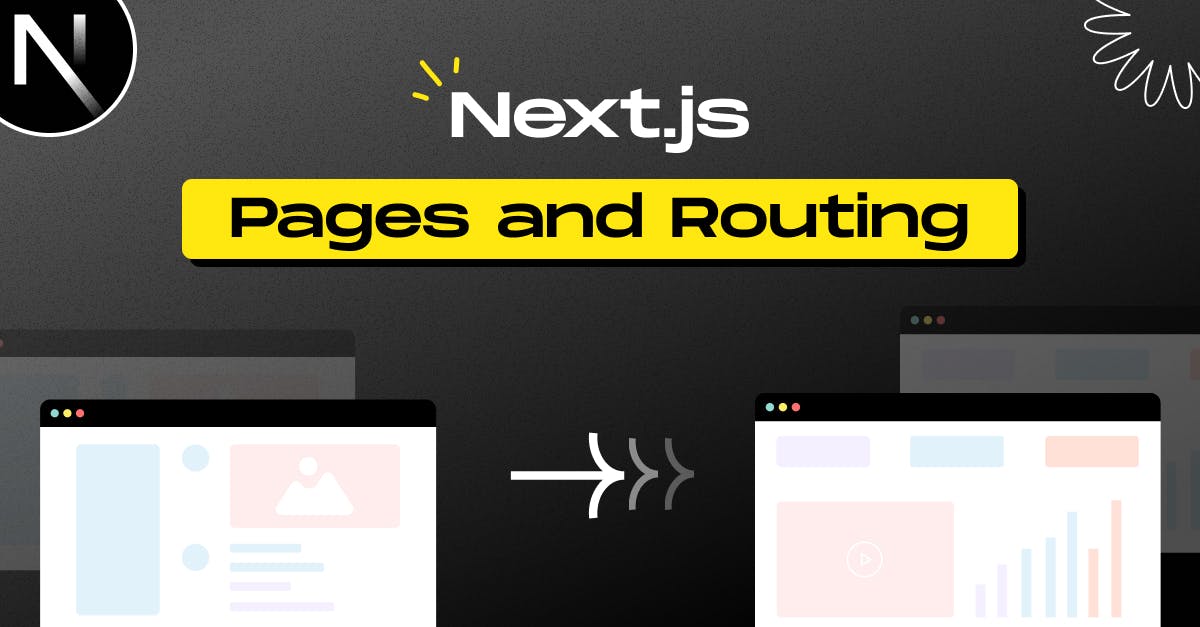
A Comprehensive Guide to Next.js Pages and Routing
- Md. Saad
- February 25, 2024
Have you ever experienced the thrill of smoothly moving among many pages in a web application, each with its own set of material and functionality? That's where Next.js shines! In this blog post, we'll go on a trip to examine the principles of Next.js, with a special emphasis on its pages and routing system.
How to Create a Route with Pages Directory
Every Next.js application is built around the pages directory. It's more than simply a folder; it's the architectural blueprint that defines your web app's routes. Each .js or .jsx file in this directory is immediately converted into a page with a corresponding route.
Let us consider a basic example. If you create a file named about.js in the pages directory, Next.js will naturally map it to the /about route. It's that easy! This file-based routing streamlines the organization of your project, making it simple to comprehend and maintain.
Dynamic Routes
Next.JS does not limit itself to static routes; it embraces dynamic wholeheartedly. You can design dynamic routes that capture URL values by enclosing them in square brackets [ ]. For example, consider the file [id].js converts into a dynamic route that allows you to navigate to different pages based on the value of id in the URL.
// pages/post/[id].js
import { useRouter } from 'next/router';
const Post = () => {
const router = useRouter();
const { id } = router.query;
return <p>Post ID: {id}</p>;
};
export default Post;
How to Create a Route with App Directory
The App Router also allows you to create directories within the app directory. To define your route, place a page.js file within the relevant subdirectory. If you want to create /about route in the App directory, create an app/about folder and place a page.js file inside it. Next.js will naturally map it to the /about route.
Dynamic Routes in App Directory
Next.js also support dynamic routes in the App Directory. You can add a dynamic route by enclosing a folder name [id] and adding page.js file inside it. Suppose you want to add a blog page and blog/ids pages. To do that first, add a blog folder inside the app directory and add a page.js file. This will create a route /blog. Now inside the blog folder add another folder within the third bracket such as [id]. This will create routes /blog/1 or /blog/.
The Link Component
Next.js introduces the powerful Link component from the next/link module, allowing client-side navigation without requiring a full page reload. It is essential for smooth transitions between pages and improving the user experience.
// pages/index.js
import Link from 'next/link';
const HomePage = () => (
<div>
<h1>Home Page</h1>
<Link href="/about">
<a>About Page</a>
</Link>
</div>
);
export default HomePage;
In Conclusion
Next.js provides a great experience for developing online apps, and its pages and routing architecture lay the groundwork for this experience. The ease of file-based routing, dynamic routes, Link components, and programmatic navigation all contribute to a developer-friendly ecosystem.
As we get to the end of our journey, keep in mind that Next.js pages and routes are more than simply components and URLs; they provide the foundation for a seamless and interactive user experience. So, let's keep diving into the realm of Next.js and discover its possibilities for developing strong and dynamic web apps!