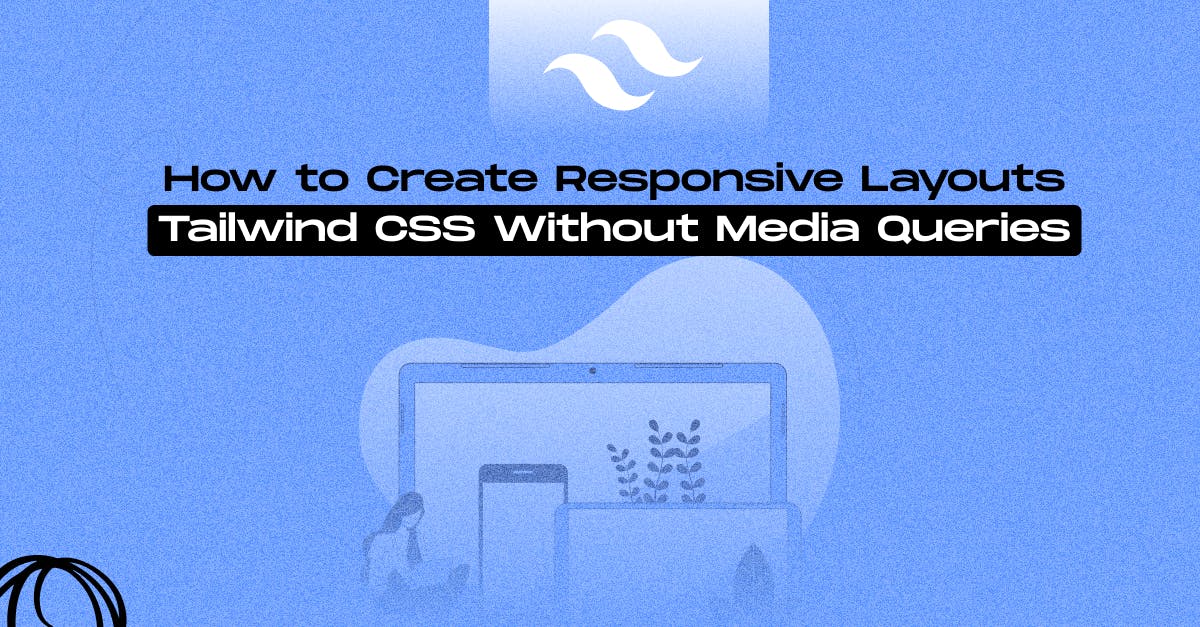
How to Create Responsive Layouts Using Tailwind CSS Without Media Queries
- Author: Md. Saad
- Published at: March 30, 2025
- Updated at: May 12, 2025
A key component of contemporary web development is responsive web design, which ensures that applications run smoothly and look excellent across all screen sizes. While media queries have historically been the preferred method for producing responsive designs, Tailwind CSS's user-friendly utility classes allow you to create responsive layouts without explicitly writing media queries.
Creating Responsive Layouts Using Tailwind CSS Without Media Queries Using Tailwind V4
Tailwind CSS allows you to apply utility classes conditionally at various breakpoints, enabling seamless responsive design without modifying your HTML structure.
Before using responsive utilities, ensure you have included the viewport meta tag in your <head>:
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
To apply a utility class only at a specific breakpoint, prepend the utility with the breakpoint name followed by “:”:
<!-- Default width: 16, medium screens: 32, large screens: 48 -->
<img class="w-16 md:w-32 lg:w-48" src="..." />
Default Breakpoints
Tailwind includes five default breakpoints based on common device resolutions:
Prefix | Min Width | CSS Rule |
---|---|---|
sm |
40rem (640px) |
@media (width >= 40rem) { ... } |
md |
48rem (768px) |
@media (width >= 48rem) { ... } |
lg |
64rem (1024px) |
@media (width >= 64rem) { ... } |
xl |
80rem (1280px) |
@media (width >= 80rem) { ... } |
2xl |
96rem (1536px) |
@media (width >= 96rem) { ... } |
These breakpoints apply to all utility classes, allowing full customization of properties like spacing, typography, and more.
Example: Responsive Component
The following example demonstrates a layout that stacks on small screens and switches to a side-by-side layout on larger screens:
<div class="mx-auto max-w-md overflow-hidden rounded-xl bg-white shadow-md md:max-w-2xl">
<div class="md:flex">
<div class="md:shrink-0">
<img class="h-48 w-full object-cover md:h-full md:w-48" src="/img/building.jpg" alt="Building" />
</div>
<div class="p-8">
<div class="text-sm font-semibold tracking-wide text-indigo-500 uppercase">Company retreats</div>
<a href="#" class="mt-1 block text-lg font-medium text-black hover:underline">
Incredible accommodation for your team
</a>
<p class="mt-2 text-gray-500">
Plan a retreat with great food and sunshine. Explore our curated destinations.
</p>
</div>
</div>
</div>
Mobile-First Approach
Tailwind follows a mobile-first strategy, meaning base styles apply to all screen sizes unless overridden with a prefixed utility:
<!-- Centers text on all screens -->
<div class="text-center"></div>
<!-- Centers text on mobile, aligns left on screens 640px+ -->
<div class="text-center sm:text-left"></div>
Targeting Breakpoint Ranges
By default, styles like md:flex apply from the md breakpoint and up. To limit styles to a specific range, use a max-* variant:
<div class="md:max-xl:flex">
<!-- Applies flex only between md and xl breakpoints -->
</div>
Available max-* variants:
Variant | Media Query |
---|---|
max-sm |
@media (width < 40rem) |
max-md |
@media (width < 48rem) |
max-lg |
@media (width < 64rem) |
max-xl |
@media (width < 80rem) |
max-2xl |
@media (width < 96rem) |
Customizing Tailwind CSS in V4
Customizing Breakpoints
Modify breakpoints in your theme using CSS variables:
@import "tailwindcss";
@theme {
--breakpoint-xs: 30rem;
--breakpoint-2xl: 100rem;
--breakpoint-3xl: 120rem;
}
Usage example:
<div class="grid xs:grid-cols-2 3xl:grid-cols-6">
<!-- Responsive grid using custom breakpoints -->
</div>
Removing Default Breakpoints
To reset a breakpoint to its default value:
@import "tailwindcss";
@theme {
--breakpoint-2xl: initial;
}
To remove all defaults and define custom breakpoints:
@import "tailwindcss";
@theme {
--breakpoint-*: initial;
--breakpoint-tablet: 40rem;
--breakpoint-laptop: 64rem;
--breakpoint-desktop: 80rem;
}
Using Arbitrary Values
For one-off breakpoints, use min-* and max-* variants with custom values:
<div class="max-[600px]:bg-sky-300 min-[320px]:text-center">
<!-- Custom breakpoint styles -->
</div>
Container Queries
Container queries allow styling based on the size of a parent element rather than the viewport. This makes components more reusable and adaptable.
Basic Example
Mark an element as a container using @container, then apply styles to child elements based on container size:
<div class="@container">
<div class="flex flex-col @md:flex-row">
<!-- Responsive layout within container -->
</div>
</div>
Max-Width Container Queries
Apply styles below a specific container size:
<div class="@container">
<div class="flex flex-row @max-md:flex-col">
<!-- Changes layout below md container size -->
</div>
</div>
Named Containers
For nested containers, use named queries:
<div class="@container/main">
<div class="flex flex-row @sm/main:flex-col">
<!-- Styles applied within named container -->
</div>
</div>
Customizing Container Sizes
Modify container sizes using theme variables:
@import "tailwindcss";
@theme {
--container-8xl: 96rem;
}
Example usage:
<div class="@container">
<div class="flex flex-col @8xl:flex-row">
<!-- Responsive container-based layout -->
</div>
</div>
Arbitrary Container Query Values
For unique container queries:
<div class="@container">
<div class="flex flex-col @min-[475px]:flex-row">
<!-- Custom query handling -->
</div>
</div>
Creating Responsive Layouts in Tailwind CSS using V3
Uses of responsive layouts using Tailwind CSS V3 are almost the same. The only difference lies in the setup option. First, create a Tailwind project in V3. You can get assistance from this link. The key differences are in customising the container size or creating a new breakpoint. Another difference is that v4 uses rem instead of px to measure the breakpoint.
Existing Default Breakpoint In Tailwind CSS V3
Prefix | Min Width | CSS Rule |
---|---|---|
sm |
640px |
@media (width >= 640px) { ... } |
md |
768px |
@media (width >= 768px) { ... } |
lg |
1024px |
@media (width >= 1024px) { ... } |
xl |
1280px |
@media (width >= 1280px) { ... } |
2xl |
1536px |
@media (width >= 1536px) { ... } |
You can also change the existing breakpoint from tailwind.config.js file like:
module.exports = {
theme: {
screens: {
'sm': '600px', // => @media (min-width: 640px) { ... }
'md': '768px', // => @media (min-width: 768px) { ... }
'lg': '1024px', // => @media (min-width: 1024px) { ... }
'xl': '1280px', // => @media (min-width: 1280px) { ... }
}
}
}
Creating New Breakpoints using Tailwind CSS v3
Tailwind CSS allows you to completely customize your breakpoints by modifying the tailwind.config.js file. This flexibility lets you define screen sizes that best fit your project’s responsive design needs.
You can specify custom breakpoints under the theme.screens section in your tailwind.config.js file:
/** @type {import('tailwindcss').Config} */
module.exports = {
theme: {
screens: {
'mobile': '480px', // Target small devices
// => @media (min-width: 480px) { ... }
'tablet': '768px', // Common breakpoint for tablets
// => @media (min-width: 768px) { ... }
'laptop': '1024px', // Suitable for most laptops
// => @media (min-width: 1024px) { ... }
'desktop': '1440px', // High-resolution screens
// => @media (min-width: 1440px) { ... }
},
},
}
Each key inside screens represents a named breakpoint. The values define the minimum width at which the styles will apply. For example:
@media (min-width: 1024px) {
/* Styles for laptops and larger screens */
}
Conclusion
By using Flexbox, Grid, Tailwind CSS's utility-first approach, and pre-installed responsive prefixes, you can create responsive layouts without manually writing media queries. As a result, development becomes quicker, cleaner, and easier to manage. Start using these techniques in your projects to improve the efficiency of your responsive design process!
Happy coding and customizing!
Need help with Tailwind problems in your project? Get in Touch With Us Today!