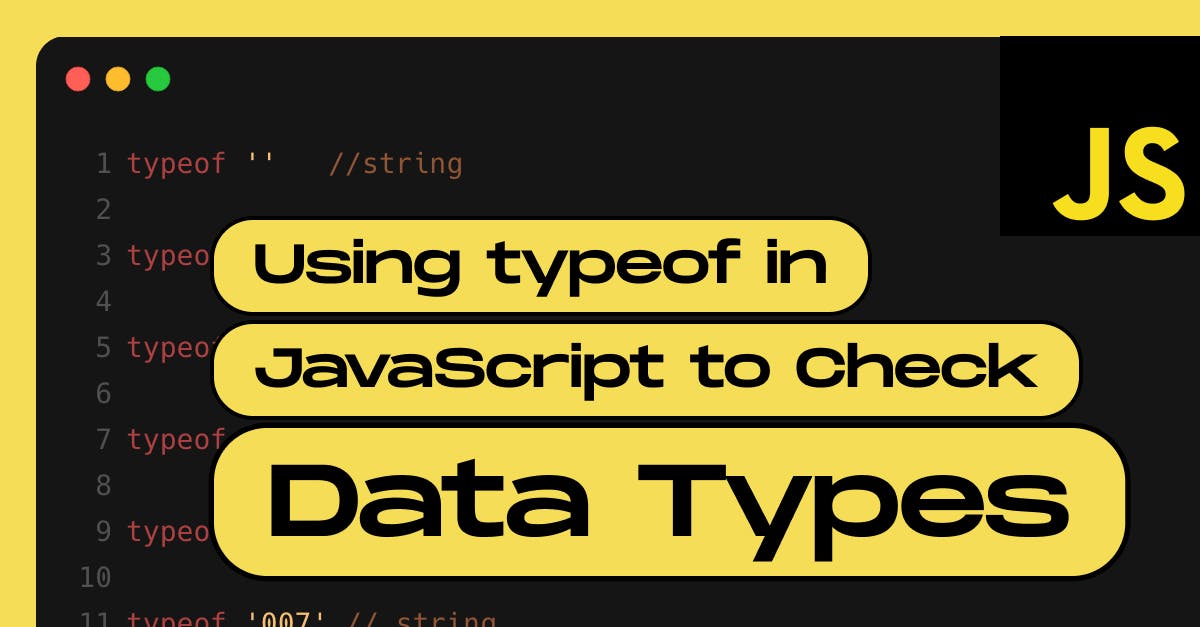
Using typeof in JavaScript to Check Data Types
- Author: Md. Saad
- Published at: December 22, 2024
- Updated at: December 26, 2024
What is the typeof operator?
The typeof operator in JavaScript is used to return a string that describes the type of its operand. The typeof operator is beneficial since it provides an easy method to check the variable type in your code.
The typeof operator returns a string that indicates the current type of a variable. The syntax is straightforward:
typeof operand
The operand can be any variable, object, function, or literal.
Common Use Cases
Here are the most common scenarios where typeof is used:
Checking Primitive Data Types
JavaScript has seven primitive data types: string, number, bigint, boolean, null, undefined, and symbol. Using typeof makes it easy to identify these:
typeof "John" // Returns string
typeof ("John"+"Doe") // Returns string
typeof 3.14 // Returns number
typeof 33 // Returns number
typeof (33 + 66) // Returns number
typeof true // Returns boolean
typeof false // Returns boolean
typeof 1234n // Returns bigint
typeof Symbol() // Returns symbol
In javascript, null is a primitive value. But when we check its typeof it returns object.
typeof null // Returns object
Complex Data Types in JavaScript
In JavaScript, complex data types store multiple values or a mix of data types in a single structure. These types are essential for creating sophisticated programs and managing structured data.
The Complex Data Type: object
JavaScript defines one primary complex data type: the object.
Objects can include multiple values organized as key-value pairs, enabling powerful ways to structure and manipulate data.
Variants of Objects
Several specialised structures in JavaScript are derived from the object type. These include:
- Arrays: ordered collections of elements.
- Functions: Blocks of reusable code.
- Sets: Collections of unique values.
- Maps: Key-value pairs with better performance and flexibility for dynamic keys.
Despite their differences, all these are fundamental objects in JavaScript.
The Role of the typeof Operator
The typeof operator is used to determine the type of a value. When applied to complex types:
- It returns "object" for objects, arrays, sets, maps, and more.
- It returns "function" specifically for functions.
console.log(typeof { key: "value" }); // "object"
console.log(typeof [1, 2, 3]); // "object" (arrays are objects)
console.log(typeof new Set()); // "object"
console.log(typeof function() {}); // "function"
Notice that both objects and arrays return"object," which is why additional checks (like Array.isArray()) are often necessary to distinguish between them.
const fruits = ["apples", "bananas", "oranges"];
Array.isArray(fruits);
Best Practices for Using typeof
The typeof operator is handy for checking if a variable is defined before attempting to access it in your code. This helps prevent errors that might occur when referencing an undefined variable.
function(a){
if (typeof(a) === 'undefined') {
console.log('variable a is not defined');
return;
}
// continue with function here...
}
Conclusion
The typeof operator is a valuable tool in every JavaScript developer's toolkit. Understanding its behavior and restrictions will allow you to successfully use it for type-checking and debugging. While there is no one-size-fits-all solution, combining typeof with additional type-checking techniques can help you write more reliable code.
Keep exploring and enhancing your knowledge of JavaScript! Please do not hesitate to contact StaticMania with any questions or feedback. Have fun coding!
FAQs About the typeof Operator in JavaScript
The typeof operator returns a string describing the type of its operand, such as string, number, boolean, object, undefined, function, or symbol.
The syntax is simple: typeof operand, where the operand can be a variable, object, function, or literal value.
This is a historical issue in JavaScript. Although null is a primitive value, the typeof operator incorrectly identifies it as an object.
No, typeof returns "object" for both arrays and objects. To distinguish them, additional methods like Array.isArray() are required.
The typeof operator specifically returns "function" for functions.
Its limitations include returning "object" for both arrays and objects, as well as incorrectly identifying null as "object."