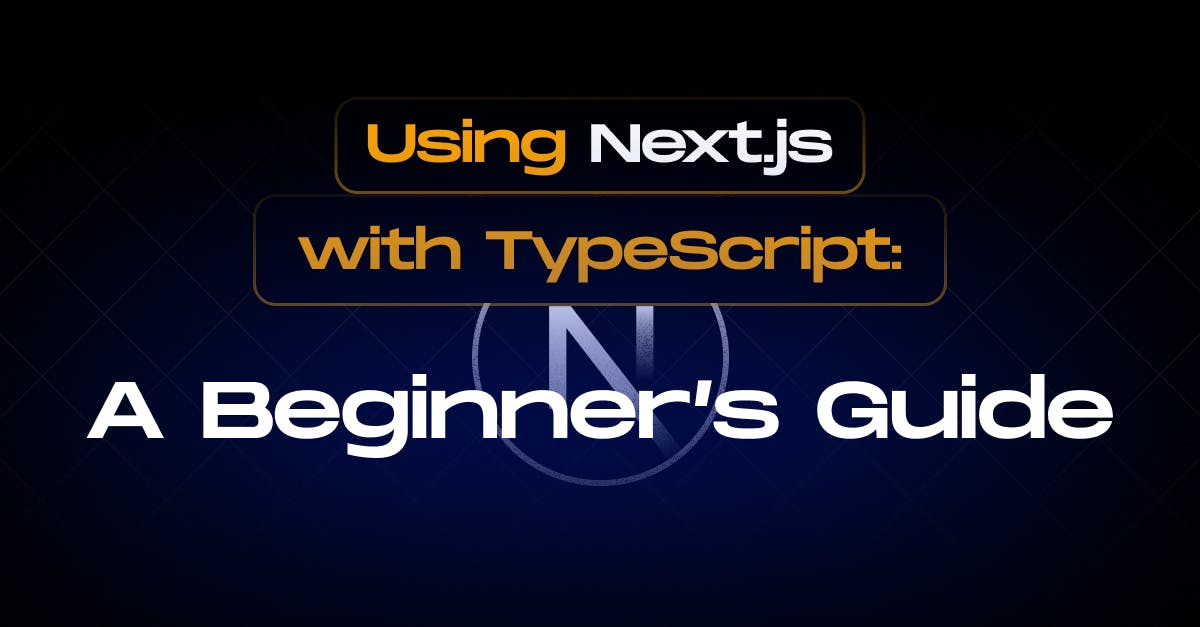
Using Next.js with TypeScript: A Beginner’s Guide
- Md. Saad
- June 23, 2024
What Is Next.Js?
Next.js is a popular React-based web framework that simplifies the development of dynamic and server-rendered web applications. It provides a powerful and flexible environment for building modern, responsive, and performant web pages.
Why Typescript?
TypeScript provides strong typing, which can significantly improve the developer experience by catching errors early and providing better documentation through types. With Next.js and TypeScript, you are well-equipped to build scalable and maintainable web applications.
More importantly, Next.js has excellent TypeScript support. As a result, we can easily integrate all the typescript functionality in a Next.js application.
In this article, we will discuss how to start with next.js using typescript. Furthermore, we will also learn how to convert an existing site into Typescript.
Pre Requisite on Next.js
Before diving into Next.js, I will assume that you have a basic understanding of the following technologies:
- Typescript
- React Js
- Node Modules
- HTML/CSS
Next.js Installation Guide
To get started with Next.js, you can follow these steps:
- Ensure that Node.js and npm are installed on your machine. You can download them from the official website: Node.js.
- Now, open your terminal and run the following command to create a new Next.js app:
npx create-next-appnext-app
- On installation, you'll be asked some questions about project configuration. Next.js by default uses Typescript and support for automatically installing the necessary packages and configuring the proper settings. So, we will set Typescript Yes in the installation process. You will see the following questions:
After answering all the questions, create-next-app will create a folder with your project name.
- Now navigate to the project using the following command.
cd my-next-app
- Install All the dependencies using the following codes:
npm install
- Now, start the development server by running:
npm run dev
- This command starts your Next.js app on http://localhost:3000 by default.
- Open your web browser and navigate to http://localhost:3000 to see your new Next.js app.
Congratulations, we have successfully created a next.js app with Typescript. Let’s make some new pages.
Create New Page
- The app/page.tsx file serves as a route or path definition in a webpage, taking its name from the folder within which it resides. If placed in the root (app) directory, it maps to the homepage (/) of the website.
- The app/layout.tsx file defines the layout for a particular route if placed in a folder. However, when placed in the root (app) directory, it becomes the layout for the entire web application. Consequently, all descendant routes inherit the layout specified in this file.
- Create a blog folder within the app directory to create specific routes, such as /blog. Inside this folder, include a page.tsx file that exports a function component. For example:
import React from "react";
const BlogPage = () => {
return (
<div>
<h1>Welcome to the Blog Page!</h1>
{/* Additional content for the blog page */}
</div>
);
};
export default BlogPage;
After running the server and navigating to /blog, you'll encounter a page displaying the heading "Welcome to Blog Page." In Next.js, all components and routes within the app directory are server components by default. Server components, such as forms, do not support client-side interactions. To enable client-side components, you have to include "use client"; at the beginning of the page.tsx file where you want to use it as a client component. For example:
"use client";
import React from "react";
const BlogPage = () => {
return (
<div>
<h1>Welcome to the Blog Page!</h1>
{/* Additional content for the blog page */}
</div>
);
};
export default BlogPage;
By adding the “use client “ directive, you specify that this page is intended for client-side rendering, allowing client-side interactions like forms to be incorporated. This distinction allows for flexibility in handling different components based on their requirements in a Next.js application.
Dynamic Routes
We have already seen how to create a static route but what should we do to create a dynamic route? Suppose we have to create a route for each blog post. Then we have to create a /blog/[slug] folder. Now, again create a page.tsx file inside the [slug] folder. Now paste the following code inside it.
"use client";
import React from "react";
const BlogSinglePage = ({params}) => {
const {slug} = params;
return (
<div>
<h1>Welcome to the Blog Page {slug}!</h1>
{/* Additional content for the blog page */}
</div>
);
};
export default BlogSinglePage;
To get the value of the slug from the path, we use the params prop. The params object is directly destructured in the function component, allowing you to access the slug directly and fetch data based on the dynamic parameter.
Converting an Existing Project
By adding a tsconfig.json file to the project's base directory, you can activate TypeScript on an already-existing Next.js project. So, first, create tsconfig.json file at the root. Now, Run npm run dev and npm run build to automatically install the necessary dependencies at the tsconfig.json file with the recommended config options. Finally, rename the existing project f files into .ts or .tsx. Finally, convert the javascript file to Typescript, if needed. If you already have a jsconfig.json file, copy the paths compiler option from the old jsconfig.json into the new tsconfig.json file, and remove the old jsconfig.json file.
This guide covers how to set up TypeScript in a Next.js project, whether you’re starting fresh or converting an existing project. By following these steps, you'll have a solid foundation to start building your Next.js application with TypeScript.
To attain an in-depth analysis of Next and its features, look at our How to Start with Next.js app from Scratch.
Do you have any questions or need assistance with getting started? Feel free to reach out to our Next.js experts.
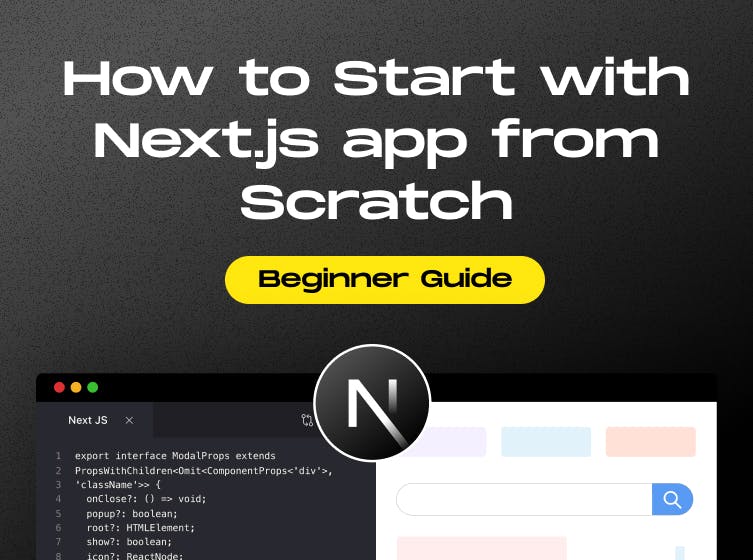
How to Start with Next.js App from Scratch
Next.js is a popular React-based web framework that simplifies the development of dynamic and server-rendered web applications. It provides a powerful and flexible environment for building modern, responsive, and performant web pages.
Read article