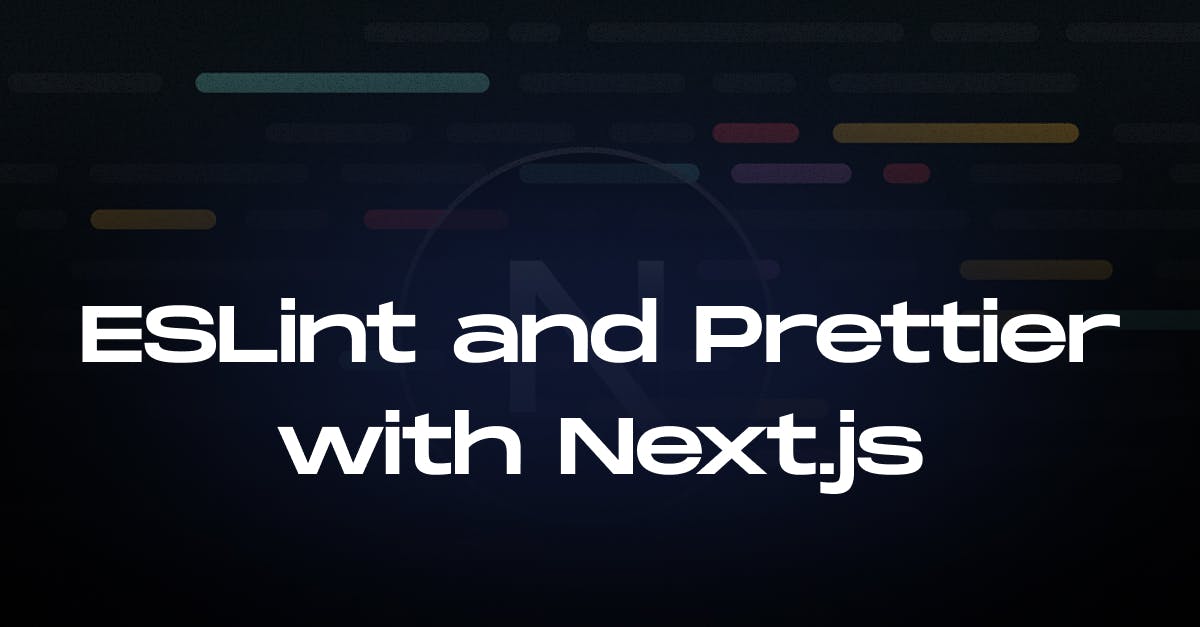
How to Integrate ESLint and Prettier with Next.js for Code Quality
- Author: Md. Saad
- Published at: September 22, 2024
- Updated at: September 22, 2024
What is ESLint?
The open-source JavaScript linting tool ESLint was created by Nicholas C. Zakas in June 2013. It often identifies problematic patterns or codes that deviate from style standards.
We can increase the code standard by setting up unique EsLint rules. These codes are pluggable. Moreover, it is written using Node.js. Thus, it can be installed easily.
What is Prettier?
Prettier is an opinionated code formatter. It enforces a consistent style by parsing and reprinting your code with its own rules that consider the maximum line length and wrapping code when necessary.
ESLint and Prettier in Next.js
Next.js has built-in ESLint support. So, integrating ESLint and Prettier with Next.js is an excellent way to maintain clean and consistent code quality. ESLint focuses on finding and fixing problematic patterns in JavaScript code, while Prettier handles automatic code formatting. Here's a step-by-step guide on how to set them up with a Next.js project:
Create Your Next.js Application
Before setting up the GitHub Actions workflow, the first and foremost step is to create a Next.js App. Use the following command to create a next.js app.
npx create-next-app@latest app-name
As it is not an article on creating the next.js app, we will not discuss it in detail. If you want to read it in detail, follow the How to Start with Next.js app from Scratch.
Install ESLint and Prettier
Install ESLint, Prettier, and some useful plugins to get them working together:
npm install --save-dev eslint prettier eslint-config-prettier eslint-plugin-prettier
- eslint: Lints your JavaScript/TypeScript code.
- prettier: Automatically formats your code.
- eslint-config-prettier: Disables ESLint rules that would conflict with Prettier.
- eslint-plugin-prettier: Integrates Prettier into ESLint so that Prettier errors show up as ESLint errors.
Configure ESLint
Next.js has built-in ESLint support, so you can initialize ESLint with:
npx next lint
This command creates a .eslintrc.json file in the root of your project, which includes some default rules.
Now, modify the .eslintrc.json to integrate Prettier. Update it as follows:
{
"extends": [eslint:recommended", "next", "next/core-web-vitals", "prettier"],
"plugins": ["prettier"],
"rules": {
"prettier/prettier": [
"error",
{
"endOfLine": "auto"
}
]
}
}
This setup does the following:
- next: Uses the default ESLint config provided by Next.js.
- next/core-web-vitals: Helps to lint for the Web Vitals metrics.
- prettier: Enables Prettier as an ESLint rule and displays formatting issues as ESLint errors.
Configure Prettier
Create a Prettier configuration file (.prettierrc) to specify your preferred formatting rules:
{
"semi": true,
"singleQuote": true,
"trailingComma": "es5",
"printWidth": 80,
"tabWidth": 2
}
You can adjust these settings to match your style guide.
Update package.json Scripts
Add scripts to your package.json file to make running ESLint and Prettier checks easier.
{
"scripts": {
"lint": "next lint",
"format": "prettier --write 'app/**/*.{js,jsx,ts,tsx,css,html}’"
}
}
Running ESLint and Prettier in VS Code (Optional)
If you're using Visual Studio Code, you can enable automatic linting and formatting on save by installing the ESLint and Prettier VS Code extensions. Then, modify your workspace settings:
- Open your workspace settings (.vscode/settings.json) and add the following:
{
"editor.codeActionsOnSave": {
"source.fixAll.eslint": true
},
"editor.formatOnSave": true,
"eslint.validate": ["javascript", "javascriptreact", "typescript", "typescriptreact"]
}
This configuration will run ESLint and Prettier every time you save a file.
How to Use Lint-staged
You can run the linter on staged git files by using next lint with lint-staged. You can specify the usage of the --file flag by creating a .lintstagedrc.js file at the root of your project. Then add the following code to the file
const path = require('path')
const buildEslintCommand = (filenames) =>
`next lint --fix --file ${filenames
.map((f) => path.relative(process.cwd(), f))
.join(' --file ')}`
module.exports = {
'*.{js,jsx,ts,tsx}': [buildEslintCommand],
}
Support for TypeScript
Create-next-app --typescript will apply Next.js ESLint rules to your configuration along with TypeScript-specific lint rules with next/typescript. You can also add them manually. In the .eslintrc.json file add the following codes to enable TypeScript support:
{
"extends": [eslint:recommended", "next", "next/core-web-vitals", "next/typescript", "prettier"],
"plugins": ["prettier"],
"rules": {
"prettier/prettier": [
"error",
{
"endOfLine": "auto"
}
]
}
}
Conclusion
Integrating ESLint and Prettier with a Next.js project significantly improves code quality by ensuring consistency and enforcing best practices. With ESLint identifying problematic patterns and Prettier handling automatic code formatting, you can streamline your development process, reduce errors, and maintain clean code. These setups enhance collaboration, readability, and maintainability across your codebase.
I hope you gathered some insightful information from this article. Feel free to get in touch with StaticMania if you have any queries or comments. Enjoy your coding!