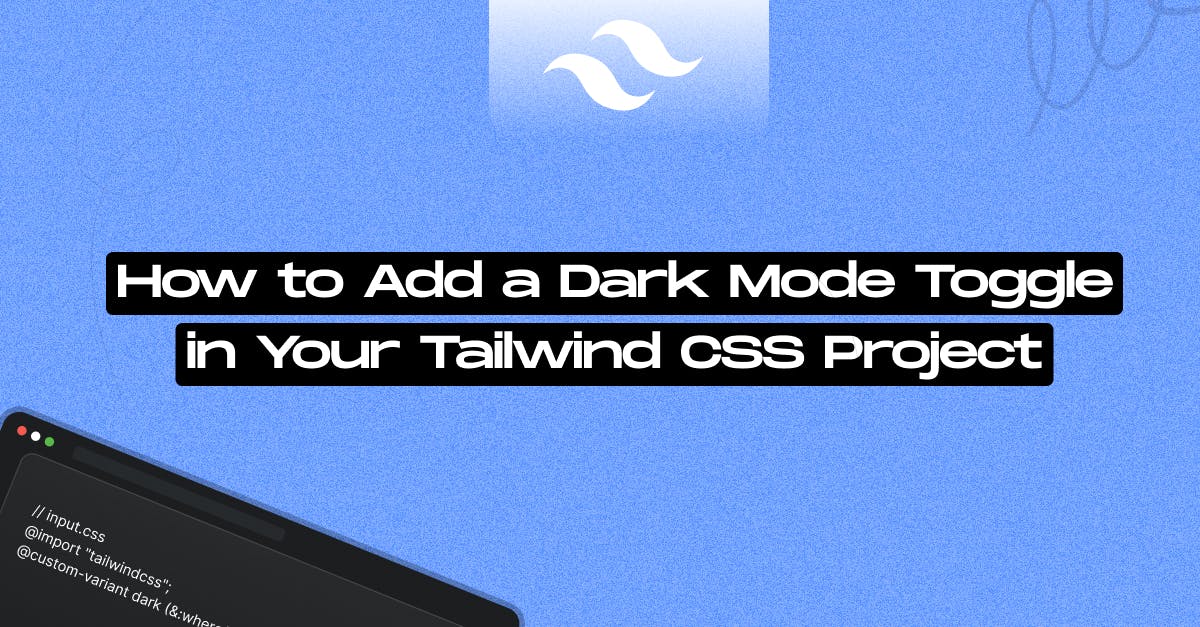
How to Add a Dark Mode Toggle in Your Tailwind CSS Project
- Author: Md. Saad
- Published at: April 12, 2025
- Updated at: April 13, 2025
Dark mode is now an essential feature in modern web development. As a result, it is becoming increasingly common to design a dark version of your website in addition to the default design. Tailwind has built-in support for dark mode through the dark: variant. This uses the prefers-color-scheme CSS media feature by default, but you can override the dark variant to build sites that allow you to toggle dark mode manually. This guide will look at how to use Tailwind CSS to implement a dark mode toggle.
Initial Project Setup
Before diving into dark mode implementation, we have to set up a basic Tailwind CSS project. Read the article to create a basic Tailwind project.
Setting Up Tailwind for Dark Mode in Tailwind V4
If you want your dark theme to be driven by a CSS selector instead of the prefers-color-scheme media query, override the dark variant to use your custom selector. Add the following codes in your input.css file.
// input.css
@import "tailwindcss";
@custom-variant dark (&:where(.dark, .dark *));
That’s all. You are ready to use dark mode on your site. But we also want to toggle it with light mode. Let’s create a button and some JavaScript code to do that.
Creating a Dark Mode Toggle Button And Javascript Code
Next, let's add a button to toggle dark mode. First, structure your HTML:
<button
id="themeToggle"
class="mb-5 rounded-lg bg-amber-800 px-2.5 py-1.5 text-black dark:bg-lime-100 dark:text-black"
>
Toggle Theme
</button>
Now, use JavaScript to handle the toggle functionality:
function applyTheme() {
let savedTheme = localStorage.getItem("theme");
const prefersDark = window.matchMedia("(prefers-color-scheme: dark)");
function setTheme(event) {
// If there's no saved theme, use system preference
if (!savedTheme) {
savedTheme = event
? event.matches
? "dark"
: "light"
: prefersDark.matches
? "dark"
: "light";
}
const isDark = savedTheme === "dark";
document.documentElement.classList.toggle("dark", isDark);
document.documentElement.setAttribute(
"data-theme",
isDark ? "dark" : "light",
);
}
setTheme();
prefersDark.addEventListener("change", setTheme);
}
// Function to toggle theme and save preference
function toggleTheme() {
const isDarkMode = document.documentElement.classList.contains("dark");
if (isDarkMode) {
document.documentElement.classList.remove("dark");
document.documentElement.setAttribute("data-theme", "light");
localStorage.setItem("theme", "light");
} else {
document.documentElement.classList.add("dark");
document.documentElement.setAttribute("data-theme", "dark");
localStorage.setItem("theme", "dark");
}
}
// Apply theme on page load
applyTheme();
document
.getElementById("themeToggle")
.addEventListener("click", toggleTheme);
This script ensures that dark mode persists across page reloads by storing the preference in localStorage. Again, you can manage this however you like, even storing the preference server-side in a database and rendering the class on the server — it's totally up to you.
Styling Elements for Dark Mode
Now, let's apply dark mode styles to different elements using Tailwind's dark: variant. Add dark: variant before any class name to add it in the dark mode.
<body class="bg-gray-100 p-8 dark:bg-gray-800">
<button
id="themeToggle"
class="mb-5 rounded-lg bg-amber-800 px-2.5 py-1.5 text-black dark:bg-lime-100 dark:text-black"
>
Toggle Theme
</button>
<h1 class="text-shadow font-display text-gray-600 dark:text-gray-100">
Hello, Tailwind with Custom Styles!
</h1>
</body>
With these classes, the background and text colors will automatically switch when dark mode is enabled.
Setting Up Tailwind for Dark Mode in Tailwind V3
You can enable dark mode in Tailwind V3 in your tailwind.config.js file by setting the mode to class:
module.exports = {
darkMode: 'class', // Enables class-based dark mode
theme: {
extend: {},
},
plugins: [],
};
This approach allows you to toggle dark mode using JavaScript by adding or removing a dark class on the HTML tag.
Conclusion
To implement dark mode using Tailwind CSS, use the dark: variation and class-based toggling. By integrating Tailwind with JavaScript, you can offer customers a seamless theme-switching experience that lasts across sessions.
Now that you have a functional dark mode implementation, feel free to add transitions, animations, or even system-preferred dark mode detection!
Need help with Tailwind problems in your project? Get in Touch With Us Today!