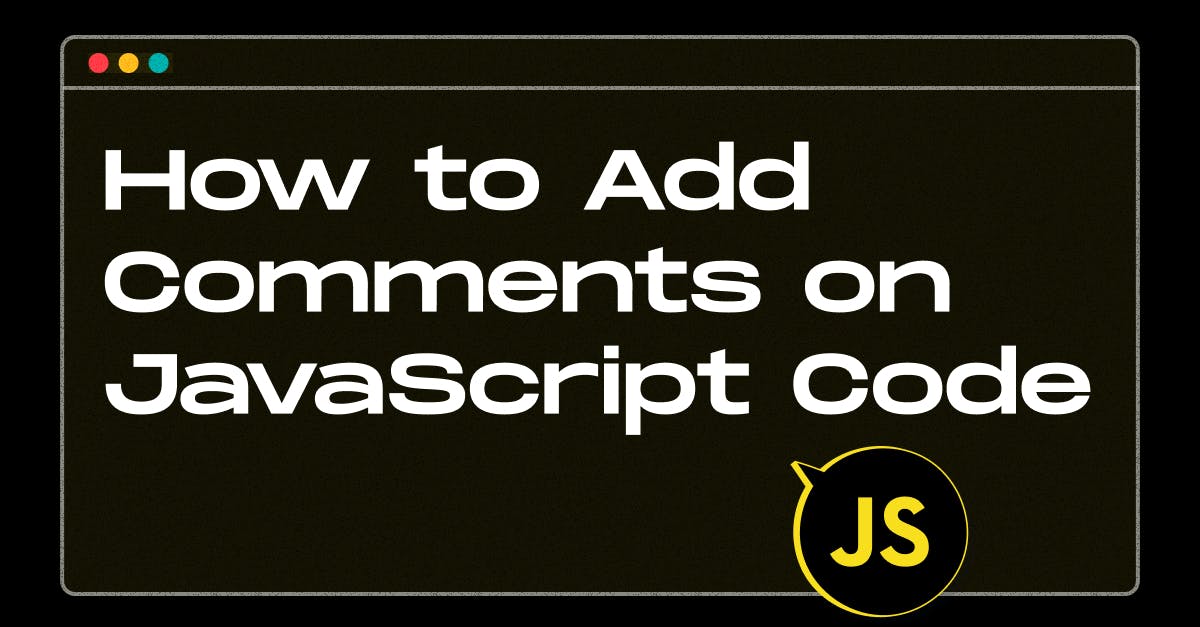
How to Add Comments on JavaScript Code?
- Author: Md. Saad
- Published at: December 29, 2024
- Updated at: January 06, 2025
Introduction
Comments are vital in improving code readability, maintainability, and collaboration within a development team. They add context, explain purpose, and make code easier to maintain. Well-commented code is easier for others (as well as one's future self) to understand. This blog will examine why comments are important, when to use them, and how to comment on JavaScript code effectively.
Why Are Comments Important?
- Enhance Readability: Comments help others (or you in the future) understand the purpose of your code, especially if it involves complex logic.
- Ease Debugging and Maintenance: When bugs need fixing or new features are added, comments can save time by explaining the code's original intent.
- Collaborative Development: In team settings, comments ensure everyone stays on the same page, making collaboration smoother.
Types of Comments in JavaScript
JavaScript supports two types of comments:
- Single-line comments: When writing short inline comments, use //.
// This is a single-line comment
let x = 10; // Initialize x with 10
- Multi-line comments: For more detailed explanations or to temporarily deactivate code blocks, use /* */.
/*
This is a multi-line comment.
It can span multiple lines.
*/
let y = 20;
Best Practices for Writing Comments
1. Use Descriptive Comments
Descriptive comments explain why the code exists and what it intends to do, rather than just restating it. They provide valuable context that can help developers quickly understand the logic behind specific sections.
Example:
Instead of this redundant comment:
let total = price * quantity; // Multiplying price by quantity
Write a comment that explains why:
// Calculate total cost based on selected items and their prices
let total = price * quantity;
2. Avoid Over-commenting
Too many comments can clutter up the code and make it difficult to maintain. Avoid adding comments to simple, self-explanatory code.
Bad Example (over-commented):
let count = 0; // Initialize count to 0
count++; // Increment count by 1
Good Example (minimal, meaningful comment):
// Keep track of user login attempts
let loginAttempts = 0;
3. Update Comments Regularly
Outdated comments can be more confusing than no comments at all. As your code evolves, it is critical to maintain comments up to date to ensure they are correct and relevant.
Example:
Suppose a function's behavior changes, but its comment is left unmodified:
// Returns the user's full name
function getUserName(user) {
return user.firstName; // Now only returns the first name, comment is outdated
}
Updated version:
// Returns the user's first name
function getUserName(user) {
return user.firstName;
}
4. Comment Complex Sections
Some of your code may contain complex logic, algorithms, or calculations that are not immediately apparent. Leaving comments in these areas can help others (or your future self) understand the logic behind them.
Example:
// Use dynamic programming to find the longest common subsequence (LCS)
// between two strings. This approach has a time complexity of O(m*n),
// where m and n are the lengths of the input strings.
function longestCommonSubsequence(str1, str2) {
const dp = Array(str1.length + 1).fill(null).map(() => Array(str2.length + 1).fill(0));
for (let i = 1; i <= str1.length; i++) {
for (let j = 1; j <= str2.length; j++) {
if (str1[i - 1] === str2[j - 1]) {
dp[i][j] = dp[i - 1][j - 1] + 1;
} else {
dp[i][j] = Math.max(dp[i - 1][j], dp[i][j - 1]);
}
}
}
return dp[str1.length][str2.length];
}
Conclusion
Writing effective comments is an important skill for any JavaScript developer. They improve teamwork and ensure your projects are future-proof by bridging the gap between code and human intelligence. By following the best practices outlined here, you'll be able to write comments that add value without overwhelming the codebase. Remember, the best code should be self-explanatory, but even the best code sometimes needs a little extra clarification!
We hope this blog helps you learn how to comment on JavaScript code. StaticMania, as a professional web development and top web design service agency, is happy to share coding knowledge. Keep writing effective comments! Reach out to StaticMania for any help or feedback. Our experts are ready to explore your dream. Happy coding!
FAQs about Javascript Comments
Comments are lines of code ignored by the JavaScript engine. They are used to explain code, improve readability, or temporarily disable code during debugging.
JavaScript supports two types of comments:
- Single-line comments, which start with //.
- Multi-line comments, enclosed between /* and */.
To write a single-line comment, start the line with //, followed by your comment text.
For multi-line comments, use /* to begin and */ to end the comment. Place your comment text between these symbols.
No, comments do not affect the performance since they are ignored during execution. However, excessive comments in production code can increase the file size if not minified.
No, there is no specific limit to the length of comments. However, it is best to keep them concise and relevant.
Comments improve code readability, help others understand your logic, and make future updates or debugging easier.
No, comments should be used only when necessary to explain complex logic, assumptions, or why a particular approach is used. Over-commenting can clutter the code.
You can comment out code temporarily to prevent its execution while testing other parts of the program.
Yes, comments can include any characters, but avoid using symbols that might confuse the reader or resemble code.
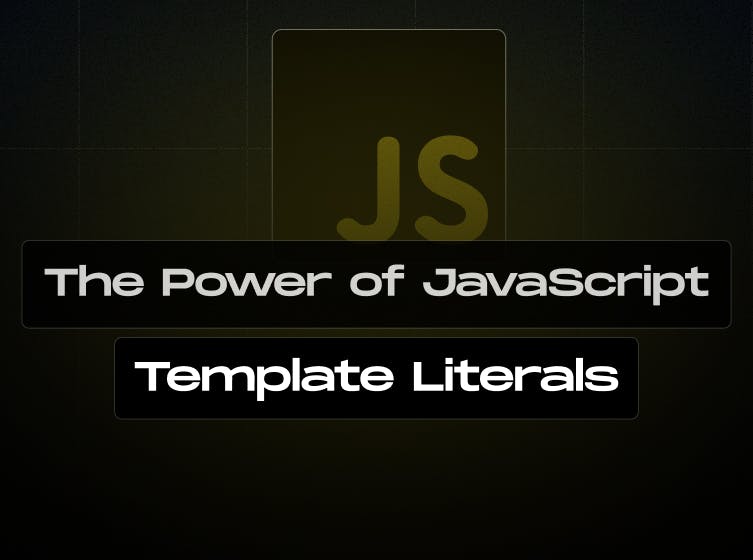
The Power of JavaScript Template Literals
A primary benefit of template literals is string interpolation. By doing this, you can include variables and expressions straight into your strings, eliminating the tedious concatenation operators (+).
Read article