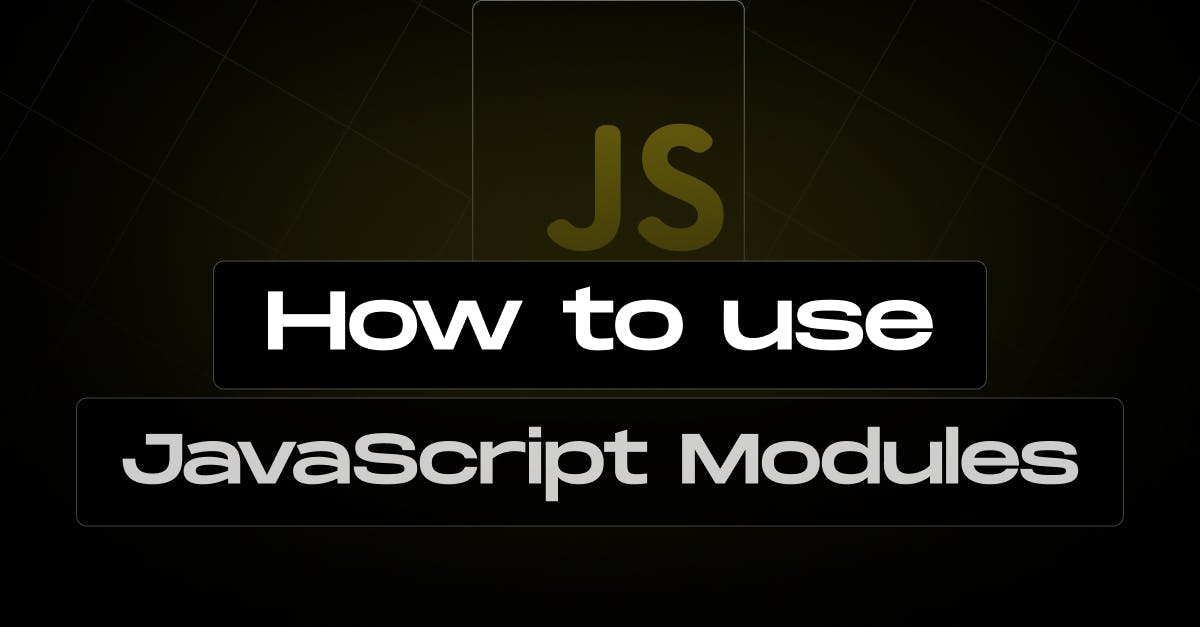
How to use JavaScript Modules
- Author: Md. Saad
- Published at: February 09, 2025
- Updated at: February 10, 2025
Introduction to JavaScript Modules
JavaScript has evolved significantly over the years, with ECMAScript 2015 (commonly referred to as ES6) introducing a wealth of new features that simplify and enhance development. One of the notable features is JavaScript Modules. This blog will explore what modules are, why they're important, and how to use them in your projects.
What Are JavaScript Modules?
Modules structure JavaScript code by splitting it into reusable and separate segments. Each module can export variables, functions, or classes and import functionality from other modules, making code more organized, reusable, and maintainable.
Why Use Modules?
1. Improved Code Organization
Modules allow developers to split large codebases into smaller, more manageable files. This not only makes it easier to locate and fix bugs but also simplifies the process of adding new features.
2. Enhanced Reusability
Encapsulating logic within modules allows you to reuse code across different parts of your application or even in entirely separate projects, reducing redundancy and improving efficiency.
3. Avoid Global Namespace Pollution
By isolating code inside their own scope, modules avoid conflicts and lessen the possibility of inadvertently overwriting global variables or functions. Cleaner, more dependable code results from this.
4. Lazy Loading
Modules allow you to load only the relevant portions of your code, increasing efficiency and cutting down on wasteful resource use.
Syntax of JavaScript Modules
Exporting from a Module
You can make functionality available to other modules using the export keyword. There are two types of exports:
- Named Exports – Allows multiple exports per module.
- Default Exports – Allows only one export per module.
Let’s explore each type in detail.
Named Exports
Named exports allow you to export multiple values from a module. This is useful when a module contains several functions, constants, or classes that need to be accessible elsewhere.
To use named exports, you define each export individually using the export keyword or list them all at once.
// mathUtils.js
export const add = (a, b) => a + b;
export const subtract = (a, b) => a - b;
export const multiply = (a, b) => a * b;
Here, add, subtract, and multiply functions are exported individually. Any other file can import them as needed.
Importing Named Exports
To use the functions from mathUtils.js, you import them using curly braces {}:
import { add, subtract } from './mathUtils.js';
console.log(add(5, 3)); // Output: 8
console.log(subtract(10, 4)); // Output: 6
If you need all exports from a module, you can import everything using the * syntax
import * as MathUtils from './mathUtils.js';
console.log(MathUtils.multiply(4, 2)); // Output: 8
This method imports everything as an object, where each exported function is a property.
Default Export
A default export allows a module to export a single value, function, or class. Unlike named exports, a default export does not require curly braces when imported, making it useful when a module is meant to provide a single primary functionality.
Example: Default Export
// logger.js
export default function log(message) {
console.log(message);
}
Here, the log function is the only exported value from logger.js.
Importing a Default Export
When importing a default export, you can name the imported value anything:
import log from './logger.js';
log('Hello, World!'); // Output: Hello, World!
Since it's a default export, there's no need for curly braces, and the function can be given any name during import:
import customLogger from './logger.js';
customLogger('Logging a message'); // Output: Logging a message
This flexibility makes default exports ideal for modules with a single task.
Combining Named and Default Imports
Both approaches can be combined in a single module if needed:
// utilities.js
export const greet = (name) => `Hello, ${name}!`;
export default function sayGoodbye(name) {
console.log(`Goodbye, ${name}!`);
}
Now, importing them:
import sayGoodbye, { greet } from './utilities.js';
console.log(greet('Alice')); // Output: Hello, Alice!
sayGoodbye('Alice'); // Output: Goodbye, Alice!
This structure allows flexibility while keeping the code modular and maintainable.
Practical Example
Here is a simple example of how you might use modules in a project:
File: greetings.js
export function sayHello(name) {
return `Hello, ${name}!`;
}
export function sayGoodbye(name) {
return `Goodbye, ${name}!`;
}
File: main.js
import { sayHello, sayGoodbye } from './greetings.js';
console.log(sayHello('Alice'));
console.log(sayGoodbye('Bob'));
Run the above code using a modern browser or a bundler like Webpack to see the modular system in action.
Browser Support
Modern browsers natively support ES6 modules. To use them in the browser, include the type="module" attribute in your <script> tag:
<script type="module" src="main.js"></script>
Note that modules loaded in this way follow stricter rules:
- Module scripts are deferred by default.
- Modules run in strict mode.
- Cross-origin module imports require the correct CORS headers.
Conclusion
JavaScript modules have revolutionized web development by offering a powerful, standardized way to structure and share code. With native support in modern browsers and development tools, there's never been a better time to incorporate them into your projects. Whether working on a small or large-scale app, using modules will help you write cleaner, more maintainable code.
Happy coding!
Need Help Implementing Javascript Modules in Your Project? Get in Touch With Us Today!
Frequently Asked Questions for JavaScript Modules
JavaScript modules allow you to break code into separate, reusable files. Each module can export and import functions, variables, or classes into other files. This helps improve code organization, reusability, and maintainability.
- Named exports allow multiple functions, variables, or classes to be exported from a module. When importing, curly braces {} are required.
- Default exports allow only one value to be exported per module. Curly braces are unnecessary when importing, and the imported value can be given any name.
JavaScript modules provide several benefits, including:
✅ Better Code Organization – Makes it easier to manage large codebases.
✅ Reusability – Allows code to be reused across different files and projects.
✅ Avoiding Global Namespace Pollution – Prevents variable conflicts.
✅ Lazy Loading – Loads only the required parts of the code, improving performance.
You can import everything from a module using the * syntax. This will create an object containing all the exported elements, allowing you to access them as properties.
Modern browsers support ES6 modules. To use modules in a browser, include type="module" in your <script> tag. However, older browsers may require a module bundler like Webpack or Babel for compatibility.
The best approach depends on project complexity, but common best practices include:
- Keeping related functions in the same module for better maintainability.
- Using default exports for standalone functionality and named exports for shared utilities.
Placing all modules in a dedicated modules or utils directory.